


Tracer
Tracer is part of Observability which plays an important role in the implementation of Microservices Architecture and provides a 'Trace' overview of the processes running in an application logic.
Simply put, in Webservice, Tracer will provide an idea of how long the execution time of a logic is by emitting a Trace signal. Later, this Tracer will be visualized and seen in the form of a nested span after transmitting a signal through the Exporter to the Collector. [OpenTelemetry: Traces]
OpenTelemetry
To be able to transmit Traces signals which can later be collected by the Collector, Webservice requires OpenTelemetry as a library which has become a standard Observability protocol which is usually called OpenTelemetry Protocol (OTLP). [OpenTelemetry: Language - Go]
Jaeger
Visualization of Traces signals is really needed to provide an overview of what processes occur in the Webservice. Jaeger is an Open-Source platform that supports OTLP by utilizing the HTTP or gRPC communication protocol. [Jaeger]
Implementation in Golang
The implementation of Tracer in the Golang programming language will implement a simple case where the Webservice will only return data with different response durations. The library that will be used is:
- Chi: HTTP Framework
- OpenTelemetry: Telemetry Signaling
Setup OpenTelemetry as a Telemetry module
Implement the Telemetry module in the pkg/telemetry/telemetry.go directory:
package telemetry import ( "context" "errors" "time" "go.opentelemetry.io/otel" "go.opentelemetry.io/otel/exporters/otlp/otlptrace" "go.opentelemetry.io/otel/exporters/otlp/otlptrace/otlptracegrpc" "go.opentelemetry.io/otel/exporters/otlp/otlptrace/otlptracehttp" "go.opentelemetry.io/otel/sdk/resource" "go.opentelemetry.io/otel/sdk/trace" ) // enumeration constant for which protocol used const ( HTTP uint8 = iota GRPC ) // setup client to connect web-service with Jaeger func SetupTraceClient(ctx context.Context, protocol uint8, endpoint string) otlptrace.Client { switch protocol { case GRPC: return otlptracegrpc.NewClient(otlptracegrpc.WithEndpoint(endpoint), otlptracegrpc.WithInsecure(), otlptracegrpc.WithCompressor("gzip")) default: return otlptracehttp.NewClient(otlptracehttp.WithEndpoint(endpoint), otlptracehttp.WithInsecure(), otlptracehttp.WithCompression(otlptracehttp.NoCompression)) } } func setupTraceProvider(ctx context.Context, traceClient otlptrace.Client) (*trace.TracerProvider, error) { // set resource res, err := resource.New( ctx, resource.WithFromEnv(), ) if err != nil { return nil, err } // init trace exporter traceExporter, err := otlptrace.New(ctx, traceClient) if err != nil { return nil, err } // init trace exporter traceProvider := trace.NewTracerProvider( trace.WithBatcher( traceExporter, trace.WithBatchTimeout(time.Duration(time.Second*3)), ), trace.WithResource(res), // Discover and provide attributes from OTEL_RESOURCE_ATTRIBUTES and OTEL_SERVICE_NAME environment variables. ) return traceProvider, nil } func SetupTelemetrySDK(ctx context.Context, traceClient otlptrace.Client) (func(context.Context) error, error) { var err error var shutdownFuncs []func(context.Context) error shutdown := func(ctx context.Context) error { var err error for _, fn := range shutdownFuncs { err = errors.Join(err, fn(ctx)) } shutdownFuncs = nil return err } handleErr := func(inErr error) { err = errors.Join(inErr, shutdown(ctx)) } traceProvider, err := setupTraceProvider(ctx, traceClient) if err != nil { handleErr(err) return shutdown, err } shutdownFuncs = append(shutdownFuncs, traceProvider.Shutdown) otel.SetTracerProvider(traceProvider) return shutdown, nil }
Then, setup the Telemetry configuration in the main function main.go:
package main import ( "context" "fmt" "net/http" "os" "os/signal" "syscall" "time" "github.com/go-chi/chi/v5" "github.com/wahyurudiyan/medium/otel-jaeger/config" "github.com/wahyurudiyan/medium/otel-jaeger/pkg/telemetry" "github.com/wahyurudiyan/medium/otel-jaeger/router" ) func SetupTelemetry(ctx context.Context, config *config.Config) (func(context.Context) error, error) { otlpCli := telemetry.SetupTraceClient(ctx, telemetry.GRPC, config.JaegerGRPCEndpoint) shutdownFn, err := telemetry.SetupTelemetrySDK(ctx, otlpCli) return shutdownFn, err } func main() { ctx, cancel := context.WithTimeout(context.Background(), 5*time.Second) defer cancel() cfg := config.Get() shutdownFn, err := SetupTelemetry(ctx, cfg) if err != nil { shutdownFn(ctx) panic(err) } r := chi.NewRouter() r.Route("/api", func(r chi.Router) { router.Router(r) }) srv := http.Server{ Addr: "0.0.0.0:8080", Handler: r, } go func() { fmt.Println("Server running at port:", srv.Addr) if err := srv.ListenAndServe(); err != nil && err != http.ErrServerClosed { fmt.Printf("listen: %s\n", err) } }() quit := make(chan os.Signal, 1) signal.Notify(quit, syscall.SIGINT, syscall.SIGTERM) <p>Use Tracer in the handler in the router/router.go file to transmit Traces signals:<br> </p> <pre class="brush:php;toolbar:false">package router import ( "encoding/json" "net/http" "time" "github.com/go-chi/chi/v5" "github.com/wahyurudiyan/medium/otel-jaeger/pkg/random" "go.opentelemetry.io/otel" ) var ( tracer = otel.Tracer("WebServer-Otel-Jaeger") ) func getUserHandler(w http.ResponseWriter, r *http.Request) { _, span := tracer.Start(r.Context(), "GetUser") defer span.End() user := struct { Name string Email string Password string }{ Name: "John Doe", Email: "john@email.com", Password: "Super5ecr3t!", } blob, _ := json.Marshal(&user) sleepDuration := time.Duration(time.Millisecond * time.Duration(random.GenerateRandNum())) time.Sleep(sleepDuration) w.Header().Add("Content-Type", "application/json") w.WriteHeader(http.StatusOK) w.Write(blob) } func Router(router chi.Router) { router.Get("/user", getUserHandler) }
Deployments
Docker configuration for this Build Webservice, utilizing the Multi-Stage Build Image mechanism in the Dockerfile:
FROM golang:1.23.4 AS build WORKDIR /src COPY . . RUN go get -v RUN CGO_ENABLED=0 go build -o /bin/service . FROM alpine:latest WORKDIR /app COPY --from=build /bin/service /bin/service CMD ["/bin/service"]
Next, the image build will be carried out via the docker-compose.yaml file with the following configuration:
services: web-service: container_name: service build: context: . dockerfile: Dockerfile environment: OTEL_SERVICE_NAME: service-otel-jaeger JAEGER_GRPC_ENDPOINT: jaeger:4317 entrypoint: ["service"] ports: - 8080:8080 jaeger: container_name: jaeger image: jaegertracing/all-in-one:latest environment: COLLECTOR_ZIPKIN_HOST_PORT: :9411 ports: - 16686:16686 - 4317:4317 - 4318:4318 - 9411:9411
In service.jaeger.ports, the port exposed is the port for:
- 16686: Jaeger Dashboard
- 4317: Jaeger OTLP Protobuf with gRPC protocol
- 4318: Jaeger OTLP Protobuf/JSON with HTTP protocol
- 9411: Zipkin Collector
Run the application that has been defined in docker-compose.yaml, you can use the command:
docker compose up --build
After the application is running, you can try hitting the application on the endpoint http://127.0.0.1:8080/api/user, if the Webservice and application are connected, the service name will appear as in the picture.
a span will appear to define how long it takes to run a process.
Load Test
Now let's try using the CLI tool hey [https://github.com/rakyll/hey] to run a load-test. The following command can be used to perform a simple load-test:
package telemetry import ( "context" "errors" "time" "go.opentelemetry.io/otel" "go.opentelemetry.io/otel/exporters/otlp/otlptrace" "go.opentelemetry.io/otel/exporters/otlp/otlptrace/otlptracegrpc" "go.opentelemetry.io/otel/exporters/otlp/otlptrace/otlptracehttp" "go.opentelemetry.io/otel/sdk/resource" "go.opentelemetry.io/otel/sdk/trace" ) // enumeration constant for which protocol used const ( HTTP uint8 = iota GRPC ) // setup client to connect web-service with Jaeger func SetupTraceClient(ctx context.Context, protocol uint8, endpoint string) otlptrace.Client { switch protocol { case GRPC: return otlptracegrpc.NewClient(otlptracegrpc.WithEndpoint(endpoint), otlptracegrpc.WithInsecure(), otlptracegrpc.WithCompressor("gzip")) default: return otlptracehttp.NewClient(otlptracehttp.WithEndpoint(endpoint), otlptracehttp.WithInsecure(), otlptracehttp.WithCompression(otlptracehttp.NoCompression)) } } func setupTraceProvider(ctx context.Context, traceClient otlptrace.Client) (*trace.TracerProvider, error) { // set resource res, err := resource.New( ctx, resource.WithFromEnv(), ) if err != nil { return nil, err } // init trace exporter traceExporter, err := otlptrace.New(ctx, traceClient) if err != nil { return nil, err } // init trace exporter traceProvider := trace.NewTracerProvider( trace.WithBatcher( traceExporter, trace.WithBatchTimeout(time.Duration(time.Second*3)), ), trace.WithResource(res), // Discover and provide attributes from OTEL_RESOURCE_ATTRIBUTES and OTEL_SERVICE_NAME environment variables. ) return traceProvider, nil } func SetupTelemetrySDK(ctx context.Context, traceClient otlptrace.Client) (func(context.Context) error, error) { var err error var shutdownFuncs []func(context.Context) error shutdown := func(ctx context.Context) error { var err error for _, fn := range shutdownFuncs { err = errors.Join(err, fn(ctx)) } shutdownFuncs = nil return err } handleErr := func(inErr error) { err = errors.Join(inErr, shutdown(ctx)) } traceProvider, err := setupTraceProvider(ctx, traceClient) if err != nil { handleErr(err) return shutdown, err } shutdownFuncs = append(shutdownFuncs, traceProvider.Shutdown) otel.SetTracerProvider(traceProvider) return shutdown, nil }
The command will run a load-test for 100 requests per second (RPS) for 10 minutes. The results that will appear on the Jaeger UI page will look like the following.
If the load test has finished running, there will be a report of the load test results.
package main import ( "context" "fmt" "net/http" "os" "os/signal" "syscall" "time" "github.com/go-chi/chi/v5" "github.com/wahyurudiyan/medium/otel-jaeger/config" "github.com/wahyurudiyan/medium/otel-jaeger/pkg/telemetry" "github.com/wahyurudiyan/medium/otel-jaeger/router" ) func SetupTelemetry(ctx context.Context, config *config.Config) (func(context.Context) error, error) { otlpCli := telemetry.SetupTraceClient(ctx, telemetry.GRPC, config.JaegerGRPCEndpoint) shutdownFn, err := telemetry.SetupTelemetrySDK(ctx, otlpCli) return shutdownFn, err } func main() { ctx, cancel := context.WithTimeout(context.Background(), 5*time.Second) defer cancel() cfg := config.Get() shutdownFn, err := SetupTelemetry(ctx, cfg) if err != nil { shutdownFn(ctx) panic(err) } r := chi.NewRouter() r.Route("/api", func(r chi.Router) { router.Router(r) }) srv := http.Server{ Addr: "0.0.0.0:8080", Handler: r, } go func() { fmt.Println("Server running at port:", srv.Addr) if err := srv.ListenAndServe(); err != nil && err != http.ErrServerClosed { fmt.Printf("listen: %s\n", err) } }() quit := make(chan os.Signal, 1) signal.Notify(quit, syscall.SIGINT, syscall.SIGTERM) <h2> Github Project </h2> <p>For those who want to try or see the full code, you can clone the following repository: https://github.com/wahyurudiyan/otel-jaeger.</p>
The above is the detailed content of [Language] Tracer: Open Telemetry, Golang, and Jagger Simple Implementation. For more information, please follow other related articles on the PHP Chinese website!
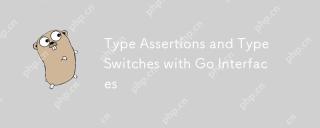
Gohandlesinterfacesandtypeassertionseffectively,enhancingcodeflexibilityandrobustness.1)Typeassertionsallowruntimetypechecking,asseenwiththeShapeinterfaceandCircletype.2)Typeswitcheshandlemultipletypesefficiently,usefulforvariousshapesimplementingthe
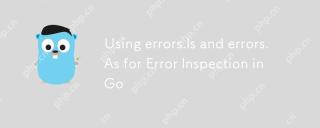
Go language error handling becomes more flexible and readable through errors.Is and errors.As functions. 1.errors.Is is used to check whether the error is the same as the specified error and is suitable for the processing of the error chain. 2.errors.As can not only check the error type, but also convert the error to a specific type, which is convenient for extracting error information. Using these functions can simplify error handling logic, but pay attention to the correct delivery of error chains and avoid excessive dependence to prevent code complexity.
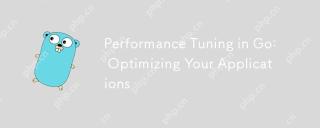
TomakeGoapplicationsrunfasterandmoreefficiently,useprofilingtools,leverageconcurrency,andmanagememoryeffectively.1)UsepprofforCPUandmemoryprofilingtoidentifybottlenecks.2)Utilizegoroutinesandchannelstoparallelizetasksandimproveperformance.3)Implement
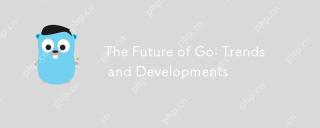
Go'sfutureisbrightwithtrendslikeimprovedtooling,generics,cloud-nativeadoption,performanceenhancements,andWebAssemblyintegration,butchallengesincludemaintainingsimplicityandimprovingerrorhandling.
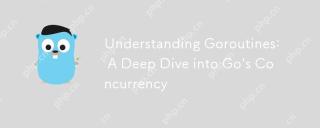
GoroutinesarefunctionsormethodsthatrunconcurrentlyinGo,enablingefficientandlightweightconcurrency.1)TheyaremanagedbyGo'sruntimeusingmultiplexing,allowingthousandstorunonfewerOSthreads.2)Goroutinesimproveperformancethrougheasytaskparallelizationandeff
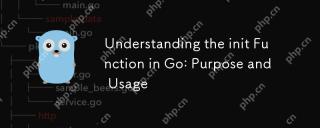
ThepurposeoftheinitfunctioninGoistoinitializevariables,setupconfigurations,orperformnecessarysetupbeforethemainfunctionexecutes.Useinitby:1)Placingitinyourcodetorunautomaticallybeforemain,2)Keepingitshortandfocusedonsimpletasks,3)Consideringusingexpl
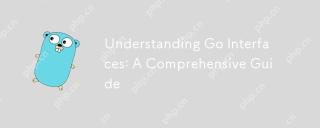
Gointerfacesaremethodsignaturesetsthattypesmustimplement,enablingpolymorphismwithoutinheritanceforcleaner,modularcode.Theyareimplicitlysatisfied,usefulforflexibleAPIsanddecoupling,butrequirecarefulusetoavoidruntimeerrorsandmaintaintypesafety.
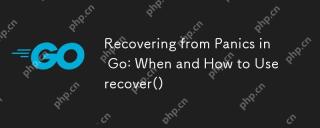
Use the recover() function in Go to recover from panic. The specific methods are: 1) Use recover() to capture panic in the defer function to avoid program crashes; 2) Record detailed error information for debugging; 3) Decide whether to resume program execution based on the specific situation; 4) Use with caution to avoid affecting performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
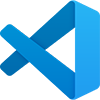
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
