The role of delegation in C#
In C#, a delegate acts as a reference to a method. While objects are easy to pass as parameters, methods present a challenge. However, in some cases, methods need to be sent as parameters, and this is where delegates come into play.
When and why to use delegates
Delegation is crucial when:
- Passing methods as arguments: They allow you to pass a method as an argument to another method, allowing for more flexible and dynamic code execution.
- Event handling: Delegates are fundamentally used in event handling, where events are declared and registered.
Advantages of using delegation
- Method calling flexibility: Delegates allow calling specific methods based on predefined conditions or user interaction.
- Loose coupling: Delegates promote loose coupling between objects, allowing modification of functionality and code organization without affecting the overall structure.
- Callback Functions: They help in defining and managing callback functions that are called when a specific event or condition is met.
Alternatives to Delegation
In rare cases, there may be alternatives to using delegates. However, they may not provide the same level of flexibility and event handling capabilities.
Necessary use cases for delegation
Delegates become essential when you need to filter a list of objects based on specific criteria, as shown in the following code snippet:
// 创建一个委托来表示过滤器 public delegate bool FilterDelegate(Person p); // 使用委托根据年龄筛选人员 static void DisplayPeople(string title, List<Person> people, FilterDelegate filter) { ... } // 为不同的条件定义过滤器方法 static bool IsChild(Person p) { return p.Age < 18; } static bool IsSenior(Person p) { return p.Age >= 65; }
By using delegates, you can easily filter lists based on different criteria without modifying the main filtering logic.
The above is the detailed content of When and Why Should I Use Delegates in C#?. For more information, please follow other related articles on the PHP Chinese website!
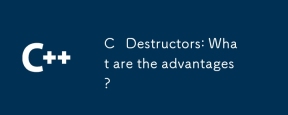
C destructorsprovideseveralkeyadvantages:1)Theymanageresourcesautomatically,preventingleaks;2)Theyenhanceexceptionsafetybyensuringresourcerelease;3)TheyenableRAIIforsaferesourcehandling;4)Virtualdestructorssupportpolymorphiccleanup;5)Theyimprovecode
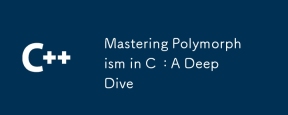
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
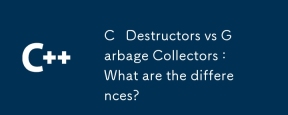
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
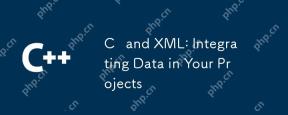
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
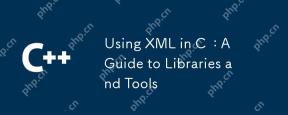
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
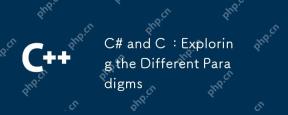
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
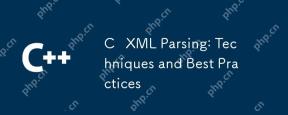
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
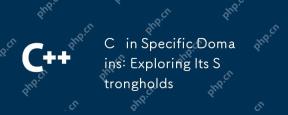
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
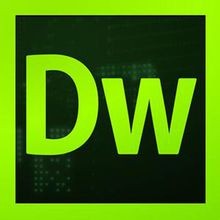
Dreamweaver CS6
Visual web development tools
