How to test Laravel’s Storage::temporaryUrl() method
Laravel provides a powerful and flexible Storage facade for file storage and operations. One feature worth noting is temporaryUrl()
, which can generate temporary URLs for files stored on services like Amazon S3 or DigitalOcean Spaces. However, Laravel's documentation does not cover how to effectively test this method. Testing this can be challenging, especially when using Storage::fake
as the mock storage driver does not support temporaryUrl()
and will throw the following error:
This driver does not support creating temporary URLs.
In this article, we will demonstrate two methods of testing Storage::temporaryUrl()
through a practical example. These methods include emulating a file system and using emulated storage. Both methods ensure your tests remain isolated and reliable.
Example settings
We will illustrate the testing process using a PriceExport
model, corresponding controllers and test cases. Here are the settings:
Model
final class PriceExport extends Model { protected $fillable = [ 'user_id', 'supplier_id', 'path', 'is_auto', 'is_ready', 'is_send', ]; public function user(): BelongsTo { return $this->belongsTo(User::class); } public function supplier(): BelongsTo { return $this->belongsTo(Supplier::class); } }
Controller
The controller uses the temporaryUrl
method to generate a temporary URL for the file:
final class PriceExportController extends Controller { /** * @throws ItemNotFoundException */ public function download(PriceExport $priceExport): DownloadFileResource { if (!$priceExport->is_ready || empty($priceExport->path)) { throw new ItemNotFoundException('price export'); } $fileName = basename($priceExport->path); $diskS3 = Storage::disk(StorageDiskName::DO_S3->value); $url = $diskS3->temporaryUrl($priceExport->path, Carbon::now()->addHour()); $downloadFileDTO = new DownloadFileDTO($url, $fileName); return DownloadFileResource::make($downloadFileDTO); } }
Test temporaryUrl()
Test Case 1: Using Storage::fake
While Storage::fake
does not natively support temporaryUrl
, we can simulate a mock store to emulate the behavior of this method. This approach ensures that you can test without the need for a real storage service.
final class PriceExportTest extends TestCase { public function test_price_export_download_fake(): void { // 安排 $user = $this->getDefaultUser(); $this->actingAsFrontendUser($user); $supplier = SupplierFactory::new()->create(); $priceExport = PriceExportFactory::new()->for($user)->for($supplier)->create([ 'path' => 'price-export/price-2025.xlsx', ]); $expectedUrl = 'https://temporary-url.com/supplier-price-export-2025.xlsx'; $expectedFileName = basename($priceExport->path); $fakeFilesystem = Storage::fake(StorageDiskName::DO_S3->value); // 模拟模拟文件系统 $proxyMockedFakeFilesystem = Mockery::mock($fakeFilesystem); $proxyMockedFakeFilesystem->shouldReceive('temporaryUrl')->andReturn($expectedUrl); Storage::set(StorageDiskName::DO_S3->value, $proxyMockedFakeFilesystem); // 操作 $response = $this->postJson(route('api-v2:price-export.price-exports.download', $priceExport)); // 断言 $response->assertOk()->assertJson([ 'data' => [ 'name' => $expectedFileName, 'url' => $expectedUrl, ] ]); } }
Test Case 2: Using Storage::shouldReceive
This method utilizes Laravel's built-in simulation capabilities to directly simulate the behavior of temporaryUrl
.
final class PriceExportTest extends TestCase { public function test_price_export_download_mock(): void { // 安排 $user = $this->getDefaultUser(); $this->actingAsFrontendUser($user); $supplier = SupplierFactory::new()->create(); $priceExport = PriceExportFactory::new()->for($user)->for($supplier)->create([ 'path' => 'price-export/price-2025.xlsx', ]); $expectedUrl = 'https://temporary-url.com/supplier-price-export-2025.xlsx'; $expectedFileName = basename($priceExport->path); // 模拟存储行为 Storage::shouldReceive('disk')->with(StorageDiskName::DO_S3->value)->andReturnSelf(); Storage::shouldReceive('temporaryUrl')->andReturn($expectedUrl); // 操作 $response = $this->postJson(route('api-v2:price-export.price-exports.download', $priceExport)); // 断言 $response->assertOk()->assertJson([ 'data' => [ 'name' => $expectedFileName, 'url' => $expectedUrl, ] ]); } }
Key Points
-
Limitations of Storage::fake: Not supported by the simulated storage driver
temporaryUrl
. Use a simulated version of the simulated store to resolve this issue. -
Mock Storage: Laravel's
Storage::shouldReceive
simplifies the process of mocking methods liketemporaryUrl
when testing controllers. - Isolation: Both methods ensure that your tests are not dependent on external services, keeping your tests fast and reliable.
By combining these techniques, you can effectively test Storage::temporaryUrl()
and ensure that your application functionality is fully verified.
The above is the detailed content of Testing Temporary URLs in Laravel Storage. For more information, please follow other related articles on the PHP Chinese website!
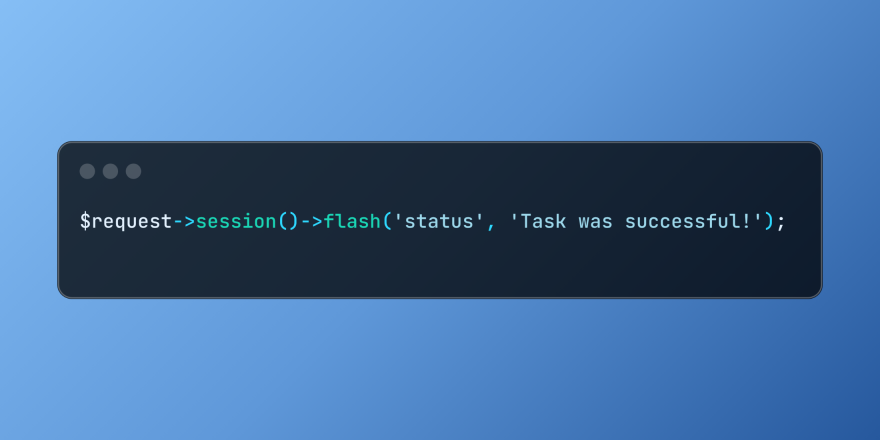
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
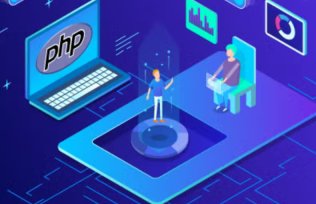
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
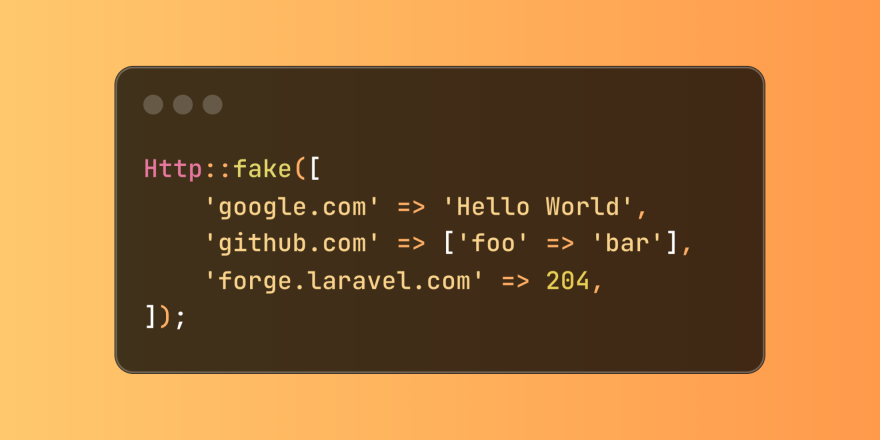
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
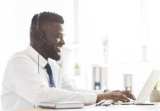
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
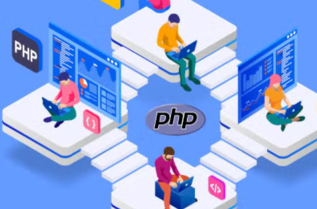
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
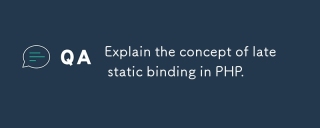
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
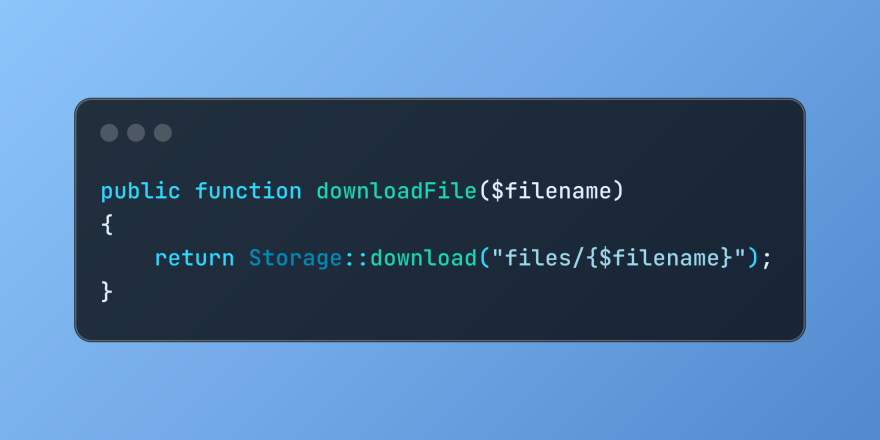
The Storage::download method of the Laravel framework provides a concise API for safely handling file downloads while managing abstractions of file storage. Here is an example of using Storage::download() in the example controller:
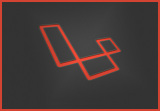
Laravel's service container and service providers are fundamental to its architecture. This article explores service containers, details service provider creation, registration, and demonstrates practical usage with examples. We'll begin with an ove


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
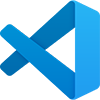
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
