


C# generic types and instantiation of parameterized constructors
Suppose you have a generic method that accepts a generic type, and you want to instantiate an instance of that type with specific constructor parameters. However, the type's constructor requires a parameter, and you don't know if you can do that.
The following code snippet demonstrates an attempt to create a fruit object in a generic method:
public void AddFruit<T>() where T : BaseFruit { BaseFruit fruit = new T(weight); /*new Apple(150);*/ fruit.Enlist(fruitManager); }
This approach may fail because BaseFruit
does not have a parameterless constructor. It takes an integer parameter to specify the weight of the fruit.
To solve this problem, C# provides the Activator
class. You can create an instance of a type using the Activator.CreateInstance
method and pass an array of objects as a constructor argument:
return (T)Activator.CreateInstance(typeof(T), new object[] { weight });
Note that using the T
constraint on new()
only ensures that the compiler checks for public parameterless constructors at compile time. The actual code used to create the type is handled by the Activator
class.
While this approach allows you to instantiate a type with a parameterized constructor, you must ensure that the specific constructor is present in the type definition. Relying on this approach may indicate potential code smells and suggest that you should explore other design options.
The above is the detailed content of How Can I Instantiate Generic Types with Parameterized Constructors in C#?. For more information, please follow other related articles on the PHP Chinese website!
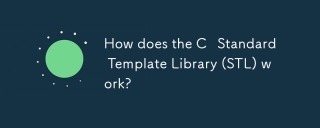
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
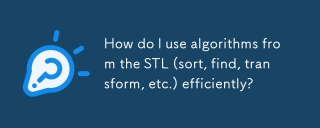
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
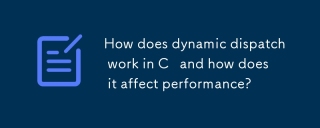
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
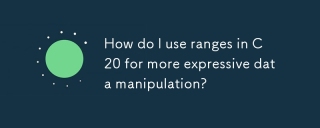
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
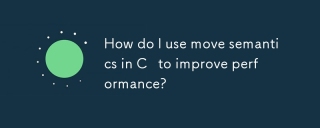
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
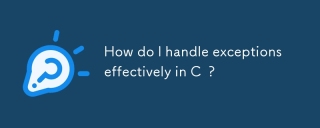
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
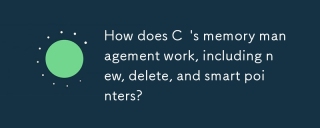
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
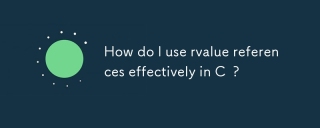
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
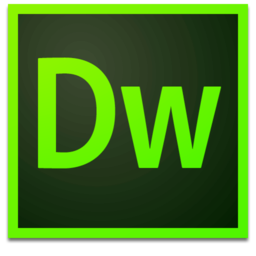
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
