


Ever juggled multiple objects at once? Python tuples do just that! This guide explores Python tuple unpacking and its use in list element swapping. Understanding this allows efficient multiple variable assignment from tuples.
What are Tuples?
Think of a tuple as a secure, unchangeable container. Once an item is added, its position remains fixed.
Technically, a Python tuple is an immutable collection data type, similar to a list but with fixed contents after creation.
Tuples are defined using parentheses ()
, holding multiple items. For example:
my_tuple = (1, 2, 3)
The Power of Tuple Unpacking
Tuple unpacking is like a streamlined unboxing process; assigning multiple variables from a tuple simultaneously. For instance:
a, b = (1, 2)
a
becomes 1, and b
becomes 2.
Swapping Elements with Tuple Unpacking
Tuple unpacking simplifies value swapping, eliminating the need for temporary variables. Consider:
a = [65, 90, 80, 100]
To swap elements at indices 1 and 3:
a[1], a[3] = a[3], a[1]
The right side a[3], a[1]
creates a tuple (100, 90)
. The left side unpacks this, assigning 100 to a[1]
and 90 to a[3]
, effectively swapping them in a single line.
Why Tuple Immutability?
Tuples are like sealed containers; their contents cannot be altered after creation.
Technically, tuples are immutable; their elements cannot be modified individually.
For example:
scores = (95, 87, 92)
scores[0] = 96
# Raises a TypeError! Tuples are immutable.
To change values, a new tuple must be created.
Iterating Through Tuples
While tuples are immutable, their contents are accessible through iteration. This allows processing each element sequentially. For example:
# Student grades grades = ('A', 'B+', 'A-') for grade in grades: print(f"Got a {grade}!")
The loop assigns each tuple element ('A'
, 'B '
, 'A-'
) to grade
successively.
Key Takeaways
- Use tuples for data that shouldn't be modified.
- Tuple unpacking streamlines multiple variable assignments from tuples or sequences.
- It simplifies value swapping compared to using temporary variables.
- Iteration allows accessing each tuple element without altering the tuple itself.
- For frequently modified data, lists are preferable.
The above is the detailed content of Understanding Tuple Unpacking and Iteration in Python: A Beginners Guide. For more information, please follow other related articles on the PHP Chinese website!
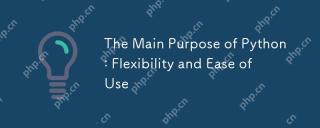
Python's flexibility is reflected in multi-paradigm support and dynamic type systems, while ease of use comes from a simple syntax and rich standard library. 1. Flexibility: Supports object-oriented, functional and procedural programming, and dynamic type systems improve development efficiency. 2. Ease of use: The grammar is close to natural language, the standard library covers a wide range of functions, and simplifies the development process.
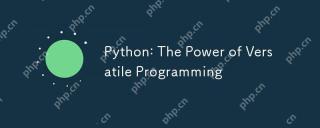
Python is highly favored for its simplicity and power, suitable for all needs from beginners to advanced developers. Its versatility is reflected in: 1) Easy to learn and use, simple syntax; 2) Rich libraries and frameworks, such as NumPy, Pandas, etc.; 3) Cross-platform support, which can be run on a variety of operating systems; 4) Suitable for scripting and automation tasks to improve work efficiency.
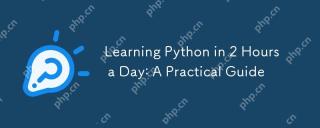
Yes, learn Python in two hours a day. 1. Develop a reasonable study plan, 2. Select the right learning resources, 3. Consolidate the knowledge learned through practice. These steps can help you master Python in a short time.
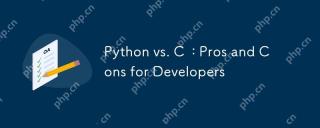
Python is suitable for rapid development and data processing, while C is suitable for high performance and underlying control. 1) Python is easy to use, with concise syntax, and is suitable for data science and web development. 2) C has high performance and accurate control, and is often used in gaming and system programming.
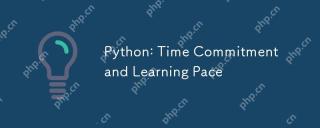
The time required to learn Python varies from person to person, mainly influenced by previous programming experience, learning motivation, learning resources and methods, and learning rhythm. Set realistic learning goals and learn best through practical projects.
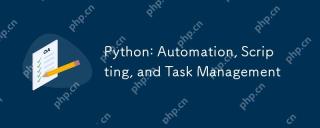
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
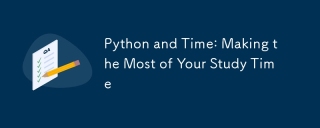
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
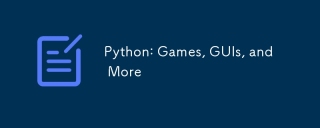
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
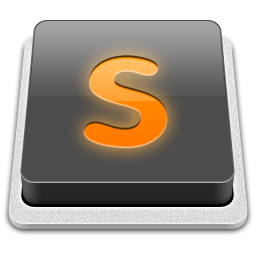
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.