


Loading Assemblies and Dependencies into a Separate AppDomain: A Recursive Approach
Loading assemblies with intricate dependencies into a new AppDomain can present challenges. Errors such as "Could not load file or assembly... or one of its dependencies" frequently occur because referenced assemblies aren't automatically loaded. This requires a manual, recursive loading process.
The solution involves these key steps:
-
AppDomain Creation: First, establish a new AppDomain:
AppDomainSetup setup = AppDomain.CurrentDomain.SetupInformation; setup.ApplicationBase = dir; AppDomain domain = AppDomain.CreateDomain("SomeAppDomain", null, setup);
-
Loading the Main Assembly: Load the primary assembly using its
AssemblyName
:domain.Load(AssemblyName.GetAssemblyName(path));
-
Recursive Reference Resolution: Iterate through the references of the loaded assembly within the new AppDomain:
foreach (AssemblyName refAsmName in Assembly.ReflectionOnlyLoadFrom(path).GetReferencedAssemblies()) { // Utilize a proxy object for cross-AppDomain access Type type = typeof(Proxy); var value = (Proxy)domain.CreateInstanceAndUnwrap(type.Assembly.FullName, type.FullName); // Load the referenced assembly in the target AppDomain value.GetAssembly(refAsmName.FullName); }
-
Proxy Class for Cross-Domain Interaction: The
Proxy
class facilitates communication between AppDomains:class Proxy : MarshalByRefObject { public Assembly GetAssembly(string assemblyPath) { try { return Assembly.LoadFile(assemblyPath); } catch (Exception) { return null; } } }
This method ensures that all necessary dependencies are loaded recursively into the target AppDomain, preventing runtime errors and enabling successful assembly execution.
The above is the detailed content of How to Recursively Load Assemblies and Their Dependencies into a Separate AppDomain?. For more information, please follow other related articles on the PHP Chinese website!
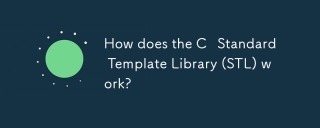
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
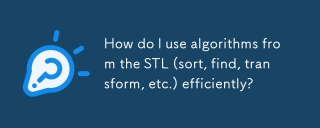
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
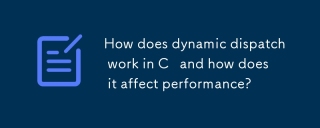
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
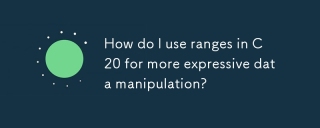
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
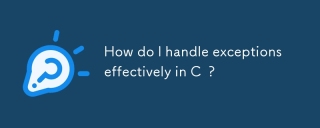
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
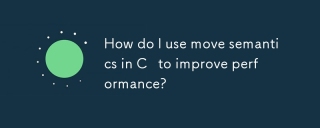
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
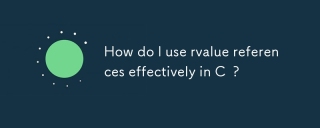
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
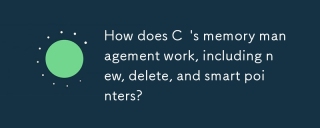
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor
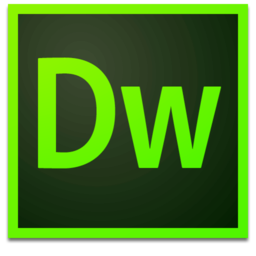
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
