


IEnumerable and IEnumerator in C#: How Do They Enable foreach Loops and Custom Iterators?
In-depth understanding of IEnumerable and IEnumerator: the core mechanism of .NET iterators
In .NET programming, implementing the IEnumerable interface is the key to enabling foreach loops. Although the foreach syntax is concise and easy to use, its underlying dependence is on the IEnumerable and IEnumerator interfaces.
Application scenarios of IEnumerable and IEnumerator
IEnumerable is not used to "replace" foreach; on the contrary, implementing IEnumerable is a prerequisite for using foreach. When you write a foreach loop, the compiler actually converts it into a series of calls to the IEnumerator's MoveNext and Current methods.
The difference between IEnumerable and IEnumerator
- IEnumerable: defines an interface with the GetEnumerator method, which returns an IEnumerator.
- IEnumerator: defines the interface of MoveNext and Current methods. MoveNext moves the iterator to the next item in the sequence, while Current returns the current item.
Why do we need IEnumerable and IEnumerator?
- Custom Iterator: By implementing IEnumerable on your custom classes, you can use a foreach loop to iterate over those classes.
- Understanding the iterator mechanism: IEnumerator provides a low-level mechanism for traversing a collection, allowing you to control the traversal process.
-
Generic Collections: The C# compiler uses IEnumerable and IEnumerator to create generic collection classes, such as List
and Dictionary . - Asynchronous Programming: IEnumerator can be used in conjunction with the asynchronous programming model to traverse asynchronous sequences.
implements IEnumerable
To make a class iterable, you need to implement the IEnumerable interface, which requires implementing the GetEnumerator method that returns an IEnumerator.
Example
Consider the following custom object class that implements the IEnumerable interface:
public class CustomCollection : IEnumerable<int> { private int[] data; public IEnumerator<int> GetEnumerator() { return new CustomEnumerator(data); } IEnumerator IEnumerable.GetEnumerator() { return this.GetEnumerator(); } private class CustomEnumerator : IEnumerator<int> { // MoveNext 和 Current 方法用于遍历 data } }
By implementing IEnumerable and providing a custom enumerator, you can now use a foreach loop to iterate over instances of a CustomCollection.
The above is the detailed content of IEnumerable and IEnumerator in C#: How Do They Enable foreach Loops and Custom Iterators?. For more information, please follow other related articles on the PHP Chinese website!
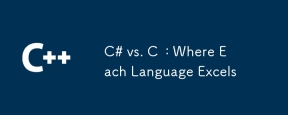
C# is suitable for projects that require high development efficiency and cross-platform support, while C is suitable for applications that require high performance and underlying control. 1) C# simplifies development, provides garbage collection and rich class libraries, suitable for enterprise-level applications. 2)C allows direct memory operation, suitable for game development and high-performance computing.
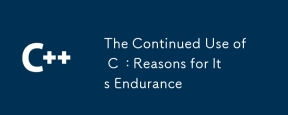
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
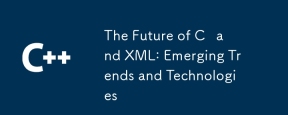
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
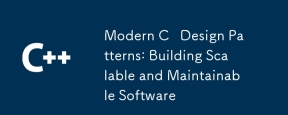
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
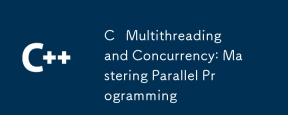
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
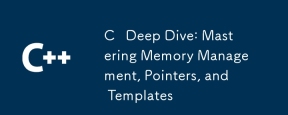
C's memory management, pointers and templates are core features. 1. Memory management manually allocates and releases memory through new and deletes, and pay attention to the difference between heap and stack. 2. Pointers allow direct operation of memory addresses, and use them with caution. Smart pointers can simplify management. 3. Template implements generic programming, improves code reusability and flexibility, and needs to understand type derivation and specialization.
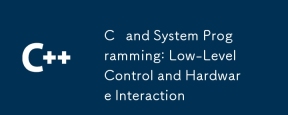
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.
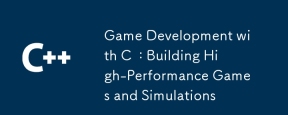
C is suitable for building high-performance gaming and simulation systems because it provides close to hardware control and efficient performance. 1) Memory management: Manual control reduces fragmentation and improves performance. 2) Compilation-time optimization: Inline functions and loop expansion improve running speed. 3) Low-level operations: Direct access to hardware, optimize graphics and physical computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
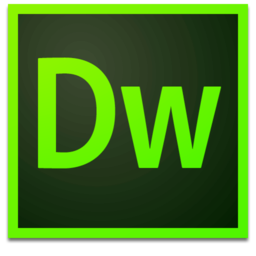
Dreamweaver Mac version
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
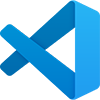
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool