


Optimizing Bulk Data Insertion into SQL Server with C#
Handling large datasets often necessitates efficient database insertion techniques. When dealing with web-sourced data, parameterized queries are crucial for security and performance. This article demonstrates a superior method to the common, inefficient row-by-row approach.
Addressing Inefficient Single-Row Inserts
Iterative insertion using individual INSERT
statements (e.g., INSERT INTO DATABASE('web',@data)
) is slow and vulnerable to SQL injection.
The Solution: Table-Valued Parameters (TVPs)
SQL Server 2008 and later versions support Table-Valued Parameters (TVPs), offering a highly efficient method for bulk insertion while maintaining parameterization for security.
1. Defining a User-Defined Table Type (UDT)
Begin by creating a UDT that mirrors the structure of your target database table:
CREATE TYPE MyTableType AS TABLE ( Col1 int, Col2 varchar(20) ) GO
2. Creating a Stored Procedure with a TVP
Next, create a stored procedure accepting the TVP:
CREATE PROCEDURE MyProcedure ( @MyTable dbo.MyTableType READONLY -- READONLY is crucial for TVPs! ) AS INSERT INTO MyTable (Col1, Col2) SELECT Col1, Col2 FROM @MyTable GO
3. C# Implementation using the TVP
The C# code utilizes a DataTable
to hold the data before insertion:
DataTable dt = new DataTable(); dt.Columns.Add("Col1", typeof(int)); dt.Columns.Add("Col2", typeof(string)); // Populate the DataTable with your data here using (var con = new SqlConnection("ConnectionString")) { using (var cmd = new SqlCommand("MyProcedure", con)) { cmd.CommandType = CommandType.StoredProcedure; cmd.Parameters.AddWithValue("@MyTable", dt); // Simplified parameter addition con.Open(); cmd.ExecuteNonQuery(); } }
This approach leverages TVPs for significantly faster and more secure bulk data insertion compared to individual INSERT
statements. The use of AddWithValue
simplifies parameter handling. Remember to replace "ConnectionString"
with your actual connection string.
The above is the detailed content of How Can I Efficiently Bulk Insert Parameterized Data into SQL Server Using C#?. For more information, please follow other related articles on the PHP Chinese website!
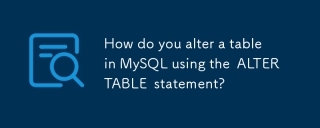
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
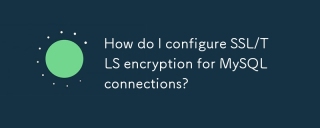
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
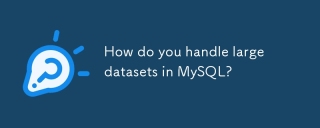
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
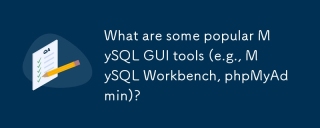
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
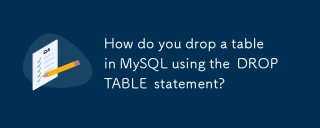
The article discusses dropping tables in MySQL using the DROP TABLE statement, emphasizing precautions and risks. It highlights that the action is irreversible without backups, detailing recovery methods and potential production environment hazards.
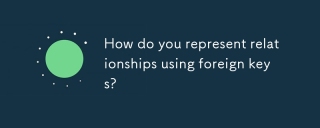
Article discusses using foreign keys to represent relationships in databases, focusing on best practices, data integrity, and common pitfalls to avoid.
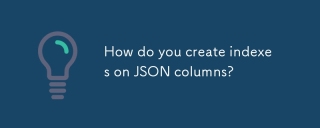
The article discusses creating indexes on JSON columns in various databases like PostgreSQL, MySQL, and MongoDB to enhance query performance. It explains the syntax and benefits of indexing specific JSON paths, and lists supported database systems.
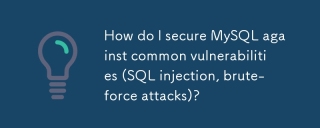
Article discusses securing MySQL against SQL injection and brute-force attacks using prepared statements, input validation, and strong password policies.(159 characters)


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
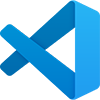
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
