


Handling Discrepancies Between JSON and .NET Property Names with JavaScriptSerializer
The JavaScriptSerializer
in .NET sometimes requires adjustments when mapping JSON field names to your .NET object properties. For example, a JSON field like "user_id" might need to be mapped to a .NET property named "UserId". Directly customizing field names with JavaScriptSerializer
using annotations isn't feasible.
A Superior Solution: DataContractJsonSerializer
For flexible field name mapping, DataContractJsonSerializer
offers a more robust solution. It leverages the [DataMember]
attribute to explicitly define the mapping:
[DataContract] public class DataObject { [DataMember(Name = "user_id")] public int UserId { get; set; } [DataMember(Name = "detail_level")] public string DetailLevel { get; set; } }
This code ensures that the JSON "user_id" field correctly populates the UserId
property in your .NET object.
Testing the DataContractJsonSerializer
Here's a sample unit test demonstrating the functionality:
using System.Runtime.Serialization.Json; using System.Text; using System.IO; using Microsoft.VisualStudio.TestTools.UnitTesting; [TestClass] public class DataContractJsonSerializerTest { [TestMethod] public void DataObjectSerializationTest() { DataContractJsonSerializer serializer = new DataContractJsonSerializer(typeof(DataObject)); string jsonData = "{\"user_id\":1234,\"detail_level\":\"low\"}"; //Example JSON using (MemoryStream ms = new MemoryStream(Encoding.UTF8.GetBytes(jsonData))) { DataObject dataObject = (DataObject)serializer.ReadObject(ms); Assert.IsNotNull(dataObject); Assert.AreEqual("low", dataObject.DetailLevel); Assert.AreEqual(1234, dataObject.UserId); } } }
Important Note on Enums:
When dealing with enums in your server's JSON response, convert them to strings before deserialization with DataContractJsonSerializer
to prevent potential parsing errors. Direct enum mapping can be problematic.
The above is the detailed content of How Can I Map JSON Field Names to Different .NET Property Names Using JavaScriptSerializer?. For more information, please follow other related articles on the PHP Chinese website!

Gulc is a high-performance C library prioritizing minimal overhead, aggressive inlining, and compiler optimization. Ideal for performance-critical applications like high-frequency trading and embedded systems, its design emphasizes simplicity, modul
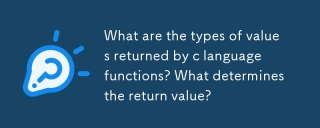
This article details C function return types, encompassing basic (int, float, char, etc.), derived (arrays, pointers, structs), and void types. The compiler determines the return type via the function declaration and the return statement, enforcing
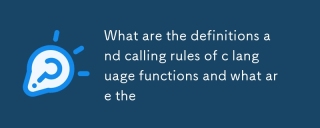
This article explains C function declaration vs. definition, argument passing (by value and by pointer), return values, and common pitfalls like memory leaks and type mismatches. It emphasizes the importance of declarations for modularity and provi

This article details C functions for string case conversion. It explains using toupper() and tolower() from ctype.h, iterating through strings, and handling null terminators. Common pitfalls like forgetting ctype.h and modifying string literals are
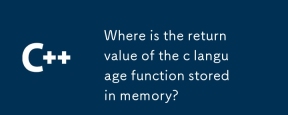
This article examines C function return value storage. Small return values are typically stored in registers for speed; larger values may use pointers to memory (stack or heap), impacting lifetime and requiring manual memory management. Directly acc
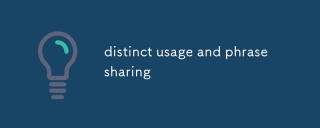
This article analyzes the multifaceted uses of the adjective "distinct," exploring its grammatical functions, common phrases (e.g., "distinct from," "distinctly different"), and nuanced application in formal vs. informal
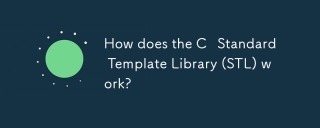
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
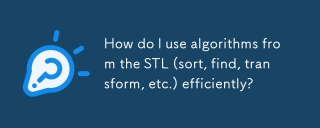
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
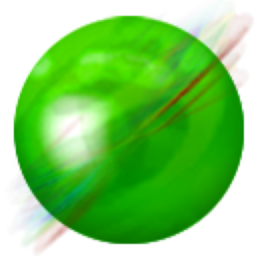
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor
