JavaScript Simplified: A Beginner's Guide to Mastering Interactive Web Development
Post 4 of 30: Understanding Variables in JavaScript
In this post, we’ll explore what variables are, how to declare them, and how they work in JavaScript.
What is a Variable?
A variable is a way to store a value in JavaScript so we can use it later. Think of a variable like a labeled box where you can keep things and retrieve them when needed.
For example, instead of writing "John" multiple times in your code, you can store it in a variable and use it anywhere.
Declaring Variables in JavaScript
JavaScript provides three ways to declare variables:
- var (older method, not recommended)
- let (modern and recommended for changeable values)
- const (for values that should not change)
1. Using let (Recommended)
let name = "John"; console.log(name);
Output:
John
Here, we:
- Created a variable called name
- Assigned it the value "John"
- Used console.log() to print the value of name
2. Using const (For Constant Values)
const PI = 3.1416; console.log(PI);
Output:
3.1416
- const is used for values that should not change.
- Once assigned, you cannot reassign a new value to PI.
3. Using var (Older Method – Avoid Using)
var age = 25; console.log(age);
Output:
25
- var was commonly used before let and const, but it has scoping issues, so use let or const instead.
Changing Variable Values
With let, you can change a variable’s value, but with const, you cannot.
Example with let:
let city = "New York"; console.log(city); // Output: New York city = "Los Angeles"; // Changing the value console.log(city); // Output: Los Angeles
Example with const (This will cause an error)
const country = "USA"; console.log(country); country = "Canada"; // ❌ This will cause an error console.log(country);
Error: Uncaught TypeError: Assignment to constant variable.
Variable Naming Rules
When naming variables, follow these rules:
✔️ Can contain letters, numbers, $, and _
✔️ Must start with a letter, $, or _ (not a number)
✔️ Case-sensitive (name and Name are different)
✔️ Cannot be a reserved keyword (like let, console, function, etc.)
Examples of Valid Variable Names:
let name = "John"; console.log(name);
Examples of Invalid Variable Names:
John
Practical Exercise: Storing and Changing Values
Try this in your script.js file:
const PI = 3.1416; console.log(PI);
Expected Output:
3.1416
Next Steps
Now that we understand how variables work, the next step is to explore data types in JavaScript—including numbers, strings, booleans, and more!
Stay tuned for the next post! ?
Pro Tip:
? Use let when you expect the value to change.
? Use const when the value should stay the same.
? Avoid var unless you specifically need it.
Follow me on LinkedIn - Ridoy Hasan
Visit my website - Ridoyweb
*Visit my agency website *- webention digital
The above is the detailed content of JavaScript Variables: let vs const vs var. For more information, please follow other related articles on the PHP Chinese website!
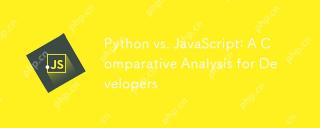
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
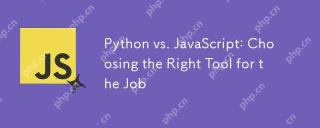
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
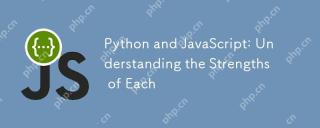
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
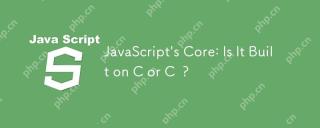
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
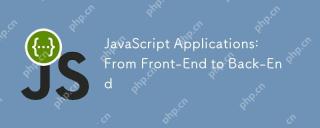
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
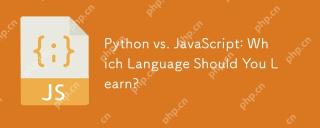
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
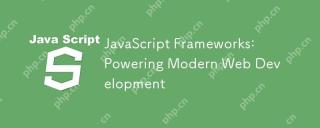
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
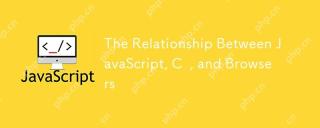
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
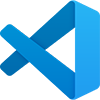
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
