How to bind WPF buttons to commands in ViewModelBase
In WPF (Windows Presentation Foundation), connecting user interface (UI) elements to commands defined in the view model (such as ViewModelBase) is crucial to enable data binding and reactive applications. This article provides a detailed solution for binding a button's click event to a command in ViewModelBase.
Problem Overview
The original problem was that the buttons in the AttributeView were not able to trigger the commands in the ViewModelBase class when clicked. The button's binding code is not bound to the command.
Solution implementation
To solve this problem, we need to follow the correct WPF binding syntax and ensure that the ViewModelBase class contains the required properties and bindings. Here is the updated code:
<grid><grid.columndefinitions><columndefinition width="*"></columndefinition></grid.columndefinitions> </grid>
// MainWindow.xaml.cs public partial class MainWindow : Window { public MainWindow() { InitializeComponent(); DataContext = new ViewModelBase(); } }
// ViewModelBase.cs public class ViewModelBase : INotifyPropertyChanged // Added INotifyPropertyChanged { private ICommand _clickCommand; public ICommand ClickCommand { get { return _clickCommand ?? (_clickCommand = new CommandHandler(() => MyAction(), () => CanExecute)); } } public bool CanExecute { get { // 定义命令是否可执行的逻辑 // 在此示例中,始终返回true return true; } } public void MyAction() { // 实现按钮单击时要执行的操作 } public event PropertyChangedEventHandler PropertyChanged; protected virtual void OnPropertyChanged([CallerMemberName] string propertyName = null) { PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName)); } }
// CommandHandler.cs public class CommandHandler : ICommand { private Action _action; private Func<bool> _canExecute; public CommandHandler(Action action, Func<bool> canExecute) { _action = action; _canExecute = canExecute; } public event EventHandler CanExecuteChanged { add { CommandManager.RequerySuggested += value; } remove { CommandManager.RequerySuggested -= value; } } public bool CanExecute(object parameter) { return _canExecute.Invoke(); } public void Execute(object parameter) { _action(); } }
Description
The provided code uses the MVVM (Model-View-ViewModel) pattern. The ClickCommand property in ViewModelBase is bound to the button's Command property. The CommandHandler class is responsible for performing an action (MyAction) and determining whether the command can be executed (CanExecute). By implementing this binding, buttons can now trigger commands when clicked. The ViewModelBase
class adds the INotifyPropertyChanged
interface and implements the OnPropertyChanged
method to ensure that property changes are correctly reflected on the UI.
Conclusion
Binding buttons to commands in a ViewModelBase using WPF requires correct syntax and a command handler class to manage command execution and validation. By adhering to the principles outlined in this article, developers can effectively connect UI elements to commands and improve the responsiveness of their applications.
The above is the detailed content of How to Bind a WPF Button's Click Event to a Command in a ViewModelBase?. For more information, please follow other related articles on the PHP Chinese website!
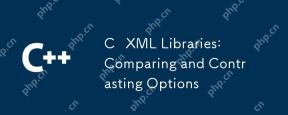
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
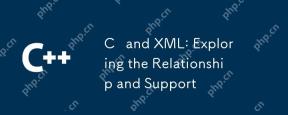
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
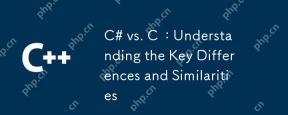
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
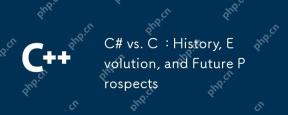
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
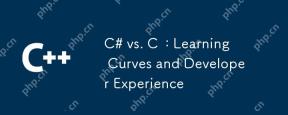
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
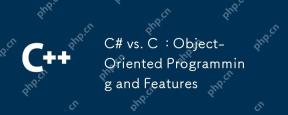
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
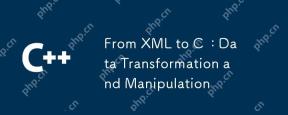
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
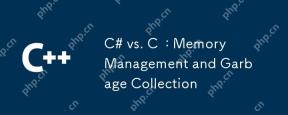
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
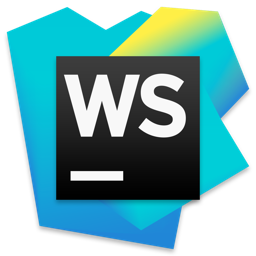
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
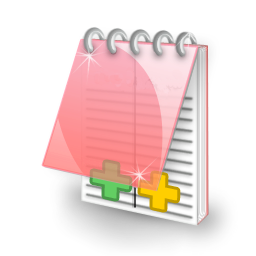
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software