Capturing Console Output in a C# Windows Forms TextBox
Many C# Windows Forms applications need to run external console applications and display their output within the application's user interface. A common solution is to redirect this console output to a TextBox. This guide outlines the process.
The key steps involve starting the external process with output redirection, handling the output events, and then monitoring the process.
-
Initiating the Process and Redirecting Output:
Begin by creating a
Process
object and configuring itsStartInfo
properties to redirect standard output and standard error streams:Process p = new Process(); p.StartInfo.FileName = @"C:\ConsoleApp.exe"; p.StartInfo.RedirectStandardOutput = true; p.StartInfo.RedirectStandardError = true; p.EnableRaisingEvents = true; p.StartInfo.CreateNoWindow = true; // Prevents a separate console window p.Start();
-
Processing Output Events:
Next, register event handlers for
OutputDataReceived
andErrorDataReceived
events. These events fire whenever the external process sends data to its standard output or standard error streams. A single event handler can be used for both:p.ErrorDataReceived += Proc_DataReceived; p.OutputDataReceived += Proc_DataReceived; p.BeginErrorReadLine(); p.BeginOutputReadLine();
The
Proc_DataReceived
event handler (which you'll need to define) will receive the output data and update the TextBox accordingly. For example:private void Proc_DataReceived(object sender, DataReceivedEventArgs e) { if (e.Data != null) { this.textBox1.Invoke((MethodInvoker)delegate { textBox1.AppendText(e.Data + Environment.NewLine); }); } }
-
Running and Waiting for the Process:
Finally, start the process and wait for it to complete:
p.Start(); p.WaitForExit();
By following these steps, you can seamlessly integrate the output of external console applications into your C# Windows Forms application, providing real-time feedback and enhancing the user experience. Remember to handle potential exceptions and consider adding error checking for robustness.
The above is the detailed content of How to Redirect Console Output to a TextBox in C#?. For more information, please follow other related articles on the PHP Chinese website!
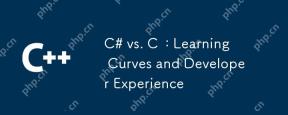
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
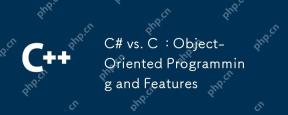
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
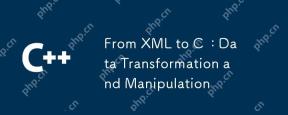
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
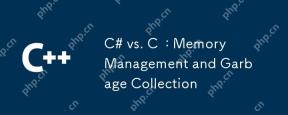
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.

C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
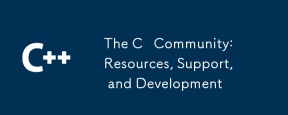
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
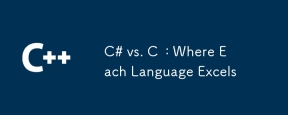
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
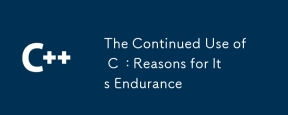
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
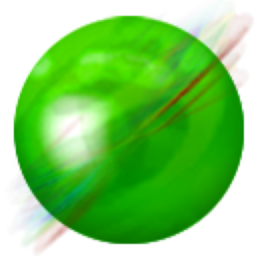
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
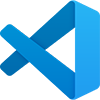
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.