This guide explores Angular Signals, a modern reactive programming paradigm simplifying state management. We'll examine their core functionality, including writable and computed signals, and demonstrate their use with effects. Practical examples will solidify your understanding.
Understanding Angular Signals
Angular Signals are a reactive primitive designed for more predictable and manageable state. Unlike older methods (Observables, EventEmitters), Signals offer a cleaner approach to reactive variables, automatically updating the view on state changes.
Key Signal Attributes:
- Synchronous Updates: Signal changes are immediately reflected.
- Dependency Tracking: Angular automatically tracks signal dependencies within components and directives.
- Intuitive Usage: Signals streamline state management compared to Observables.
Writable Signals: The Basics
Writable Signals are the fundamental building block, representing mutable reactive state.
Creating a Writable Signal:
Use the signal()
function:
import { signal } from '@angular/core'; export class CounterComponent { counter = signal(0); increment() { this.counter.update(val => val + 1); } decrement() { this.counter.update(val => val - 1); } }
Writable Signal Methods:
-
set(newValue)
: Directly assigns a new value.this.counter.set(10);
-
update(callback)
: Updates using a callback function.this.counter.update(val => val * 2);
-
mutate(callback)
: Directly mutates objects or arrays.this.arraySignal.mutate(arr => arr.push(5));
Computed Signals: Derived Values
Computed Signals derive their value from other signals, automatically updating when dependencies change. They are read-only.
Defining a Computed Signal:
Use the computed()
function:
import { signal, computed } from '@angular/core'; export class PriceCalculator { price = signal(100); quantity = signal(2); totalPrice = computed(() => this.price() * this.quantity()); }
Updating price
or quantity
automatically recalculates totalPrice
.
Effects: Managing Side Effects
Effects execute side effects (logging, API calls, DOM manipulation) when signals change.
Creating an Effect:
Use the effect()
function:
import { signal, effect } from '@angular/core'; export class EffectExample { counter = signal(0); constructor() { effect(() => console.log('Counter:', this.counter())); } increment() { this.counter.update(val => val + 1); } }
Effect Use Cases:
- Console logging
- HTTP requests
- External DOM manipulation
Complete Example: A Counter App
This counter app demonstrates writable, computed signals, and effects:
import { Component, signal, computed, effect } from '@angular/core'; @Component({ selector: 'app-counter', template: ` <div> <p>Counter: {{ counter() }}</p> <p>Double: {{ doubleCounter() }}</p> <button (click)="increment()">Increment</button> <button (click)="decrement()">Decrement</button> </div> ` }) export class CounterComponent { counter = signal(0); doubleCounter = computed(() => this.counter() * 2); constructor() { effect(() => console.log('Counter changed:', this.counter())); } increment() { this.counter.update(val => val + 1); } decrement() { this.counter.update(val => val - 1); } }
Signal Best Practices:
- Minimize Effects: Keep side effects concise.
- Use Computed Signals: For derived state, prefer
computed()
. - Avoid Over-Mutation: Favor
update()
overmutate()
. - Combine with Dependency Injection: Integrate signals with services for scalability.
Conclusion
Angular Signals provide a modern, efficient approach to reactive state management. Their simplicity and capabilities enhance developer experience and application performance, leading to more maintainable and predictable Angular code.
The above is the detailed content of Understanding Angular Signals: A Comprehensive Guide. For more information, please follow other related articles on the PHP Chinese website!
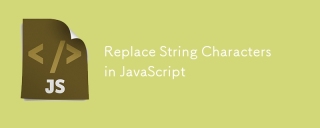
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
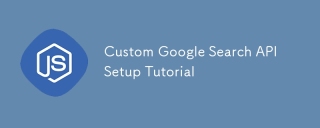
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
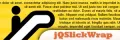
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
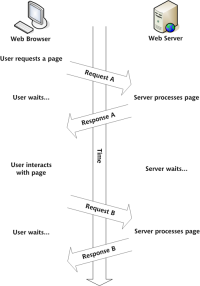
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
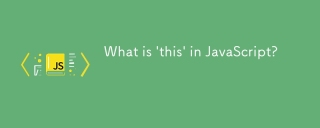
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
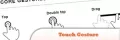
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig
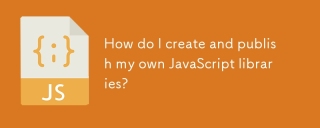
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
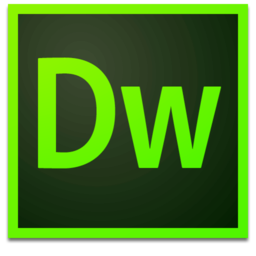
Dreamweaver Mac version
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
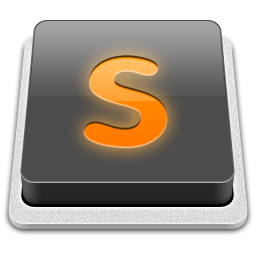
SublimeText3 Mac version
God-level code editing software (SublimeText3)
