


Use setDate() method in PreparedStatement
To improve code standardization, you need to replace hardcoded SQL variables with prepared statements and bind them. However, you have a problem using the setDate() method.
Original code
String vDateMDYSQL = vDateMDY; java.sql.Date date = new java.sql.Date(0000-00-00); ... prs.setDate(2, date.valueOf(vDateMDYSQL));
Error encountered
When executing the SQL statement, the following error occurred:
<code>java.lang.IllegalArgumentException at java.sql.Date.valueOf(Date.java:138)</code>
Alternatives
The problem is that the date variable is incorrectly initialized using 0000-00-00 as the default value. To resolve this issue, consider the following alternatives when dealing with dates:
java.sql.Date
For tables with DATE column type:
-
Use string:
ps.setDate(2, java.sql.Date.valueOf("2013-09-04"));
-
Use java.util.Date:
ps.setDate(2, new java.sql.Date(endDate.getTime()));
-
Get the current date:
ps.setDate(2, new java.sql.Date(System.currentTimeMillis()));
java.sql.Timestamp
For tables with TIMESTAMP or DATETIME column types:
-
Use string:
ps.setTimestamp(2, java.sql.Timestamp.valueOf("2013-09-04 13:30:00"));
-
Use java.util.Date:
ps.setTimestamp(2, new java.sql.Timestamp(endDate.getTime()));
-
Get the current timestamp:
ps.setTimestamp(2, new java.sql.Timestamp(System.currentTimeMillis()));
Use any of the above methods to correctly set the date in the prepared statement, thus avoiding the error you encountered.
The above is the detailed content of How to Correctly Use setDate() in Java PreparedStatements to Avoid `IllegalArgumentException`?. For more information, please follow other related articles on the PHP Chinese website!
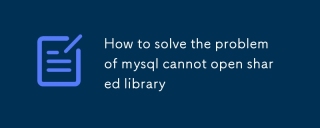
This article addresses MySQL's "unable to open shared library" error. The issue stems from MySQL's inability to locate necessary shared libraries (.so/.dll files). Solutions involve verifying library installation via the system's package m
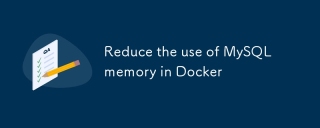
This article explores optimizing MySQL memory usage in Docker. It discusses monitoring techniques (Docker stats, Performance Schema, external tools) and configuration strategies. These include Docker memory limits, swapping, and cgroups, alongside
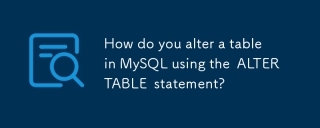
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
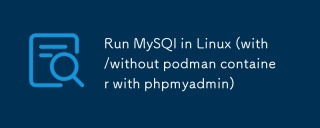
This article compares installing MySQL on Linux directly versus using Podman containers, with/without phpMyAdmin. It details installation steps for each method, emphasizing Podman's advantages in isolation, portability, and reproducibility, but also
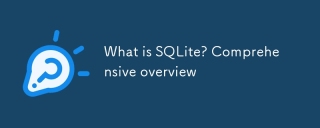
This article provides a comprehensive overview of SQLite, a self-contained, serverless relational database. It details SQLite's advantages (simplicity, portability, ease of use) and disadvantages (concurrency limitations, scalability challenges). C
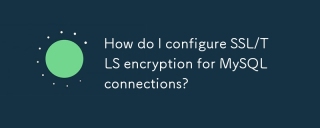
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
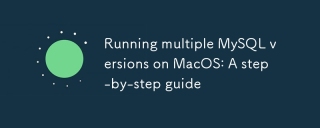
This guide demonstrates installing and managing multiple MySQL versions on macOS using Homebrew. It emphasizes using Homebrew to isolate installations, preventing conflicts. The article details installation, starting/stopping services, and best pra
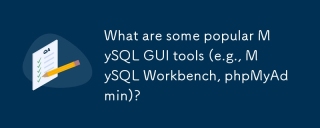
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
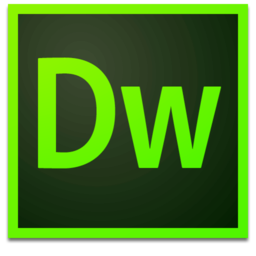
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
