Achieving True Object Independence with Deep Copies of Lists
The example code shows two lists (List<book> books_1</book>
and List<book> books_2</book>
) containing Book
objects. A simple assignment makes books_2
appear to be a copy of books_1
, but changes to books_2
also affect books_1
because they share references to the same Book
objects.
The Importance of Deep Cloning
To create truly independent copies, we must avoid shared references. This requires creating entirely new Book
objects and populating them with data from the originals – a process called deep copying.
Implementing Deep Copying
Deep copies can be efficiently created using a lambda expression with either the Select
or ConvertAll
methods:
Using Select
:
List<Book> books_2 = books_1.Select(book => new Book(book.title)).ToList();
Using ConvertAll
:
List<Book> books_2 = books_1.ConvertAll(book => new Book(book.title));
Both approaches generate new Book
instances within the lambda expression, copying the title
property from the original Book
objects. The resulting books_2
list contains completely new, independent Book
objects.
Advantages of Deep Copying
Deep copying ensures that modifications to books_2
will not affect books_1
. This is crucial when you need to work with a modified copy without altering the original data. This approach guarantees data integrity and prevents unintended side effects.
The above is the detailed content of How to Create Deep Copies of List for True Object Independence?. For more information, please follow other related articles on the PHP Chinese website!
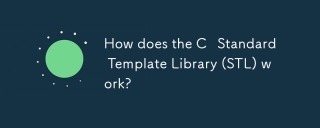
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
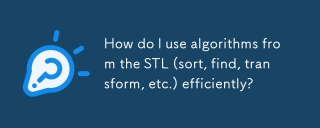
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
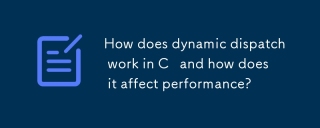
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
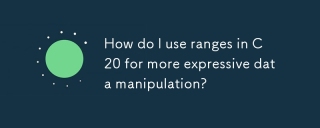
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
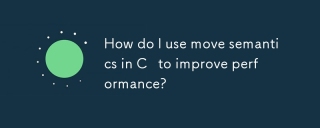
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
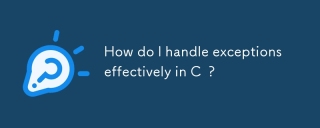
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
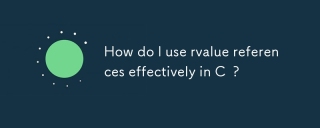
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
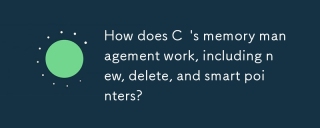
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Zend Studio 13.0.1
Powerful PHP integrated development environment
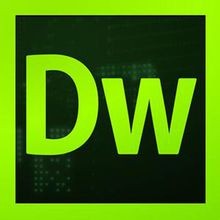
Dreamweaver CS6
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
