While I generally avoid classes in my TypeScript projects, favoring functions for their simplicity and tree-shaking benefits, using classes with Svelte's reactive $state
variables can offer performance advantages. Rich Harris himself suggests this approach in certain situations. The key is that classes bypass the compilation overhead associated with getters and setters for $state
variables.
A Sharable Rune Class
This requires a sharable Rune
class leveraging Svelte's context API.
// rune.svelte.ts import { getContext, hasContext, setContext } from "svelte"; type RCurrent<TValue> = { current: TValue }; export class Rune<TRune> { readonly #key: symbol; constructor(name: string) { this.#key = Symbol(name); } exists(): boolean { return hasContext(this.#key); } get(): RCurrent<TRune> { return getContext(this.#key); } init(value: TRune): RCurrent<TRune> { const _value = $state({ current: value }); // Assuming '$state' is available from a library like 'svelte-state' return setContext(this.#key, _value); } update(getter: () => TRune): void { const context = this.get(); $effect(() => { // Assuming '$effect' is available from a library like 'svelte-state' context.current = getter(); }); } }
We can then export custom runes as needed:
// counter.svelte.ts import { Rune } from "./rune.svelte"; export const counter = new Rune<number>('counter');
This method, while similar to common $state
sharing techniques, offers server-side compatibility (as discussed in previous posts). Context naming follows standard conventions.
Initialization
Initialization of the $state
variable happens only once, within the parent component:
<script> import { counter } from '$lib/counter.svelte'; const count = counter.init(0); </script>
Reading the Value
Child components can safely access and read the current value:
<script> import { counter } from '$lib/counter.svelte'; const count = counter.get(); </script> <h1 id="Hello-from-Child-count-current">Hello from Child: {count.current}</h1> <button on:click={() => count.current++}>Increment From Child</button>
Reactive Updates
Updating the shared $state
requires a function to trigger reactivity:
<script> import { counter } from '$lib/counter.svelte'; let value = $state(8); // Assuming '$state' is available counter.update(() => value); </script>
This enables reactive updates, handling signals and store variables similarly to $derived
.
Direct Updates
Direct updates are also supported:
<script> import { counter } from '$lib/counter.svelte'; const count = counter.get(); count.current = 9; </script>
- Repo Link (Please provide if available)
- Demo Link (Please provide if available)
This approach provides a structured and performant way to manage shared reactive state in Svelte applications.
The above is the detailed content of Sharing Runes in Svelte ith the Rune Class. For more information, please follow other related articles on the PHP Chinese website!
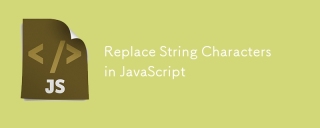
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
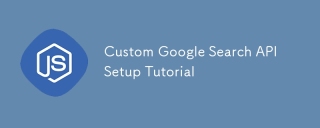
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
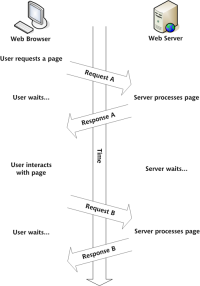
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
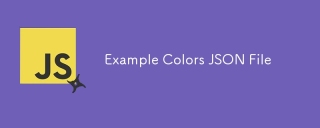
This article series was rewritten in mid 2017 with up-to-date information and fresh examples. In this JSON example, we will look at how we can store simple values in a file using JSON format. Using the key-value pair notation, we can store any kind
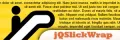
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
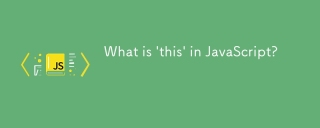
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
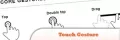
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
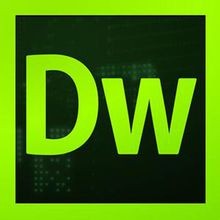
Dreamweaver CS6
Visual web development tools
