Thread-Safe WPF UI Updates with Dispatcher.Invoke()
WPF applications often require UI updates from background threads, for instance, during lengthy data processing. Directly accessing UI elements from non-main threads, however, leads to cross-threading exceptions and unpredictable behavior. The solution lies in using Dispatcher.Invoke()
.
The WPF Dispatcher
is a crucial component enabling thread-safe communication with UI elements. Dispatcher.Invoke()
provides a mechanism to execute code on the main UI thread.
Understanding Dispatcher.Invoke()
Dispatcher.Invoke()
accepts an Action
delegate—the code to be executed on the UI thread. It blocks until the UI thread is available, then executes the delegate.
Illustrative Example: Progress Bar Update
Imagine updating a progress bar from a background thread:
// Background worker for data retrieval BackgroundWorker backgroundWorker = new BackgroundWorker(); // Progress reporting handler (runs on UI thread) backgroundWorker.ProgressChanged += (sender, e) => { // Safe progress bar update using Dispatcher.Invoke() Application.Current.Dispatcher.Invoke(DispatcherPriority.Background, () => { this.progressBar.Value = e.ProgressPercentage; }); }; // Initiate background work backgroundWorker.RunWorkerAsync();
Here, the ProgressChanged
event fires on the UI thread. The crucial step is using Dispatcher.Invoke()
to safely update the progressBar
.
Important Notes:
Dispatcher.Invoke()
is best suited for brief operations avoiding UI thread blockage. For lengthy tasks, employ BackgroundWorker
or Task
with appropriate continuation mechanisms.
Avoid long-running operations within Dispatcher.Invoke()
to prevent UI freezes. Consider asynchronous techniques like timers or async
/await
for smoother UI responsiveness.
The above is the detailed content of How Can I Safely Update WPF Controls from a Non-Main Thread?. For more information, please follow other related articles on the PHP Chinese website!
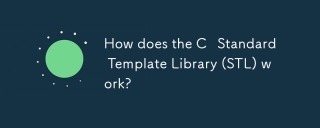
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
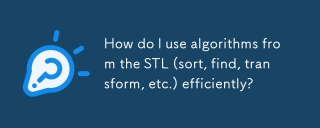
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
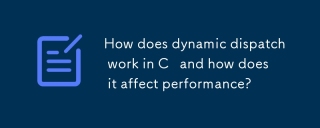
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
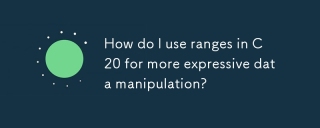
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
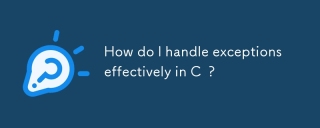
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
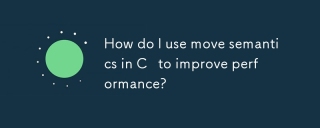
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
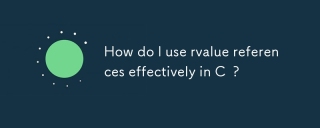
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
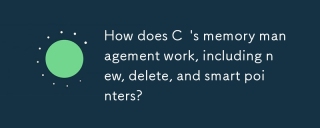
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
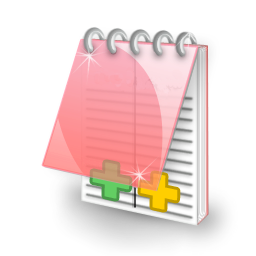
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
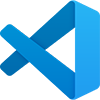
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
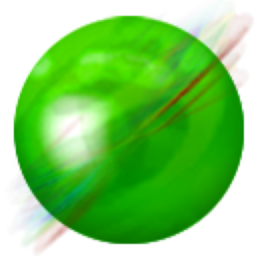
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
