


Using JavaScriptSerializer
for JSON Deserialization: Field Name Mapping Challenges
Problem: Directly deserializing JSON data into a C# object using JavaScriptSerializer
often presents challenges when JSON field names differ from C# property names. The XmlElement
attribute doesn't solve this mapping issue.
Scenario:
Let's say you have this JSON:
{"user_id":1234, "detail_level":"low"}
And this corresponding C# class:
public class DataObject { public int UserId { get; set; } public DetailLevel DetailLevel { get; set; } public enum DetailLevel { Low, /* ... other values */ } }
JavaScriptSerializer
will fail to correctly map the fields because of the name mismatch.
Solution: Leveraging DataContractJsonSerializer
The recommended approach is to use DataContractJsonSerializer
instead. This serializer allows explicit mapping through the DataMember
attribute.
Modified C# Class:
using System.Runtime.Serialization; [DataContract] public class DataObject { [DataMember(Name = "user_id")] public int UserId { get; set; } [DataMember(Name = "detail_level")] public string DetailLevel { get; set; } }
Note: The DetailLevel
property is now a string
to avoid potential deserialization problems with enums.
Example Usage:
using System.IO; using System.Runtime.Serialization.Json; using System.Text; // ... within a test method ... [TestMethod] public void DataContractJsonSerializerTest() { string jsonData = "{\"user_id\":1234, \"detail_level\":\"low\"}"; DataContractJsonSerializer serializer = new DataContractJsonSerializer(typeof(DataObject)); MemoryStream ms = new MemoryStream(Encoding.UTF8.GetBytes(jsonData)); // UTF8 encoding is crucial DataObject dataObject = (DataObject)serializer.ReadObject(ms); Assert.IsNotNull(dataObject); Assert.AreEqual(1234, dataObject.UserId); Assert.AreEqual("low", dataObject.DetailLevel); }
This code demonstrates how DataContractJsonSerializer
correctly maps the JSON fields ("user_id", "detail_level") to the corresponding C# properties (UserId
, DetailLevel
) despite the name differences. Remember to handle potential exceptions during deserialization in a production environment.
The above is the detailed content of How Can I Map JSON Field Names to Different C# Object Field Names Using JavaScriptSerializer?. For more information, please follow other related articles on the PHP Chinese website!
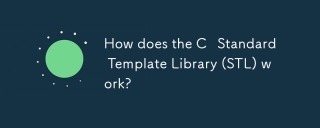
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
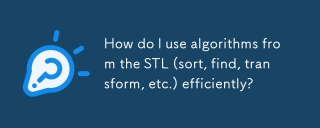
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
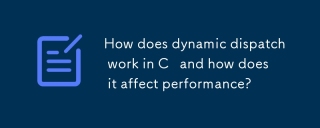
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
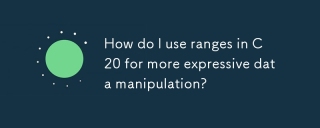
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
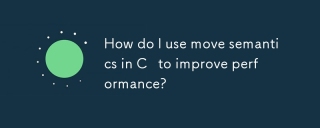
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
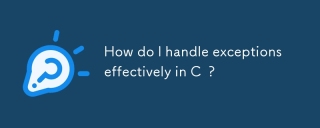
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
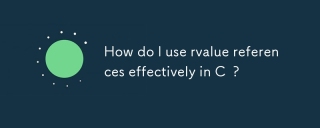
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
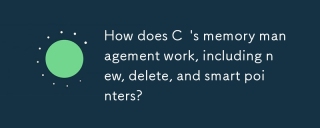
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
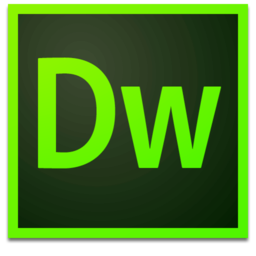
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor
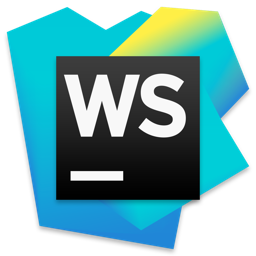
WebStorm Mac version
Useful JavaScript development tools
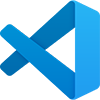
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor
