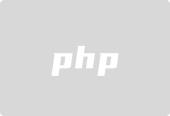
C# Singletons: A Deep Dive
A singleton in C# is a class designed to ensure only a single instance exists throughout the application's lifecycle, providing convenient access to that instance. This design pattern simplifies object management and control.
Advantages of Using Singletons
Employing singletons offers several key benefits:
-
Unified Data Source: Guarantees a single, consistent object instance across the application.
-
Controlled Access: Centralizes object creation and management, preventing multiple instances and inconsistent states.
-
Simplified Access: Offers a straightforward, global access method, eliminating the need for complex dependency injection or service location mechanisms.
When to Use Singletons
Consider using a singleton in these scenarios:
-
Global Configuration/State: When you require a globally accessible object for managing application-wide configuration or state.
-
Reduced Instantiation Overhead: To avoid the performance overhead of creating multiple object instances.
-
Coordination/Synchronization: To ensure only one instance exists for purposes of coordination or synchronization.
C# Singleton Implementation
Here's a common C# singleton implementation:
public sealed class Singleton
{
private static readonly Singleton _instance = new Singleton();
private Singleton() { }
public static Singleton Instance
{
get { return _instance; }
}
}
This implementation uses lazy initialization, ensuring only one instance is created and accessed.
Important Considerations
While beneficial, singletons should be used judiciously as they can introduce:
-
Increased Complexity: Singletons can lead to circular dependencies and make code harder to understand.
-
Testing Challenges: Mocking or stubbing singletons for testing can be difficult.
-
Thread Safety Concerns: In multi-threaded environments, thread safety must be carefully considered and implemented.
The above is the detailed content of What is a Singleton in C# and When Should You Use It?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn