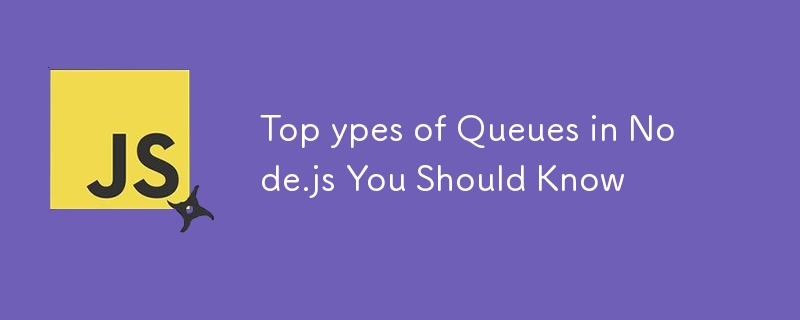
Introduction
Node.js is widely celebrated for its non-blocking, asynchronous architecture, making it an ideal choice for scalable and performant web applications. One of the key reasons behind this capability is its event-driven model and efficient handling of tasks via its event loop. Understanding the asynchronicity of Node.js requires delving into the queues that power this system. Interestingly, Node.js employs six different queues for task management, compared to the two primary queues found in browsers. Let’s explore these in detail.
The Six Queues in Node.js
Node.js has a sophisticated mechanism to handle tasks using six queues:
-
Timer Queue
-
Purpose: Handles tasks scheduled with setTimeout and setInterval.
-
Example:
setTimeout(() => {
console.log('Timer task executed');
}, 1000);
- Tasks in the Timer Queue are executed after the specified delay, but not before the current phase of the event loop is complete.
-
I/O Queue (Callback Queue)
-
Purpose: Processes I/O-related tasks, such as reading files or handling network requests.
-
Example:
const fs = require('fs');
fs.readFile('file.txt', 'utf8', (err, data) => {
if (err) throw err;
console.log(data);
});
- The I/O Queue ensures callbacks are executed once the I/O operation completes.
-
Check Queue
-
Purpose: Executes tasks scheduled using setImmediate().
-
Example:
setImmediate(() => {
console.log('Check Queue task executed');
});
-
Note: The Check Queue has one of the lowest priorities in the event loop. Tasks in this queue are processed after the I/O phase.
-
Microtask Queue
-
Purpose: Executes high-priority tasks, primarily related to promises and other microtasks.
-
Subcategories:
-
a. process.nextTick Queue:
- Handles tasks scheduled with process.nextTick().
- Tasks in this queue are given the highest priority and are executed before any other microtasks.
-
b. Separate Queue for Other Promises:
- Handles tasks related to resolved promises.
process.nextTick(() => {
console.log('process.nextTick task executed');
});
Promise.resolve().then(() => {
console.log('Promise resolved task executed');
});
- The Microtask Queue always runs to completion before moving on to the next phase of the event loop.
-
Close Queue
-
Purpose: Handles tasks related to closing operations, such as socket.on('close') events.
-
Example:
setTimeout(() => {
console.log('Timer task executed');
}, 1000);
- Tasks in the Close Queue are executed when a resource is explicitly closed.
How the Event Loop Prioritizes Queues
The event loop in Node.js follows a specific order of phases for executing tasks. Here is the priority sequence:
-
Microtask Queue (process.nextTick): Tasks in this queue are always executed first.
-
Microtask Queue (Promises): Once process.nextTick tasks are complete, tasks in the Promises queue are executed.
-
Timer Queue: Tasks scheduled with setTimeout or setInterval are processed in this phase.
-
I/O Queue: Handles completed I/O operations.
-
Check Queue: Executes tasks from setImmediate.
-
Close Queue: Processes close callbacks for resources.
Comparison with Browsers
In contrast, browsers have a simpler event loop model with only two primary queues:
-
Macro Task Queue: Handles tasks like setTimeout, setInterval, and DOM events.
-
Microtask Queue: Similar to Node.js, this queue handles tasks like resolved Promises and MutationObserver callbacks.
Node.js’s additional queues enable it to handle a wider variety of tasks, making it more suitable for server-side applications.
Key Insights
Reference:-
- https://frontendmasters.com/courses/servers-node-js/
The above is the detailed content of Top ypes of Queues in Node.js You Should Know. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn