Getting Started Guide to Enumerations in Java and C#
Developers moving from Java to C# may find that C#'s enumerations seem to be simpler than Java's implementation. Let’s explore the differences between Java and C# enumerations and how to overcome them.
Main Differences
- Method support: Java enums support defining methods, which C# enums traditionally do not.
Use extension methods to overcome differences
In C#, you can create extension methods for enumerations to add methods such as surfaceGravity()
and surfaceWeight()
. For example:
using System; class PlanetAttr : Attribute { internal PlanetAttr(double mass, double radius) { this.Mass = mass; this.Radius = radius; } public double Mass { get; private set; } public double Radius { get; private set; } } public static class Planets { public static double GetSurfaceGravity(this Planet p) { PlanetAttr attr = GetAttr(p); return G * attr.Mass / (attr.Radius * attr.Radius); } public static double G = 6.67300E-11; private static PlanetAttr GetAttr(Planet p) { return (PlanetAttr)Attribute.GetCustomAttribute(ForValue(p), typeof(PlanetAttr)); } private static MemberInfo ForValue(Planet p) { return typeof(Planet).GetField(Enum.GetName(typeof(Planet), p)); } } public enum Planet { [PlanetAttr(3.303e+23, 2.4397e6)] MERCURY, // ... other planets }
With this code, you can access the Planet
methods of each GetSurfaceGravity()
enumeration value.
Summary
While Java enums offer more built-in functionality, C# enums offer greater flexibility through extension methods. By implementing method extensions, you can replicate the functionality of a Java enumeration and customize the enumeration to your specific needs.
The above is the detailed content of Java vs. C# Enums: How Do Extension Methods Bridge the Gap?. For more information, please follow other related articles on the PHP Chinese website!
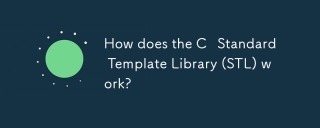
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
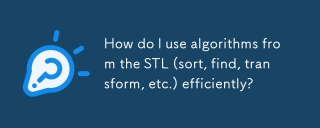
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
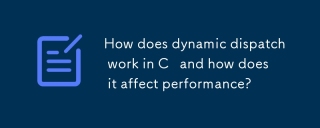
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
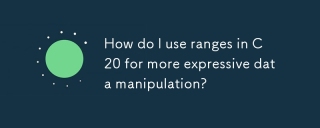
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
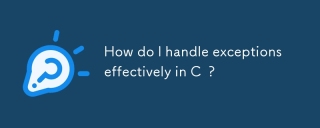
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
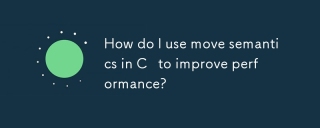
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
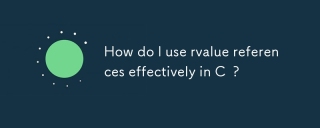
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
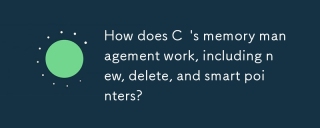
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
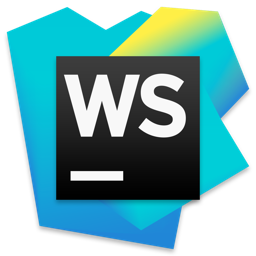
WebStorm Mac version
Useful JavaScript development tools
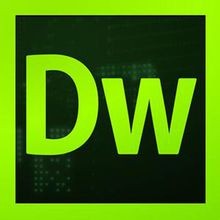
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use
