


C# Property Modification: Bypassing Direct Pass-by-Reference
C# doesn't directly support passing properties by reference. This limitation can complicate attempts to modify properties externally, leading to unexpected results.
Understanding the Compile-Time Issue
The following code illustrates the problem:
public void GetString(string inValue, ref string outValue) { // code } public void SetWorkPhone(string inputString) { GetString(inputString, ref this.WorkPhone); // Compile-time error }
This fails because properties aren't reference types; they're methods managing underlying private fields.
Alternative Approaches to Modifying Properties
While direct reference passing is impossible, several techniques achieve similar results:
- Return Value Modification: The simplest approach is to return the modified value:
public string GetString(string inputString) { return string.IsNullOrEmpty(inputString) ? this.WorkPhone : inputString; }
- Delegate-Based Update: Use a delegate to update the property:
public void GetString(string inputString, Action<string> updateWorkPhone) { if (!string.IsNullOrEmpty(inputString)) { updateWorkPhone(inputString); } }
- LINQ Expression Approach: A more advanced method uses LINQ expressions:
public void GetString<T>(string inputString, T target, Expression<Func<T, string>> outExpr) { if (!string.IsNullOrEmpty(inputString)) { var prop = (outExpr.Body as MemberExpression).Member as PropertyInfo; prop.SetValue(target, inputString); } }
- Reflection-Based Modification: Reflection offers dynamic property access and modification:
public void GetString(string inputString, object target, string propertyName) { if (!string.IsNullOrEmpty(inputString)) { var prop = target.GetType().GetProperty(propertyName); prop.SetValue(target, inputString); } }
These methods effectively circumvent the direct pass-by-reference limitation, providing controlled and safe ways to modify C# properties indirectly.
The above is the detailed content of How Can I Effectively Modify C# Properties When Direct Pass-by-Reference Isn't Supported?. For more information, please follow other related articles on the PHP Chinese website!
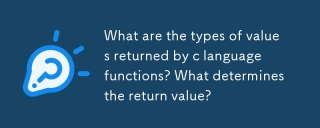
This article details C function return types, encompassing basic (int, float, char, etc.), derived (arrays, pointers, structs), and void types. The compiler determines the return type via the function declaration and the return statement, enforcing

Gulc is a high-performance C library prioritizing minimal overhead, aggressive inlining, and compiler optimization. Ideal for performance-critical applications like high-frequency trading and embedded systems, its design emphasizes simplicity, modul
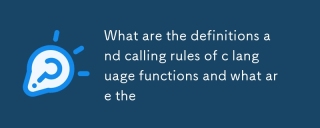
This article explains C function declaration vs. definition, argument passing (by value and by pointer), return values, and common pitfalls like memory leaks and type mismatches. It emphasizes the importance of declarations for modularity and provi

This article details C functions for string case conversion. It explains using toupper() and tolower() from ctype.h, iterating through strings, and handling null terminators. Common pitfalls like forgetting ctype.h and modifying string literals are
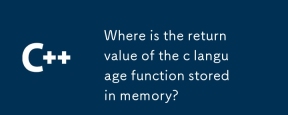
This article examines C function return value storage. Small return values are typically stored in registers for speed; larger values may use pointers to memory (stack or heap), impacting lifetime and requiring manual memory management. Directly acc
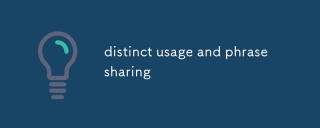
This article analyzes the multifaceted uses of the adjective "distinct," exploring its grammatical functions, common phrases (e.g., "distinct from," "distinctly different"), and nuanced application in formal vs. informal
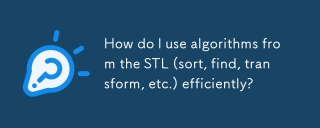
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
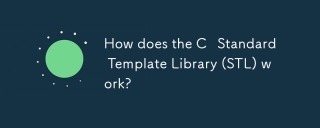
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
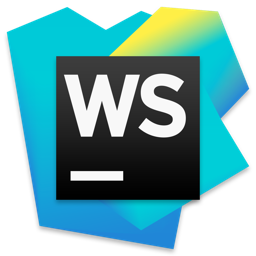
WebStorm Mac version
Useful JavaScript development tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
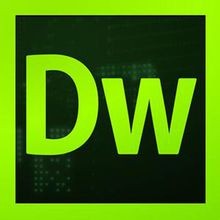
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
