LINQ – Tips for left joins, grouping and precise counting
Converting complex SQL queries to LINQ to SQL can sometimes be tricky. A typical example is to perform a left join, group by column and count the number of records that meet a certain condition.
Consider the following SQL query:
SELECT p.ParentId, COUNT(c.ChildId) FROM ParentTable p LEFT OUTER JOIN ChildTable c ON p.ParentId = c.ChildParentId GROUP BY p.ParentId
This query retrieves the ParentId and matching ChildId count from two tables, ParentTable and ChildTable.
To convert this to LINQ to SQL, first do a left join:
from p in context.ParentTable join c in context.ChildTable on p.ParentId equals c.ChildParentId into j1
However, the COUNT(c.ChildId)
part needs to be handled carefully. Without special considerations, LINQ-generated SQL will always return COUNT(*)
, which may not provide the expected results.
To accurately count only matching ChildId values, add the following after the into
clause:
from j2 in j1.DefaultIfEmpty()
This ensures that if no matching child record is found, a null value is assigned to j2.
Finally, perform grouping and counting:
group j2 by p.ParentId into grouped select new { ParentId = grouped.Key, Count = grouped.Count(t => t.ChildId != null) }
This code groups by ParentId and only counts records whose ChildId is not empty. The result is an object with ParentId and Count properties, matching the format of the original SQL query. This ensures that we only count the cases where sub-records exist, avoiding null values caused by left joins that affect the accuracy of the counting results.
The above is the detailed content of How to Accurately Count Child Records in a LINQ Left Join and Group By?. For more information, please follow other related articles on the PHP Chinese website!
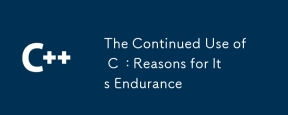
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
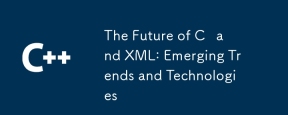
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
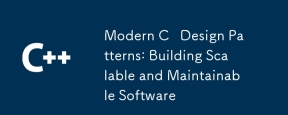
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
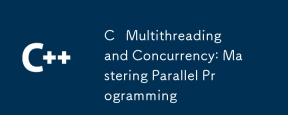
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
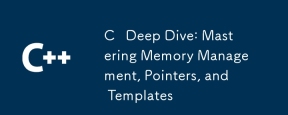
C's memory management, pointers and templates are core features. 1. Memory management manually allocates and releases memory through new and deletes, and pay attention to the difference between heap and stack. 2. Pointers allow direct operation of memory addresses, and use them with caution. Smart pointers can simplify management. 3. Template implements generic programming, improves code reusability and flexibility, and needs to understand type derivation and specialization.
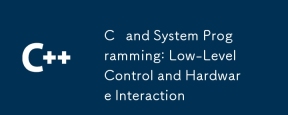
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.
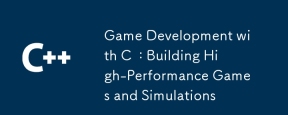
C is suitable for building high-performance gaming and simulation systems because it provides close to hardware control and efficient performance. 1) Memory management: Manual control reduces fragmentation and improves performance. 2) Compilation-time optimization: Inline functions and loop expansion improve running speed. 3) Low-level operations: Direct access to hardware, optimize graphics and physical computing.
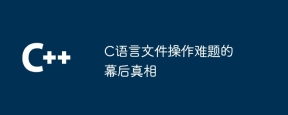
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
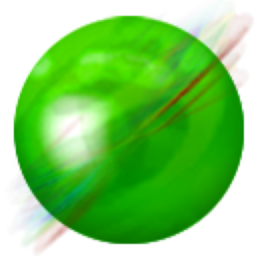
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.