Handling Lists of Open Generic Types in C#
Working with open generic types (generics with unspecified type parameters) in C# presents challenges due to limitations in generic polymorphism.
The Challenge:
Consider these classes:
public abstract class Data<T> { } public class StringData : Data<string> { } public class DecimalData : Data<decimal> { }
Attempting to create a list containing instances of these different Data
types directly fails:
List<Data> dataCollection = new List<Data>(); // Compiler error! dataCollection.Add(new DecimalData()); dataCollection.Add(new StringData());
The compiler error arises because Data
is an open generic type, requiring a type argument.
The Solution:
C#'s lack of a diamond operator prevents direct instantiation of lists of open generics. Polymorphism doesn't directly apply to open generic types. The workaround is to use a common base type or interface:
public interface IData { void SomeMethod(); } public abstract class DataBase { public abstract void SomeMethod(); }
Now, you can create a list using the interface or base class:
List<IData> dataList = new List<IData>(); dataList.Add(new StringData()); // StringData must implement IData dataList.Add(new DecimalData()); // DecimalData must implement IData foreach (var item in dataList) item.SomeMethod(); List<DataBase> dataBaseList = new List<DataBase>(); dataBaseList.Add(new StringData()); // StringData must inherit from DataBase dataBaseList.Add(new DecimalData()); // DecimalData must inherit from DataBase foreach (var item in dataBaseList) item.SomeMethod();
This approach allows for a collection of different Data
types, but it sacrifices strong typing compared to a truly generic solution. The SomeMethod()
example highlights the need for a common interface or abstract method to maintain functionality across different types.
Further Reading:
- Generics Open and Closed Constructed Types
- C# Variance Problem: Assigning List
as List - How to do generic polymorphism on open types in C#?
- C# : Is Variance (Covariance / Contravariance) another word for Polymorphism?
- C# generic inheritance and covariance part 2
- still confused about covariance and contravariance & in/out
- Covariance and Contravariance (C#)
The above is the detailed content of How Can I Create a List of Open Generic Types in C#?. For more information, please follow other related articles on the PHP Chinese website!
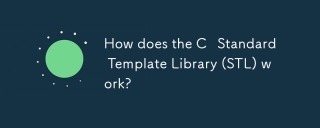
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
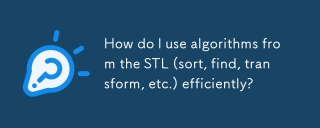
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
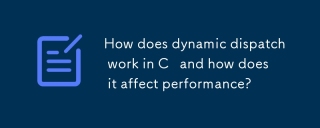
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
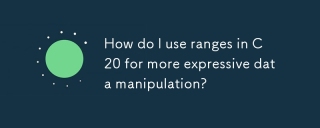
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
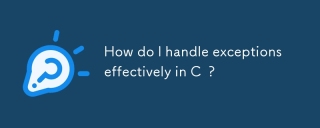
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
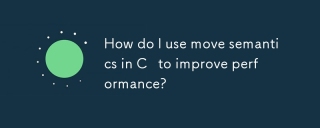
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
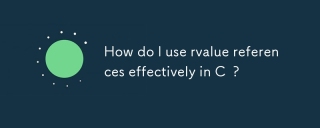
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
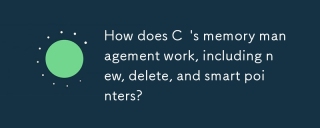
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
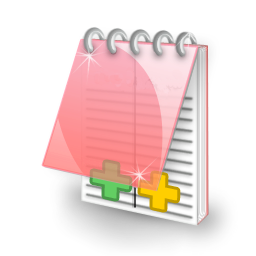
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
