Static vs Instance Variables
Whenever a variable is declared as static, this means there is only one copy of it for the entire class, rather than each instance having its own copy.
Static variable
When a static variable is declared, a single copy of the variable is created and shared among all objects at the class level. Static variables are, essentially, global variables. All instances of the class share the same static variable.
Important points to note on static variables
We can only create static variables at the class level.
static block and static variables are executed in the order they are present in a program.
Static variables can be called directly with the help of the class only, we do not need to create an object for the class in this.
// Java program to demonstrate execution // of static blocks and variables class Test { // static variable static int a = m1(); // static block static { System.out.println("Inside static block"); } // static method static int m1() { System.out.println("from m1"); return 20; } // static method(main !!) public static void main(String[] args) { System.out.println("Value of a : " + a); System.out.println("from main"); } }
The results from the above code:
from m1 Inside static block Value of a : 20 from main
Static methods can call another static methods or variables using the classname. They can not call an instance method or variable.
Instance methods can call static methods or variables using a className or reference variable. They can call another instance method or variable using instance variables.
The Final Keyword
When a final keyword is marked static, you can not override it. In Java, the final keyword is used to indicate that a variable, method, or class cannot be modified or extended.When a variable is declared as final, its value cannot be changed once it has been initialized.
Designing static methods and fields
Static methods do not require an instance of a class. They are shared among all users of the class. There is only one copy of the code for the instance methods. Each instance of a class can call it as many times as it would like.
when a class loads for the first time the static variables will be given memory. For static variable methods, we use the className instead of the object eg className.variable
Having static methods eliminates the need for the caller to instantiate the object just to call the method. You can also use an instance of an object to call a static method. The compiler will check for the type of the reference and use that instead of the object.
If you make a static reference to a non-static method, you will get a Compiler error.
Only instance methods can call other instance methods on the same class without using a reference variable. Instance methods do require an object.
Key takeaways:
- An instance method can call a static method
- A static method can not call an instance method
- Static variables can not use instance variables
- Constant variables (status variables) are meant to never change and use the final specifier to make sure the variable never changes.
Final variables can be reassigned when the statickeyword is the first assignment eg If we declare a static final variable, it means we can initialize it exactly once in a static block. If a final variable is declared and never initialised, we get a compiler error.
Feel free to add your knowledge about Static and Instance variables in the comments below.
The above is the detailed content of Static variables in Java. For more information, please follow other related articles on the PHP Chinese website!
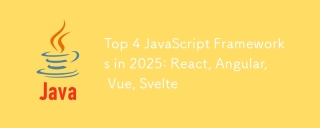
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
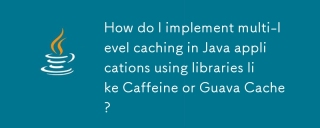
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
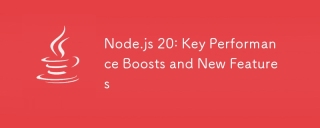
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
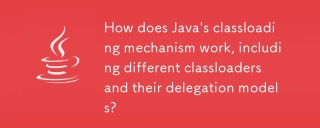
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
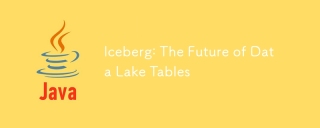
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
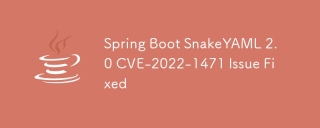
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
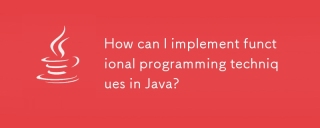
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
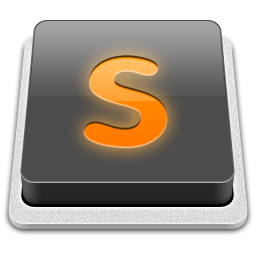
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
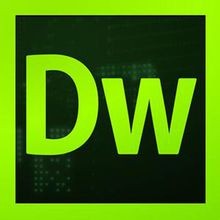
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
