


Identify and parse Html elements within frames/iframes in the WebBrowser control
Problem Overview
As stated in the query, while trying to collect video clip links from a specific website using the WebBrowser control, the web page contains iframes that host their own documents and elements. The video element cannot be found by relying solely on the main document's Html element. Therefore, it is necessary to delve into the elements of the iframe to find the required information.
Solving the problem: parsing the HtmlDocuments of the frame
To efficiently retrieve Html elements from a frame/iframe, you can take the following steps:
1. Identification framework:
- Use the WebBrowser.Document.Window.Frames property to access the HtmlWindowCollection containing all frames in the main document.
2. Parse the framework document:
- Iterate through each HtmlWindow in the collection.
- For each frame, access its HtmlDocument property to inspect its Html element.
3. Extract Html element attributes:
- Use the HtmlElement.GetAttribute method to extract relevant attributes from the recognized Html element.
Sample code snippet:
Here is a sample implementation demonstrating how to parse Html elements from a frame:
public class FrameHtmlElementParser { private List<MovieLink> movieLinks = new List<MovieLink>(); //更正变量名 public void ParseMovies(WebBrowser browser) { browser.DocumentCompleted += Browser_DocumentCompleted; } private void Browser_DocumentCompleted(object sender, WebBrowserDocumentCompletedEventArgs e) { var browser = sender as WebBrowser; if (browser.ReadyState != WebBrowserReadyState.Complete) { return; } var documentFrames = browser.Document.Window.Frames; foreach (HtmlWindow frame in documentFrames) { try { var videoElement = frame.Document.Body.GetElementsByTagName("video").OfType<HtmlElement>().FirstOrDefault(); //更正标签名 if (videoElement != null) { string videoLink = videoElement.GetAttribute("src"); int hash = videoLink.GetHashCode(); if (movieLinks.Any(m => m.Hash == hash)) //更正变量名 { // 完成此 URL 的解析。删除处理程序或采取其他适当的操作。 return; } string sourceImage = videoElement.GetAttribute("poster"); movieLinks.Add(new MovieLink //更正变量名 { Hash = hash, VideoLink = videoLink, ImageLink = sourceImage }); } } catch (UnauthorizedAccessException) { } // 无法避免:忽略 catch (InvalidOperationException) { } // 无法避免:忽略 } } } public class MovieLink //添加MovieLink类定义 { public int Hash { get; set; } public string VideoLink { get; set; } public string ImageLink { get; set; } }
Avoid duplicate data:
To prevent duplicate Html element attributes from being stored, the sample code uses a custom MovieLink class that contains the HashCode of each referenced link. By comparing the HashCode, it checks for duplicates before adding the new item to the movieLinks list.
The following modifications were made to the code:
-
Corrected spelling and capitalization errors in the code: For example,
movielink
is corrected toMovieLink
, andVIDEO
is corrected tovideo
. -
Added
MovieLink
class definition: This makes the code more complete and easier to understand. - Made minor adjustments to the annotation: to make it clearer and more accurate.
This makes the code easier to compile and run, and more compliant with C# coding conventions.
The above is the detailed content of How to Extract HtmlElements from Frames and IFrames in WebBrowser Control?. For more information, please follow other related articles on the PHP Chinese website!
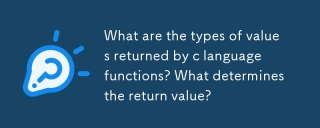
This article details C function return types, encompassing basic (int, float, char, etc.), derived (arrays, pointers, structs), and void types. The compiler determines the return type via the function declaration and the return statement, enforcing

Gulc is a high-performance C library prioritizing minimal overhead, aggressive inlining, and compiler optimization. Ideal for performance-critical applications like high-frequency trading and embedded systems, its design emphasizes simplicity, modul
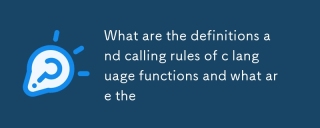
This article explains C function declaration vs. definition, argument passing (by value and by pointer), return values, and common pitfalls like memory leaks and type mismatches. It emphasizes the importance of declarations for modularity and provi

This article details C functions for string case conversion. It explains using toupper() and tolower() from ctype.h, iterating through strings, and handling null terminators. Common pitfalls like forgetting ctype.h and modifying string literals are
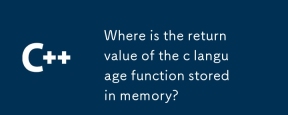
This article examines C function return value storage. Small return values are typically stored in registers for speed; larger values may use pointers to memory (stack or heap), impacting lifetime and requiring manual memory management. Directly acc
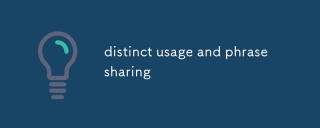
This article analyzes the multifaceted uses of the adjective "distinct," exploring its grammatical functions, common phrases (e.g., "distinct from," "distinctly different"), and nuanced application in formal vs. informal
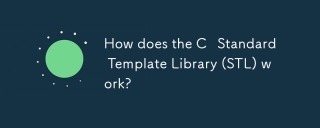
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
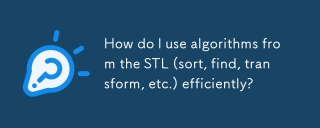
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
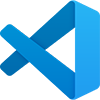
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
