Say goodbye to polling mode and embrace efficient Telegram Webhook! Unlike the polling method that continuously requests updates from the Telegram server, Webhook allows Telegram to push updates directly to your server, thereby reducing server resource consumption and significantly improving efficiency. In a previous article, I explained how to use Node.js to build a Telegram robot and use the polling method to obtain updates. While this approach works, if you're looking for scalability, even if you're not currently experiencing rate limits, switching to webhooks will ensure your bot runs more efficiently and can easily handle increasing traffic, reducing the load on your servers.
Key considerations for Telegram Webhook
When using webhooks, be sure to pay attention to the following points:
Allowed ports
Telegram only supports four webhook ports. While the reason for this restriction is currently unclear, it may change in the future. Currently supported ports are:
- 443 (HTTPS is recommended)
- 80
- 88
- 8443
Proper operation of the webhook requires one of these ports to be available and accessible. If you do not specify a port explicitly, the webhook will default to port 8443.
Server Limitations
Since only four ports are allowed, the number of applications using webhooks cannot exceed four on the same server.
Prerequisites
Before you start setting up, please make sure you have the following:
- Node.js installed: If it is not already installed, download and install Node.js from nodejs.org.
- Telegram Account: You need a Telegram account to get the bot token and interact with your bot.
- Ngrok for HTTPS URLs: Telegram requires webhooks to use HTTPS endpoints. Use Ngrok to expose your local server to the internet.
The complete code can be found on Github.
Telegram Webhook settings (Node.js example)
// 导入Telegram Bot API const TelegramBot = require('node-telegram-bot-api'); // 使用您的机器人令牌替换 const token = 'your telegram token'; // 请查看我的文章,了解如何在Telegram上从@BotFather获取机器人令牌 const WEB_HOOK_URL = 'https://localhost:3000/telegram-bot-webhook'; // 注意:它在localhost上无法工作,因此请使用ngrok之类的隧道服务。 // 创建使用Webhook的机器人 const bot = new TelegramBot(token, { webHook: { port: 88, // Telegram Webhook允许的端口:443、80、88、8443 }, }); // 初始化Webhook const initWebHook = async () => { const webhookInfo = await bot.getWebHookInfo(); if (webhookInfo.url !== WEB_HOOK_URL) { await bot.setWebHook(WEB_HOOK_URL, { max_connections: 100, }); } }; initWebHook(); // 监听任何消息 bot.on('message', (msg) => { const chatId = msg.chat.id; // 简单命令处理 if (msg.text.toLowerCase() === '/start') { bot.sendMessage(chatId, '欢迎!今天我能如何帮助您?', { reply_markup: { keyboard: [['/start', '/help']], }, }); } else if (msg.text.toLowerCase() === 'hello') { bot.sendMessage(chatId, `你好,${msg.from.first_name}!`); } else { bot.sendMessage(chatId, "我不确定如何回应。"); } }); // 导出机器人模块 module.exports = bot;
Telegram Webhook routing
const express = require("express"); const app = express(); const bot = require("./bot"); // 解析POST请求的JSON主体 app.use(express.json()); app.get("/", (req, res) => { res.send("Hello World"); }); app.post("/telegram-bot-webhook", (req, res) => { bot.processUpdate(req.body); res.sendStatus(200); }); app.listen(process.env.PORT || 3000, () => { console.log("服务器正在3000端口运行"); });
Summary
Switching to webhooks can eliminate Telegram rate limiting issues and make your bot more efficient. Just make sure you configure your server correctly, use allowed ports, and secure your connection using HTTPS.
Follow me to be notified when my next article is published ?.
The above is the detailed content of telegram webhook. For more information, please follow other related articles on the PHP Chinese website!
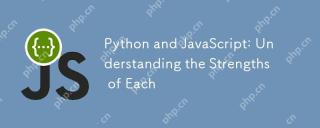
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
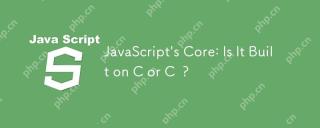
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
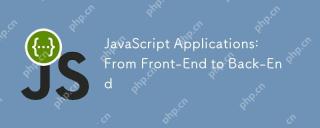
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
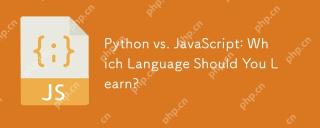
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
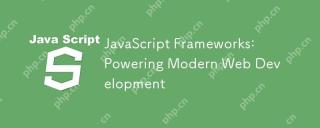
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
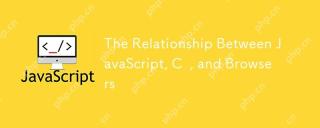
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
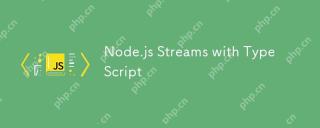
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
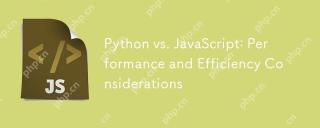
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
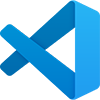
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
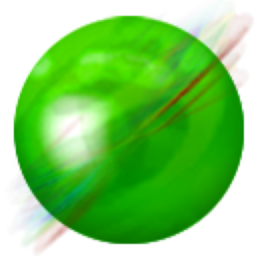
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
