Conditional statements are fundamental to any programming language. However, the way JavaScript and PHP handle "truthiness" and "falsiness"—determining whether a value is considered true or false in a conditional—differs significantly. This article explores these differences, focusing on empty arrays and objects, and their practical implications for web developers.
JavaScript's Truthiness and Falsiness
JavaScript's approach to truthiness is less intuitive than PHP's for many developers. It considers several values as "falsy":
const value1 = false; // Boolean false const value2 = 0; // Number zero const value3 = ""; // Empty string const value4 = null; // null const value5 = undefined; // undefined const value6 = NaN; // Not a Number
This also applies to reactive references in frameworks like Vue.js:
const ref1 = ref(false); const ref2 = ref(0); const ref3 = ref(""); const ref4 = ref(null); const ref5 = ref(undefined); const ref6 = ref(NaN);
Surprisingly, empty arrays and objects are considered "truthy":
const value7 = []; // Empty array const value8 = {}; // Empty object const value9 = "0"; // String "0"
The Memory Explanation
In JavaScript, empty arrays and objects are truthy because they represent valid memory references. Even though empty, they still occupy memory space.
// Arrays and Objects are memory references const emptyArray = []; // Valid memory reference const emptyObject = {}; // Valid memory reference Boolean([]) // true Boolean({}) // true Boolean(0) // false Boolean("") // false Boolean(null) // false Boolean(undefined) // false
This design choice stems from the fact that empty arrays and objects are still usable data structures. A reference, even to an empty container, differs from the absence of any value (null/undefined).
PHP's Approach
PHP adopts a more straightforward approach, treating empty data structures as "falsy." This is a key difference from JavaScript.
// Empty array is falsy $emptyArray = []; if (!$emptyArray) { echo "Empty array is false"; // This will print } // Empty object is also falsy $emptyObject = new stdClass(); if (!$emptyObject) { echo "Empty object is false"; // This will print }
Other falsy values in PHP include false
, 0
, 0.0
, ""
, null
, and empty arrays.
Explicit Empty Checks in JavaScript
To reliably check for empty arrays or objects in JavaScript, explicit checks are necessary:
//For an array [].length === 0 // true //For an object Object.keys({}).length === 0 // true
For reactive references:
const arrayRef = ref([]); const objectRef = ref({}); if (arrayRef.value.length === 0) { console.log('Array is empty'); } if (Object.keys(objectRef.value).length === 0) { console.log('Object is empty'); }
Empty Checks in PHP
PHP's simpler approach makes conditional logic cleaner:
$emptyArray = []; $emptyObject = new stdClass(); if (!$emptyArray) { echo "This will execute because empty arrays are falsy\n"; } if (!$emptyObject) { echo "This will execute because empty objects are falsy\n"; }
PHP's empty()
Function
PHP's empty()
function provides a convenient way to check for emptiness, including undefined variables:
empty(""); // true empty(0); // true empty([]); // true empty(new stdClass()); // true
empty()
is a language construct, not a function, so it cannot be used as a callback. isset()
, while useful for checking variable existence, can trigger warnings if used incorrectly with non-arrays.
Practical Implications
The contrasting approaches necessitate different coding styles. JavaScript demands explicit emptiness checks, potentially increasing code verbosity but improving clarity. PHP's approach offers concise code but may require extra checks for specific empty value types. Developers must be mindful of these differences when working with both languages, especially in cross-platform projects.
This understanding is crucial for developers bridging JavaScript and PHP, particularly those using frameworks like Laravel with React or Vue.js. Careful consideration of these nuances ensures reliable and predictable code behavior.
The above is the detailed content of The Differences in Truthiness and Falsiness in JavaScript vs PHP. For more information, please follow other related articles on the PHP Chinese website!
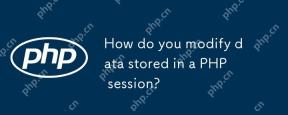
TomodifydatainaPHPsession,startthesessionwithsession_start(),thenuse$_SESSIONtoset,modify,orremovevariables.1)Startthesession.2)Setormodifysessionvariablesusing$_SESSION.3)Removevariableswithunset().4)Clearallvariableswithsession_unset().5)Destroythe
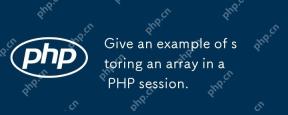
Arrays can be stored in PHP sessions. 1. Start the session and use session_start(). 2. Create an array and store it in $_SESSION. 3. Retrieve the array through $_SESSION. 4. Optimize session data to improve performance.
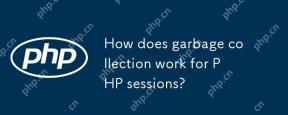
PHP session garbage collection is triggered through a probability mechanism to clean up expired session data. 1) Set the trigger probability and session life cycle in the configuration file; 2) You can use cron tasks to optimize high-load applications; 3) You need to balance the garbage collection frequency and performance to avoid data loss.
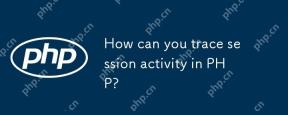
Tracking user session activities in PHP is implemented through session management. 1) Use session_start() to start the session. 2) Store and access data through the $_SESSION array. 3) Call session_destroy() to end the session. Session tracking is used for user behavior analysis, security monitoring, and performance optimization.
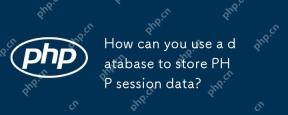
Using databases to store PHP session data can improve performance and scalability. 1) Configure MySQL to store session data: Set up the session processor in php.ini or PHP code. 2) Implement custom session processor: define open, close, read, write and other functions to interact with the database. 3) Optimization and best practices: Use indexing, caching, data compression and distributed storage to improve performance.
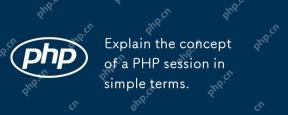
PHPsessionstrackuserdataacrossmultiplepagerequestsusingauniqueIDstoredinacookie.Here'showtomanagethemeffectively:1)Startasessionwithsession_start()andstoredatain$_SESSION.2)RegeneratethesessionIDafterloginwithsession_regenerate_id(true)topreventsessi
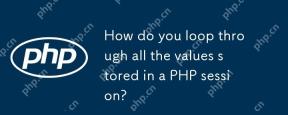
In PHP, iterating through session data can be achieved through the following steps: 1. Start the session using session_start(). 2. Iterate through foreach loop through all key-value pairs in the $_SESSION array. 3. When processing complex data structures, use is_array() or is_object() functions and use print_r() to output detailed information. 4. When optimizing traversal, paging can be used to avoid processing large amounts of data at one time. This will help you manage and use PHP session data more efficiently in your actual project.
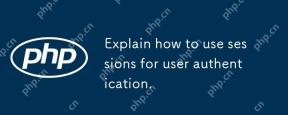
The session realizes user authentication through the server-side state management mechanism. 1) Session creation and generation of unique IDs, 2) IDs are passed through cookies, 3) Server stores and accesses session data through IDs, 4) User authentication and status management are realized, improving application security and user experience.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
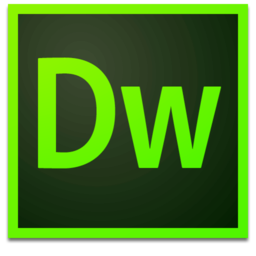
Dreamweaver Mac version
Visual web development tools
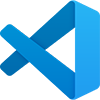
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
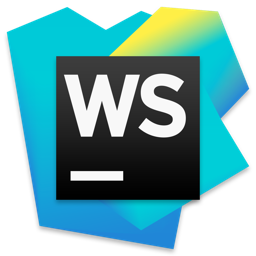
WebStorm Mac version
Useful JavaScript development tools
