Use custom enum value name in System.Text.Json
You can use the JsonConverter
class to specify custom names for enumeration values. Here’s how:
- Define a custom converter:
public class CustomEnumStringEnumConverter : JsonConverter<Enum> { protected override Enum Read(ref Utf8JsonReader reader, Type typeToConvert, JsonSerializerOptions options) { // 从JSON文本解析枚举名称 var name = reader.GetString(); // 获取枚举类型 var enumType = typeToConvert; // 按名称查找枚举值 Enum value; if (!Enum.TryParse(enumType, name, true, out value)) { throw new JsonException("无效的枚举值: " + name); } return value; } protected override void Write(Utf8JsonWriter writer, Enum value, JsonSerializerOptions options) { // 获取枚举值的自定义名称 var customName = GetCustomName(value); // 将自定义名称写入JSON文本 writer.WriteStringValue(customName); } private string GetCustomName(Enum value) { // 获取枚举值的字段信息 var fieldInfo = enumType.GetField(value.ToString()); // 获取应用于该字段的自定义属性 var attribute = fieldInfo.GetCustomAttribute<EnumMemberAttribute>(); // 返回属性中的自定义名称,如果未指定则返回默认名称 return attribute?.Value ?? value.ToString(); } }
- Register the converter using JsonSerializerOptions:
var options = new JsonSerializerOptions { Converters = { new CustomEnumStringEnumConverter() } };
- Apply the EnumMember attribute:
Use the EnumMember
attribute to decorate the enumeration value to specify a custom name:
public enum Example { [EnumMember(Value = "Trick-Or-Treat")] TrickOrTreat, // 其他枚举值 }
- Serialization and deserialization:
You can now serialize and deserialize enums using custom JsonConverter
s:
// 序列化枚举值 var json = JsonSerializer.Serialize(value, options); // 反序列化枚举值 var value = JsonSerializer.Deserialize<Enum>(json, options);
Instructions:
- This method requires .NET Core 3.1 or higher.
- If you need to use a custom converter for multiple enumeration types, you can create a generic converter.
- This method allows you to specify a custom name for the enumeration value while maintaining the ability to round-trip the enumeration value during serialization and deserialization.
The above is the detailed content of How to Use Custom Enum Value Names with System.Text.Json?. For more information, please follow other related articles on the PHP Chinese website!
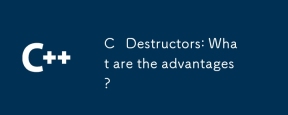
C destructorsprovideseveralkeyadvantages:1)Theymanageresourcesautomatically,preventingleaks;2)Theyenhanceexceptionsafetybyensuringresourcerelease;3)TheyenableRAIIforsaferesourcehandling;4)Virtualdestructorssupportpolymorphiccleanup;5)Theyimprovecode
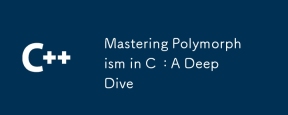
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
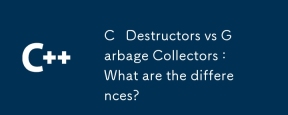
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
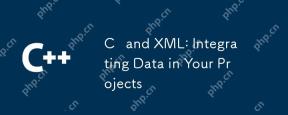
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
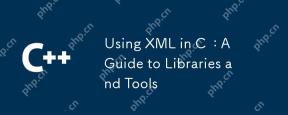
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
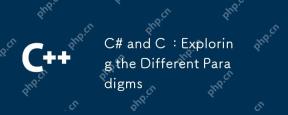
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
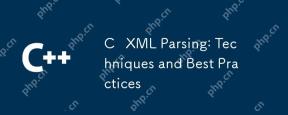
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
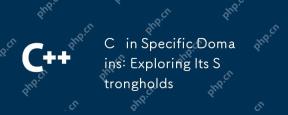
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
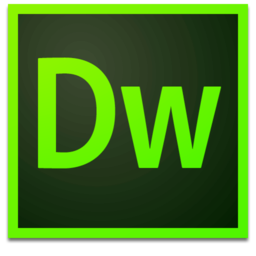
Dreamweaver Mac version
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!
