Import SQL table data into C# DataTable
In data processing, reading data from SQL table and converting it to C# DataTable is a common and important task. While inserting a DataTable into a SQL table is well documented, the process of extracting data from a SQL table into a DataTable may be less clear.
We can use the SqlDataAdapter
method of the Fill
class to accomplish this task. This method retrieves data from the specified table or query and populates the DataTable with the results.
Implementation method:
using System; using System.Data; using System.Data.SqlClient; public class DataImporter { // 创建一个DataTable来存储检索到的数据 private DataTable dataTable = new DataTable(); // 从SQL表中提取数据到DataTable的方法 public void ImportData() { // 定义连接字符串 string connString = @"Your connection string here"; // 编写查询语句以从表中选择数据 string query = "SELECT * FROM table"; // 建立数据库连接 using (SqlConnection conn = new SqlConnection(connString)) { // 创建命令对象并执行查询 using (SqlCommand cmd = new SqlCommand(query, conn)) { conn.Open(); // 创建数据适配器并使用查询结果填充DataTable using (SqlDataAdapter da = new SqlDataAdapter(cmd)) { da.Fill(dataTable); } } } } }
After calling the ImportData
method, dataTable
will be populated with data retrieved from the SQL table. This data can then be accessed and processed in C# code.
This method ensures that the data in the SQL table can be efficiently and accurately imported into the C# DataTable for further analysis and operations.
The above is the detailed content of How to Efficiently Import SQL Table Data into a C# DataTable?. For more information, please follow other related articles on the PHP Chinese website!
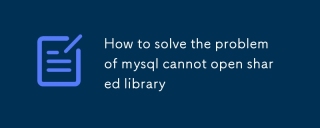
This article addresses MySQL's "unable to open shared library" error. The issue stems from MySQL's inability to locate necessary shared libraries (.so/.dll files). Solutions involve verifying library installation via the system's package m
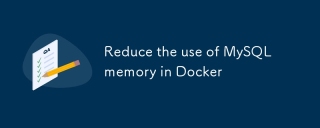
This article explores optimizing MySQL memory usage in Docker. It discusses monitoring techniques (Docker stats, Performance Schema, external tools) and configuration strategies. These include Docker memory limits, swapping, and cgroups, alongside
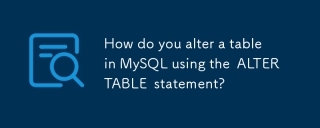
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
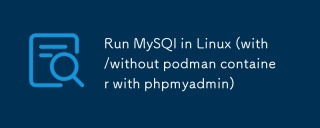
This article compares installing MySQL on Linux directly versus using Podman containers, with/without phpMyAdmin. It details installation steps for each method, emphasizing Podman's advantages in isolation, portability, and reproducibility, but also
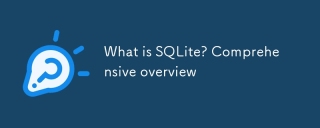
This article provides a comprehensive overview of SQLite, a self-contained, serverless relational database. It details SQLite's advantages (simplicity, portability, ease of use) and disadvantages (concurrency limitations, scalability challenges). C
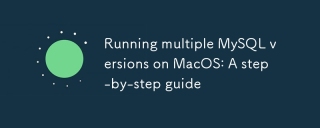
This guide demonstrates installing and managing multiple MySQL versions on macOS using Homebrew. It emphasizes using Homebrew to isolate installations, preventing conflicts. The article details installation, starting/stopping services, and best pra
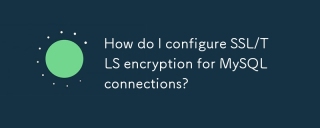
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
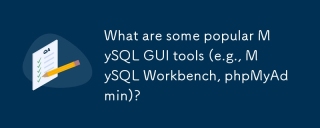
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
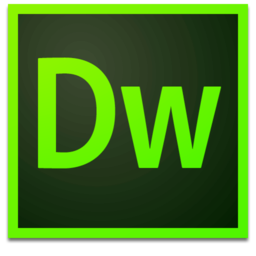
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor
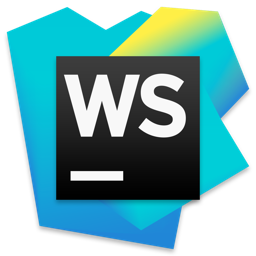
WebStorm Mac version
Useful JavaScript development tools
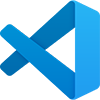
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor
