


Introduction: Navigating the Cybersecurity Landscape
The 2016 Mirai botnet attack, crippling major online services, highlighted the vulnerability of everyday devices. This underscores the critical need for practical cybersecurity training. This guide provides a hands-on exploration of modern cyber threats, focusing on the techniques attackers employ. We’ll dissect malware behavior, command and control systems, data exfiltration methods, evasion tactics, and persistence mechanisms, all illustrated with Python code examples. The goal isn't to create malicious software, but to understand how these threats function to better defend against them. This is a journey into the intricacies of cyberattacks—knowledge that empowers stronger defenses.
Malware Behavior: Evolving Threats
Polymorphic malware constantly changes its code to evade detection. The following Python script demonstrates a basic form of payload obfuscation using Base64 encoding:
import random import string import base64 def generate_payload(): payload = ''.join(random.choices(string.ascii_letters + string.digits, k=50)) obfuscated_payload = base64.b64encode(payload.encode()).decode() with open('payload.txt', 'w') as f: f.write(obfuscated_payload) print("[+] Generated obfuscated payload:", obfuscated_payload) generate_payload()
Note: This is a simplified example. Real-world malware uses far more sophisticated techniques like runtime encryption and metamorphic engines to constantly rewrite its code. Defenders use heuristic analysis and behavior-based detection to identify such threats.
Command and Control (C&C) Infrastructures: Decentralized Networks
Decentralized botnets, using peer-to-peer (P2P) communication, are harder to shut down. The following Python snippet simulates a basic encrypted P2P system:
import socket import threading import ssl import random peers = [('127.0.0.1', 5001), ('127.0.0.1', 5002)] # ... (rest of the P2P code remains the same) ...
Note: Real-world P2P botnets employ advanced encryption, dynamic peer discovery, and authentication mechanisms for enhanced resilience and security.
Data Exfiltration: Concealing Stolen Information
Steganography hides data within seemingly harmless files, like images. The following script demonstrates a basic steganography technique:
from PIL import Image import zlib # ... (steganography code remains the same) ...
Note: Advanced steganography techniques and robust anomaly detection systems are used in real-world scenarios. Steganalysis tools are employed by defenders to detect hidden data.
Evasion Strategies: Timing Attacks
Malware can delay execution to avoid detection by sandboxes. The following script simulates a simple delay tactic:
import time import random import os def delayed_execution(): delay = random.randint(60, 300) if os.getenv('SANDBOX'): delay *= 10 print(f"[*] Delaying execution by {delay} seconds...") time.sleep(delay) print("[+] Executing payload.") delayed_execution()
Persistence Mechanisms: Ensuring Survival
Malware uses various techniques to survive reboots. The following script simulates registry-based persistence in Windows:
import winreg as reg import os import time def add_to_startup(file_path): key = reg.HKEY_CURRENT_USER subkey = r'Software\Microsoft\Windows\CurrentVersion\Run' while True: with reg.OpenKey(key, subkey, 0, reg.KEY_SET_VALUE) as open_key: reg.SetValueEx(open_key, 'SystemUpdate', 0, reg.REG_SZ, file_path) print("[+] Ensured persistence in startup registry.") time.sleep(60) add_to_startup(os.path.abspath(__file__))
Note: Linux and macOS use different methods like cron jobs or launch agents.
(Deployment and Implementation Guide, Ethical Considerations, and Full Updated Script sections remain largely the same, with minor wording adjustments for consistency and clarity.)
Conclusion: Building a Stronger Defense
This hands-on exploration provides a foundation for understanding and countering real-world cyber threats. Continue your learning through ethical penetration testing, CTF competitions, open-source contributions, and relevant certifications. Remember, in cybersecurity, continuous learning is crucial for staying ahead of evolving threats. Apply this knowledge responsibly and ethically to strengthen cybersecurity defenses.
The above is the detailed content of Building a Smarter Botnet Simulation: The Ultimate Cybersecurity Playground. For more information, please follow other related articles on the PHP Chinese website!
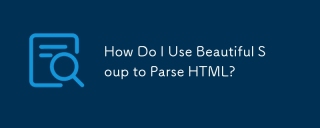
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
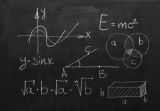
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
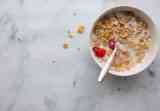
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
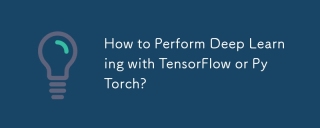
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
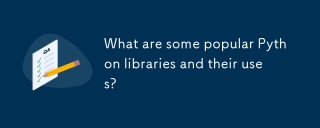
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
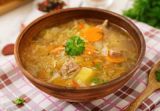
This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex
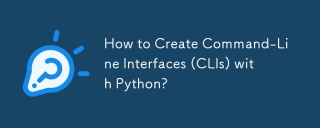
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.
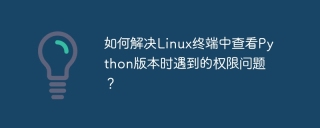
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
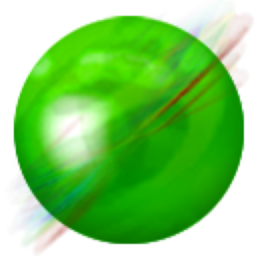
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
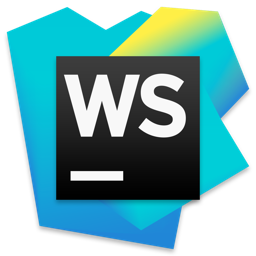
WebStorm Mac version
Useful JavaScript development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
