


How Do I Access Variables and Functions Across Different Components in Unity?
Unity cross-component variable and function access
In Unity, components attached to game objects communicate and interact through a variety of mechanisms. There are some best practices and restrictions to consider when accessing variables or calling functions in different component scripts.
Public Access
To access a variable or function from other components, it must be declared public in the script. This means that these variables or functions can be accessed by scripts attached to other game objects in the scene. In contrast, a private variable or function can only be accessed within the script in which it is defined.
GetComponent function
To access a component's variables or call its functions from another script, typically use the GetComponent
function. This function receives a parameter of type Type
, which is the component type to be retrieved.
The following example demonstrates how to access the GetComponent
function:
GameObject tempObj = GameObject.Find("NameOfGameObjectScriptAIsAttachedTo"); ScriptA scriptInstance = tempObj.GetComponent<ScriptA>();
In this example, the GameObject.Find
function retrieves the game object named "NameOfGameObjectScriptAIsAttachedTo" (assuming ScriptA is attached to that object) and then uses the GetComponent
function to retrieve a reference to the ScriptA component.
Example implementation
Consider the following scenario: We have a playerScore
component with a public variable doSomething
and a function Player
. In another component named Enemy
, we want to access the playerScore
variable and call the doSomething
function.
in Enemy
component:
public class Enemy : MonoBehaviour { private ScriptA scriptAInstance = null; void Start() { GameObject tempObj = GameObject.Find("NameOfGameObjectScriptAIsAttachedTo"); scriptAInstance = tempObj.GetComponent<ScriptA>(); } void Update() { if (scriptAInstance != null) { scriptAInstance.playerScore += 1; scriptAInstance.doSomething(); } } }
In this example, the scriptAInstance
object is assigned a reference to the ScriptA
component attached to the game object named "NameOfGameObjectScriptAIsAttachedTo". Once we have this reference, we can access public Enemy
variables from the playerScore
component and call public doSomething
functions.
By following these guidelines, you can efficiently access variables and call functions in different component scripts to implement complex interactions and functionality in your Unity project. Note that a null check if (scriptAInstance != null)
has been added to prevent null reference exceptions.
The above is the detailed content of How Do I Access Variables and Functions Across Different Components in Unity?. For more information, please follow other related articles on the PHP Chinese website!
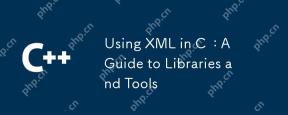
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
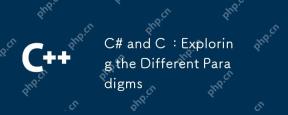
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
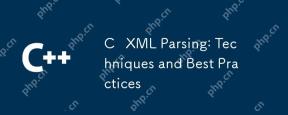
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
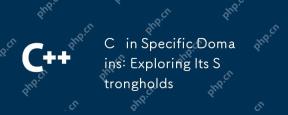
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
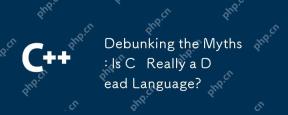
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
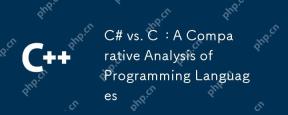
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
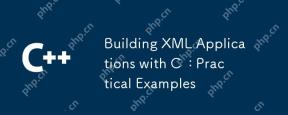
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
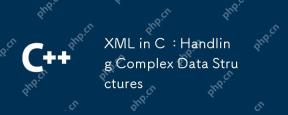
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
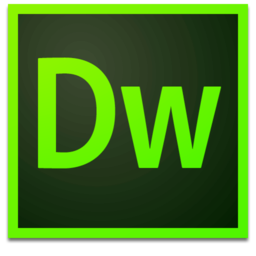
Dreamweaver Mac version
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
