


Handling null values in LINQ queries: solving "Cannot convert to Int32" error
When you encounter the "Cannot convert to value type 'Int32' because the materialized value is null" error in a LINQ query, it indicates a mismatch between the expected non-null type and the possible presence of null values in the query results.
Original query:
var creditsSum = (from u in context.User join ch in context.CreditHistory on u.ID equals ch.UserID where u.ID == userID select ch.Amount).Sum();
Question:
This query retrieves the sum of ch.Amount for users with a specific userID. However, if there is no record for that user in the CreditHistory table, the query will try to convert null values in the database results to Int32, causing an error.
Solution:
To accommodate null values, solutions include introducing nullable types and handling null cases appropriately.
Use nullable types:
First, convert the expression in the select clause to a nullable type, such as int?. This will tell the compiler that the property may contain a null value:
var creditsSum = (from u in context.User join ch in context.CreditHistory on u.ID equals ch.UserID where u.ID == userID select (int?)ch.Amount).Sum();
Use the null coalescing operator (??):
Next, use the ?? operator to handle the null case. It checks if the expression is empty and returns the default value (in this case 0):
var creditsSum = (from u in context.User join ch in context.CreditHistory on u.ID equals ch.UserID where u.ID == userID select (int?)ch.Amount).Sum() ?? 0;
By incorporating these modifications, queries can handle null values gracefully and return appropriate results, preventing conversion errors.
The above is the detailed content of How to Resolve 'Cast to Int32 Failed' Errors in LINQ Queries with Null Values?. For more information, please follow other related articles on the PHP Chinese website!
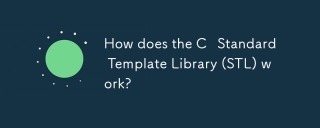
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
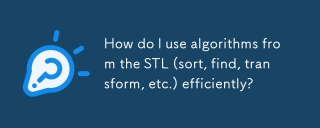
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
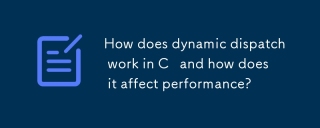
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
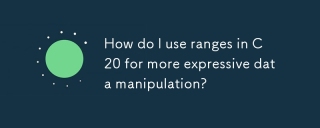
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
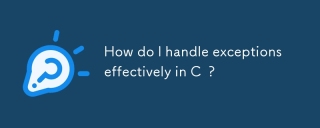
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
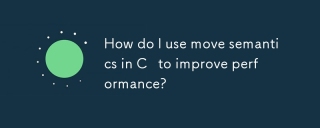
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
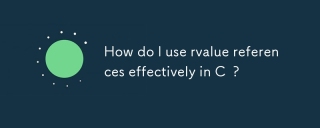
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
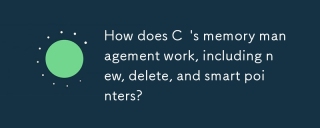
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
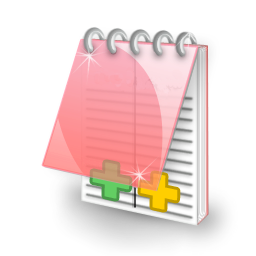
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version
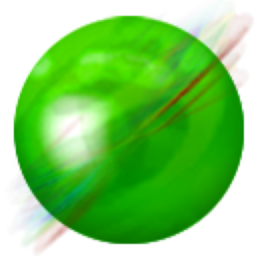
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 English version
Recommended: Win version, supports code prompts!
