JSON deserialization of dynamically named sub-objects in C#
Handling deserialization of JSON structures with dynamically named sub-objects can be challenging. Consider the following JSON example:
{ "users": { "parentname": "test", "100034": { "name": "tom", "state": "WA", "id": "cedf-c56f-18a4-4b1" }, "10045": { "name": "steve", "state": "NY", "id": "ebb2-92bf-3062-7774" }, "12345": { "name": "mike", "state": "MA", "id": "fb60-b34f-6dc8-aaf7" } } }
Attempts to deserialize using the following code may fail:
public class RootObject { public string ParentName { get; set; } public Dictionary<string, User> users { get; set; } } public class User { public string name { get; set; } public string state { get; set; } public string id { get; set; } }
This is because RootObject
the known property names in the class do not match the dynamic names of the child objects in the JSON.
The solution is to use a custom converter to handle the dynamic property names and deserialize them into a dictionary of strongly typed objects. To do this, you need:
-
Create a converter class that inherits from
JsonConverter
and provide deserialization and serialization logic:public class TypedExtensionDataConverter<T> : JsonConverter // ... 实现略 ...
-
Use the
[JsonTypedExtensionData]
attribute to mark the attribute in the data model that will hold the dictionary of dynamically named objects:[JsonConverter(typeof(TypedExtensionDataConverter<User>))] class Users { [JsonProperty("parentname")] public string ParentName { get; set; } [JsonTypedExtensionData] public Dictionary<string, User> UserTable { get; set; } }
-
Updated data model to correctly handle dynamic sub-objects:
public class RootObject { [JsonProperty("users")] public Users Users { get; set; } }
By using a custom converter, JSON structures can be successfully deserialized into a strongly typed C# object model, thus preserving the hierarchical structure and dynamic nature of the original data.
The above is the detailed content of How to Deserialize JSON with Dynamically Named Child Objects in C#?. For more information, please follow other related articles on the PHP Chinese website!
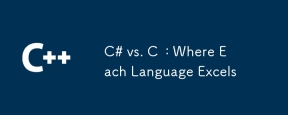
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
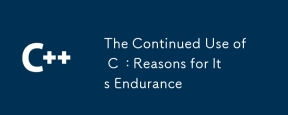
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
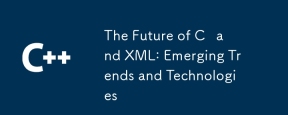
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
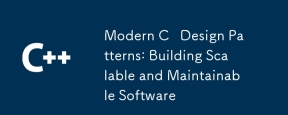
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
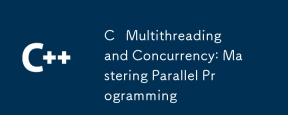
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
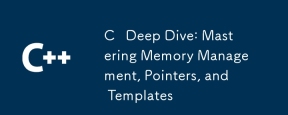
C's memory management, pointers and templates are core features. 1. Memory management manually allocates and releases memory through new and deletes, and pay attention to the difference between heap and stack. 2. Pointers allow direct operation of memory addresses, and use them with caution. Smart pointers can simplify management. 3. Template implements generic programming, improves code reusability and flexibility, and needs to understand type derivation and specialization.
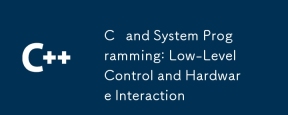
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.
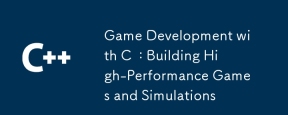
C is suitable for building high-performance gaming and simulation systems because it provides close to hardware control and efficient performance. 1) Memory management: Manual control reduces fragmentation and improves performance. 2) Compilation-time optimization: Inline functions and loop expansion improve running speed. 3) Low-level operations: Direct access to hardware, optimize graphics and physical computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
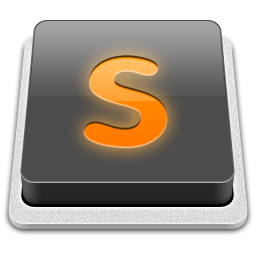
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
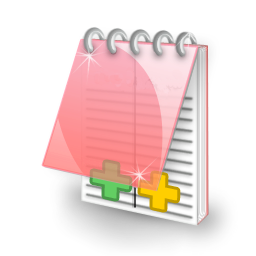
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function