


Making Python CLIs More Maintainable: A Journey with Dynamic Command Loading
This blog post details a recent improvement to our HyperGraph project's command-line interface (CLI): a dynamic command loading system. Initially, adding new CLI commands was a multi-step manual process, violating DRY principles and the Open/Closed Principle.
The Challenge: Manual Command Registration
Adding a new command involved:
- Creating the command's implementation file.
- Updating imports within
__init__.py
. - Adding the command to a static list in the command loader.
This was tedious, prone to errors, and required modifying existing code for each new feature—far from ideal.
Exploring Solutions: Automation vs. Dynamic Loading
Two solutions were considered:
- An automation script to handle file modifications.
- A dynamic loading system leveraging Python's module discovery capabilities.
While an automation script seemed simpler initially, it would only address the symptoms, not the underlying design flaw.
The Solution: Dynamic Command Discovery
The chosen solution was a dynamic loading system that automatically registers commands. The core code is:
async def load_commands(self) -> None: implementations_package = "hypergraph.cli.commands.implementations" for _, name, _ in pkgutil.iter_modules([str(self.commands_path)]): if name.startswith("_"): # Skip private modules continue module = importlib.import_module(f"{implementations_package}.{name}") for item_name, item in inspect.getmembers(module): if (inspect.isclass(item) and issubclass(item, BaseCommand) and item != BaseCommand): command = item(self.system) self.registry.register_command(command)
This approach offers several advantages:
- Eliminates manual command registration.
- Maintains backward compatibility with existing code.
- Simplifies adding new commands to placing a new file in the
implementations
directory. - Leverages standard Python libraries, adhering to the "batteries included" philosophy.
Key Lessons Learned
- Avoid Quick Fixes: While automation offered short-term relief, dynamic loading provides a more sustainable, long-term solution.
-
Preserve Compatibility: Maintaining original
CommandRegistry
methods ensures existing code continues to function. - Robust Error Handling: Comprehensive error handling and logging are vital for debugging in a dynamic system.
A Minor Setback
A minor issue arose with a missing type import (Any
from typing
), highlighting the importance of thorough type hinting in Python.
Future Steps
While the dynamic system is implemented, an automation script remains a possibility as a development tool for generating command file templates. Future plans include:
- Monitoring production performance.
- Gathering developer feedback.
- Implementing further improvements based on real-world use.
Conclusion
This refactoring demonstrates the benefits of reevaluating approaches for more elegant solutions. Though requiring more initial effort than a quick fix, the result is more maintainable, extensible, and Pythonic code. Prioritizing long-term maintainability simplifies future development.
Tags: #Python #Refactoring #CleanCode #CLI #Programming
For detailed technical information, refer to our Codeberg repository.
The above is the detailed content of Making Python CLIs More Maintainable: A Journey with Dynamic Command Loading. For more information, please follow other related articles on the PHP Chinese website!
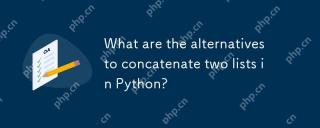
There are many methods to connect two lists in Python: 1. Use operators, which are simple but inefficient in large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use the = operator, which is both efficient and readable; 4. Use itertools.chain function, which is memory efficient but requires additional import; 5. Use list parsing, which is elegant but may be too complex. The selection method should be based on the code context and requirements.
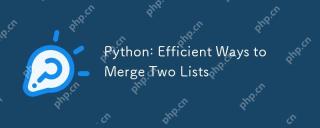
There are many ways to merge Python lists: 1. Use operators, which are simple but not memory efficient for large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use itertools.chain, which is suitable for large data sets; 4. Use * operator, merge small to medium-sized lists in one line of code; 5. Use numpy.concatenate, which is suitable for large data sets and scenarios with high performance requirements; 6. Use append method, which is suitable for small lists but is inefficient. When selecting a method, you need to consider the list size and application scenarios.
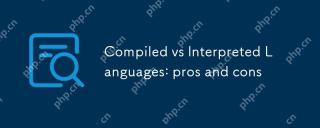
Compiledlanguagesofferspeedandsecurity,whileinterpretedlanguagesprovideeaseofuseandportability.1)CompiledlanguageslikeC arefasterandsecurebuthavelongerdevelopmentcyclesandplatformdependency.2)InterpretedlanguageslikePythonareeasiertouseandmoreportab
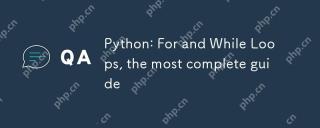
In Python, a for loop is used to traverse iterable objects, and a while loop is used to perform operations repeatedly when the condition is satisfied. 1) For loop example: traverse the list and print the elements. 2) While loop example: guess the number game until you guess it right. Mastering cycle principles and optimization techniques can improve code efficiency and reliability.
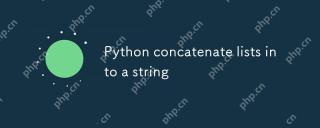
To concatenate a list into a string, using the join() method in Python is the best choice. 1) Use the join() method to concatenate the list elements into a string, such as ''.join(my_list). 2) For a list containing numbers, convert map(str, numbers) into a string before concatenating. 3) You can use generator expressions for complex formatting, such as ','.join(f'({fruit})'forfruitinfruits). 4) When processing mixed data types, use map(str, mixed_list) to ensure that all elements can be converted into strings. 5) For large lists, use ''.join(large_li
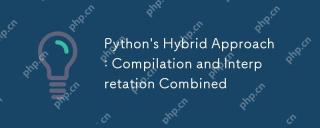
Pythonusesahybridapproach,combiningcompilationtobytecodeandinterpretation.1)Codeiscompiledtoplatform-independentbytecode.2)BytecodeisinterpretedbythePythonVirtualMachine,enhancingefficiencyandportability.
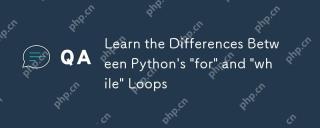
ThekeydifferencesbetweenPython's"for"and"while"loopsare:1)"For"loopsareidealforiteratingoversequencesorknowniterations,while2)"while"loopsarebetterforcontinuinguntilaconditionismetwithoutpredefinediterations.Un
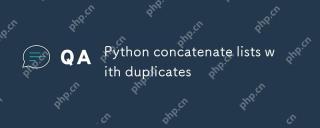
In Python, you can connect lists and manage duplicate elements through a variety of methods: 1) Use operators or extend() to retain all duplicate elements; 2) Convert to sets and then return to lists to remove all duplicate elements, but the original order will be lost; 3) Use loops or list comprehensions to combine sets to remove duplicate elements and maintain the original order.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
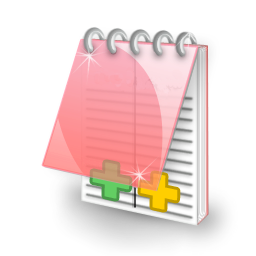
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
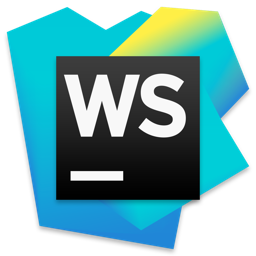
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
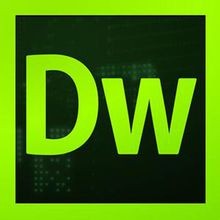
Dreamweaver CS6
Visual web development tools
