Integrating External Executables into Your C# Applications
Many C# applications require the ability to run external executable files (.exe). This article demonstrates how to seamlessly launch external executables and pass arguments using C#.
Utilizing the Process.Start
Method:
The core method for launching executables within C# is Process.Start
. This method accepts a file path as its argument, initiating the corresponding application. For instance, to run a simple executable without arguments:
Process.Start("C:\path\to\your\executable.exe");
Passing Arguments to External Executables:
To pass command-line arguments, use the ProcessStartInfo
class. This provides finer control over the process, enabling settings like hidden execution and shell execution.
ProcessStartInfo startInfo = new ProcessStartInfo(); startInfo.CreateNoWindow = true; // Run without a visible window startInfo.UseShellExecute = false; // Required for argument passing startInfo.FileName = "dcm2jpg.exe"; startInfo.WindowStyle = ProcessWindowStyle.Hidden; // Hide the window startInfo.Arguments = "-f j -o \"" + ex1 + "\" -z 1.0 -s y " + ex2; // Your arguments try { using (Process exeProcess = Process.Start(startInfo)) { exeProcess.WaitForExit(); // Wait for the process to finish } } catch (Exception ex) { // Handle exceptions appropriately, e.g., log the error Console.WriteLine("Error launching executable: " + ex.Message); }
This example launches dcm2jpg.exe
with specific arguments for image conversion. WaitForExit
ensures the C# code waits for the external process to complete before continuing. Error handling is crucial for robust application behavior.
The Process.Start
method combined with ProcessStartInfo
offers a powerful and flexible way to manage external executable execution and parameter passing within your C# applications. Remember to always handle potential exceptions.
The above is the detailed content of How Can I Launch External Executables and Pass Arguments in C#?. For more information, please follow other related articles on the PHP Chinese website!
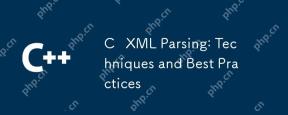
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
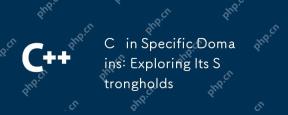
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
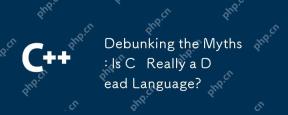
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
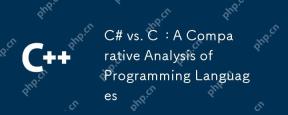
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
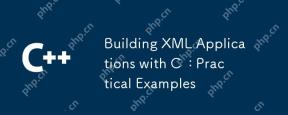
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
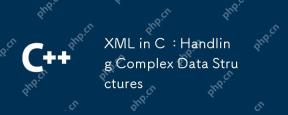
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.
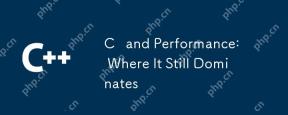
C still dominates performance optimization because its low-level memory management and efficient execution capabilities make it indispensable in game development, financial transaction systems and embedded systems. Specifically, it is manifested as: 1) In game development, C's low-level memory management and efficient execution capabilities make it the preferred language for game engine development; 2) In financial transaction systems, C's performance advantages ensure extremely low latency and high throughput; 3) In embedded systems, C's low-level memory management and efficient execution capabilities make it very popular in resource-constrained environments.
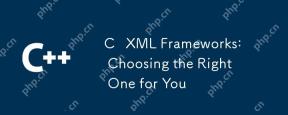
The choice of C XML framework should be based on project requirements. 1) TinyXML is suitable for resource-constrained environments, 2) pugixml is suitable for high-performance requirements, 3) Xerces-C supports complex XMLSchema verification, and performance, ease of use and licenses must be considered when choosing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
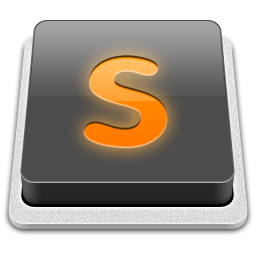
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
