


Passing a list of strings to a SQL Server stored procedure using a user-defined table type
When calling a SQL Server stored procedure from C#, you may need to pass a list of strings as parameters. While standard parameters are sufficient for handling simple data types, for complex data structures such as lists, a more sophisticated approach is required.
To pass a list of strings, we can leverage the User-Defined Table Type (UDTT) feature of SQL Server. This feature allows us to create a custom data type that simulates a table to store a collection of data.
Create user-defined table type
First, we need to create a User-Defined Table Type (UDTT):
CREATE TYPE [dbo].[StringList] AS TABLE( [Item] [NVARCHAR](MAX) NULL );
This UDTT defines a single column named "Item" to hold strings.
Modify stored procedure
Next, we modify the stored procedure to accept UDTT parameters:
CREATE PROCEDURE [dbo].[sp_UseStringList] @list StringList READONLY AS BEGIN -- 只返回我们传入的项目 SELECT l.Item FROM @list l; END
Populating UDTT in C#
In C# we use DataTable to populate UDTT:
using (var table = new DataTable()) { table.Columns.Add("Item", typeof(string)); // ...此处添加循环填充字符串列表到DataTable的代码... SqlParameter pList = new SqlParameter("@list", SqlDbType.Structured); pList.Value = table; }
Pass UDTT parameters to stored procedures
Finally, we pass the UDTT parameters to the stored procedure:
using (var cmd = new SqlCommand("exec sp_UseStringList @list", con)) { cmd.Parameters.Add(pList); using (var dr = cmd.ExecuteReader()) { while (dr.Read()) Console.WriteLine(dr["Item"].ToString()); } }
This approach allows us to efficiently pass a list of strings to a SQL Server stored procedure.
The above is the detailed content of How to Pass a List of Strings to a SQL Server Stored Procedure Using a User-Defined Table Type?. For more information, please follow other related articles on the PHP Chinese website!
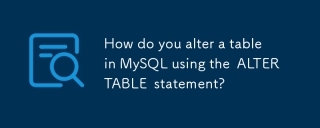
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
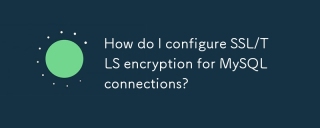
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
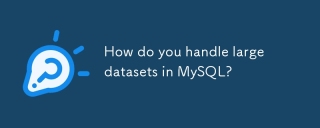
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
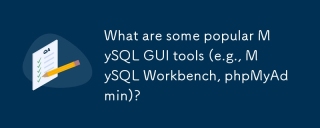
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
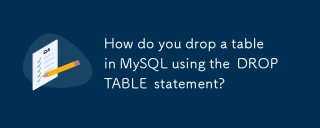
The article discusses dropping tables in MySQL using the DROP TABLE statement, emphasizing precautions and risks. It highlights that the action is irreversible without backups, detailing recovery methods and potential production environment hazards.
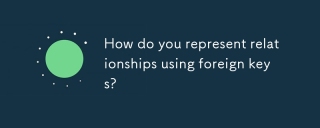
Article discusses using foreign keys to represent relationships in databases, focusing on best practices, data integrity, and common pitfalls to avoid.
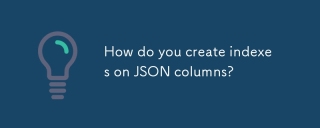
The article discusses creating indexes on JSON columns in various databases like PostgreSQL, MySQL, and MongoDB to enhance query performance. It explains the syntax and benefits of indexing specific JSON paths, and lists supported database systems.
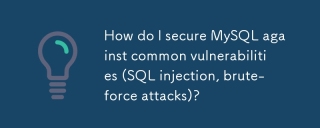
Article discusses securing MySQL against SQL injection and brute-force attacks using prepared statements, input validation, and strong password policies.(159 characters)


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
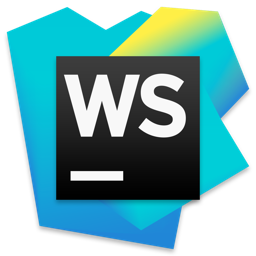
WebStorm Mac version
Useful JavaScript development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment
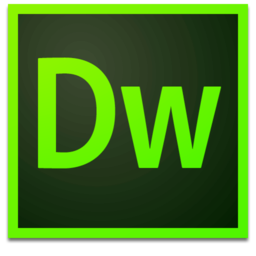
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
