I. A Deep Dive into Garbage Collection
In the realm of computer science, Garbage Collection (GC) is a crucial automatic memory management technique. It reclaims memory space no longer in use by a program, returning it to the operating system. This process utilizes various algorithms to efficiently identify and remove unused memory.
GC significantly reduces the programmer's workload and minimizes programming errors. Its origins trace back to the LISP programming language. Today, numerous languages, including Smalltalk, Java, C#, Go, and D, incorporate garbage collection mechanisms.
As a cornerstone of modern programming language memory management, GC's primary functions are twofold:
- Identifying and pinpointing unused memory resources (garbage).
- Clearing this garbage and freeing up the memory for other objects.
This automation frees programmers from the burden of manual memory management, allowing them to focus on core application logic. However, a fundamental understanding of GC remains essential for writing robust and efficient code.
II. Exploring Common Garbage Collection Algorithms
Several prominent algorithms power garbage collection:
-
Reference Counting: This method tracks the number of references to each object. When an object's reference count drops to zero, indicating no active references, the object is reclaimed. Python, PHP, and Swift utilize this approach.
- Advantages: Swift object recycling, and it doesn't wait for memory exhaustion or a specific threshold before acting.
- Disadvantages: Ineffective against circular references, and real-time reference counting adds overhead.
-
Mark-Sweep: This algorithm starts from root variables, marking all reachable objects. Unmarked objects, deemed unreachable, are then collected as garbage. Golang (using a tri-color marking method) and Python (as a supplementary mechanism) employ this technique.
- Advantages: Overcomes the limitations of reference counting.
- Disadvantages: Requires STW (Stop-The-World), temporarily halting program execution.
-
Generational Collection: This sophisticated approach divides memory into generations based on object lifespan. Long-lived objects reside in older generations, while short-lived objects are in newer generations. Different generations use varying recycling algorithms and frequencies. Java and Python (as a supplementary mechanism) leverage this method.
- Advantages: Excellent recycling performance.
- Disadvantages: Increased algorithm complexity.
III. Understanding Python's Garbage Collection
Python's memory management specifics depend on its implementation. CPython, the most common implementation, relies on reference counting for detecting inaccessible objects. However, it also includes a cycle-detecting mechanism to handle circular references. A cycle detection algorithm periodically identifies and removes these inaccessible cycles.
The gc
module provides tools for controlling garbage collection, accessing debugging statistics, and fine-tuning collector parameters. Other Python implementations (Jython, PyPy) may employ different mechanisms, such as a comprehensive garbage collector. Relying on reference counting behavior can introduce portability concerns.
-
Reference Counting in Python: Python's primary GC mechanism is reference counting. Each object maintains an
ob_ref
field tracking its references. Incrementing and decrementing this count reflects changes in references. A zero count triggers immediate object recycling.- Limitations: Requires extra space for reference counts and fails to address circular references, potentially leading to memory leaks. Consider this example:
a = {} # A's reference count is 1 b = {} # B's reference count is 1 a['b'] = b # B's reference count becomes 2 b['a'] = a # A's reference count becomes 2 del a # A's reference count is 1 del b # B's reference count is 1
<code>* After `del a` and `del b`, a circular reference exists. Reference counts aren't zero, preventing automatic cleanup.</code>
-
Mark-Sweep in Python: Python's supplementary mark-sweep algorithm, based on tracing GC, addresses circular references. It consists of two phases: marking active objects and sweeping away inactive ones. Starting from root objects, it traverses reachable objects, marking them as active. Unmarked objects are then collected. This primarily handles container objects (lists, dictionaries, etc.), as strings and numbers don't create circular references. Python utilizes a doubly linked list to manage these container objects.
- Drawbacks: Requires a full heap scan, even if only a small fraction of objects are inactive.
-
Generational Recycling in Python: This space-for-time trade-off divides memory into generations (young, middle, old) based on object age. Garbage collection frequency decreases with object age. Newly created objects start in the young generation, moving to older generations if they survive garbage collection cycles. This is also a supplementary mechanism, building upon mark-sweep.
IV. Addressing Memory Leaks
Memory leaks are uncommon in everyday Python use. However, CPython may not release all memory on exit in certain scenarios:
- Objects referenced from the global namespace or modules may persist, especially with circular references. Some C library-allocated memory might also remain.
- Python attempts to clean up memory upon exit, but this isn't always perfect.
- The
atexit
module allows running cleanup functions before program termination.
Code Example and Improvement:
a = {} # A's reference count is 1 b = {} # B's reference count is 1 a['b'] = b # B's reference count becomes 2 b['a'] = a # A's reference count becomes 2 del a # A's reference count is 1 del b # B's reference count is 1
Improved Code:
<code>* After `del a` and `del b`, a circular reference exists. Reference counts aren't zero, preventing automatic cleanup.</code>
Leapcell: Your Ideal Serverless Platform for Python Applications
Leapcell offers a superior solution for deploying Python services:
1. Versatile Language Support
Develop using JavaScript, Python, Go, or Rust.
2. Free and Unlimited Project Deployment
Pay only for actual usage – no idle charges.
3. Exceptional Cost-Effectiveness
Pay-as-you-go with no hidden fees. Example: $25 supports 6.94 million requests (60ms average response time).
4. Streamlined Developer Experience
User-friendly interface, automated CI/CD, GitOps integration, real-time metrics, and logging.
5. Effortless Scalability and High Performance
Automatic scaling handles high concurrency; zero operational overhead.
Learn more in the documentation!
Leapcell Twitter: https://www.php.cn/link/7884effb9452a6d7a7a79499ef854afd
The above is the detailed content of Python Garbage Collection: Everything You Need to Know. For more information, please follow other related articles on the PHP Chinese website!
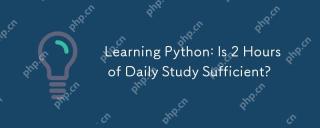
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
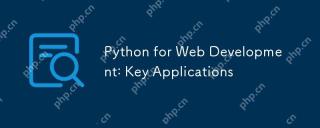
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
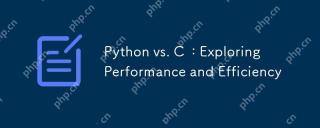
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
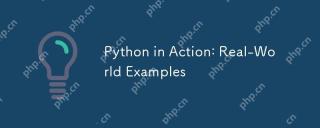
Python's real-world applications include data analytics, web development, artificial intelligence and automation. 1) In data analysis, Python uses Pandas and Matplotlib to process and visualize data. 2) In web development, Django and Flask frameworks simplify the creation of web applications. 3) In the field of artificial intelligence, TensorFlow and PyTorch are used to build and train models. 4) In terms of automation, Python scripts can be used for tasks such as copying files.
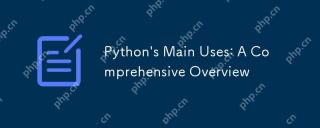
Python is widely used in data science, web development and automation scripting fields. 1) In data science, Python simplifies data processing and analysis through libraries such as NumPy and Pandas. 2) In web development, the Django and Flask frameworks enable developers to quickly build applications. 3) In automated scripts, Python's simplicity and standard library make it ideal.
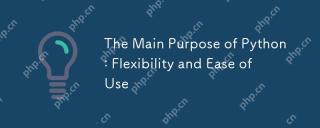
Python's flexibility is reflected in multi-paradigm support and dynamic type systems, while ease of use comes from a simple syntax and rich standard library. 1. Flexibility: Supports object-oriented, functional and procedural programming, and dynamic type systems improve development efficiency. 2. Ease of use: The grammar is close to natural language, the standard library covers a wide range of functions, and simplifies the development process.
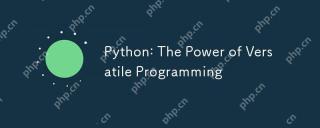
Python is highly favored for its simplicity and power, suitable for all needs from beginners to advanced developers. Its versatility is reflected in: 1) Easy to learn and use, simple syntax; 2) Rich libraries and frameworks, such as NumPy, Pandas, etc.; 3) Cross-platform support, which can be run on a variety of operating systems; 4) Suitable for scripting and automation tasks to improve work efficiency.
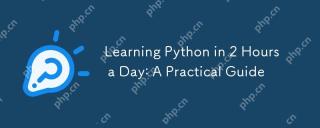
Yes, learn Python in two hours a day. 1. Develop a reasonable study plan, 2. Select the right learning resources, 3. Consolidate the knowledge learned through practice. These steps can help you master Python in a short time.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
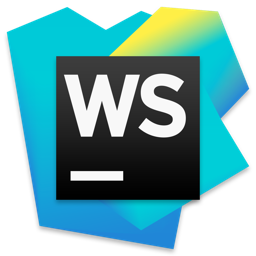
WebStorm Mac version
Useful JavaScript development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor