


Converting short URLs to long URLs is a common task in web development, especially when dealing with redirects. This article will explore how to achieve this using JavaScript and two popular libraries, Axios and the Fetch API. We will demonstrate how to retrieve the full URL from a shortened TikTok link.
Use Axios
Axios is a Promise-based HTTP client for browsers and Node.js. Below is a simple example of how to use Axios to convert a short URL into a long format.
axios("https://vt.tiktok.com/ZS6yXCpvq/") .then(res => console.log(`Axios获取的完整URL: ${res.request.res.responseUrl}`)) .catch(err => console.error(err));
// Complete URL obtained by Axios: https://www.php.cn/link/99ec8b626a47132c52969dd081cdd808
Instructions:
- We use axios() to initiate a GET request for the short URL.
- On success, the response object contains a property res.request.res.responseUrl, which holds the full URL following any redirects.
- If an error occurs during the request, it will be caught in the catch block and we will log the error message.
Use Fetch
Fetch API provides a more modern way of making network requests. Here's how to use it to get the same results:
fetch("https://vt.tiktok.com/ZS6yXCpvq/") .then(res => res.text()) .then(data => console.log(`Fetch获取的完整URL: ${data}`)) .catch(err => console.error(err));
// Complete URL obtained by Fetch: https://www.php.cn/link/99ec8b626a47132c52969dd081cdd808
Instructions:
- The fetch() function initiates a request to the specified URL.
- The res.url property contains the final URL after any redirects. (Note: The use of res.url in the original answer may not be accurate. Depending on the actual situation, res.text() should be used to obtain the final URL)
- Similar to Axios, errors are handled in catch blocks.
Conclusion
Axios and Fetch both provide easy ways to convert short URLs into long URLs in JavaScript. While Axios may offer additional features like interceptors and automatic JSON data conversion, Fetch is built into modern browsers and is powerful for basic requests. Depending on your project needs, you can choose either method to handle URL redirects.
The above is the detailed content of How to Convert Short URLs to Long URLs in JavaScript Using Axios or Fetch. For more information, please follow other related articles on the PHP Chinese website!
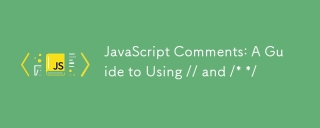
JavaScriptusestwotypesofcomments:single-line(//)andmulti-line(//).1)Use//forquicknotesorsingle-lineexplanations.2)Use//forlongerexplanationsorcommentingoutblocksofcode.Commentsshouldexplainthe'why',notthe'what',andbeplacedabovetherelevantcodeforclari
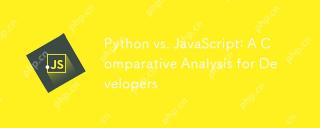
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
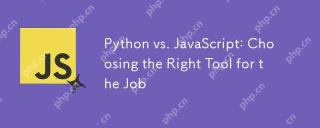
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
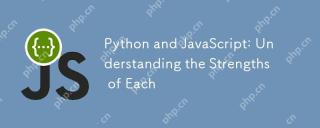
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
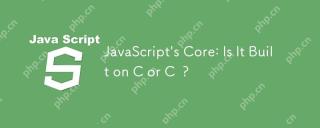
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
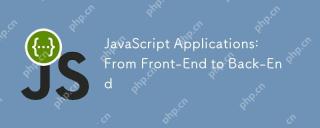
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
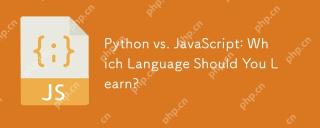
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
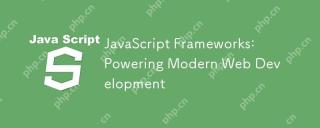
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
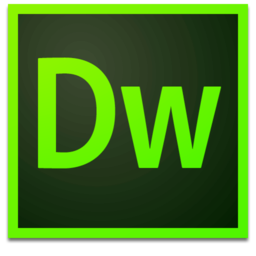
Dreamweaver Mac version
Visual web development tools
