The reason why C# asynchronous method hangs when accessing Task results
When using C#'s async
and await
keywords for asynchronous programming, certain constructs can cause unexpected behavior and potential deadlocks.
Consider the following scenario: A multi-tier application uses an extended database utility method ExecuteAsync
that asynchronously executes a SQL query and returns the results. The middle layer method GetTotalAsync
calls ExecuteAsync
to retrieve the data and stores the result in the asyncTask
variable. Finally, UI operations attempt to access the results synchronously using asyncTask.Result
. However, the application hangs indefinitely.
Cause of deadlock
The problem arises from using GetTotalAsync
in the await
method. By default, continuations of async methods are dispatched on the same SynchronizationContext
that started the method. In this case, when using await
on the UI thread, the continuation (return result;
) is also scheduled to run on the UI thread.
When asyncTask.Result
is called on the UI thread, it blocks the thread when the Task completes. However, continuations scheduled on the UI thread cannot execute until asyncTask.Result
completes. This creates a deadlock where neither thread can continue execution.
Solution
In order to solve this deadlock, there are several methods:
1. Delete Async keyword:
Eliminate the use of await
and rewrite the ExecuteAsync
and GetTotalAsync
methods as pure asynchronous methods that do not wait:
public static Task<T> ExecuteAsync<T>(this OurDBConn dataSource, Func<OurDBConn, T> function) { // ... (代码保持不变) } public static Task<ResultClass> GetTotalAsync(...) { // ... (代码保持不变) }
2. Use ConfigureAwait:
Use ConfigureAwait(false)
to specify that continuations should not be scheduled on the UI thread:
public static async Task<ResultClass> GetTotalAsync(...) { var resultTask = this.DBConnection.ExecuteAsync<ResultClass>( ds => ds.Execute("select slow running data into result")); return await resultTask.ConfigureAwait(false); }
Note that this approach requires explicit specification of await
on all ConfigureAwait(false)
operations that may lead to deadlock.
3. Use SynchronizationContext:
Create a specific SynchronizationContext
for asynchronous operations and ensure that all await
operations use that context, preventing conflicts with the UI thread.
The above is the detailed content of Why Does My Asynchronous C# Method Hang When Accessing Task Results?. For more information, please follow other related articles on the PHP Chinese website!
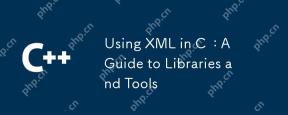
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
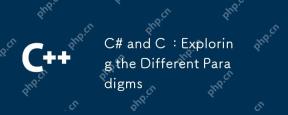
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
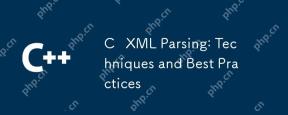
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
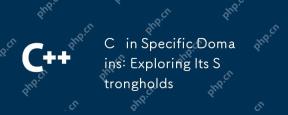
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
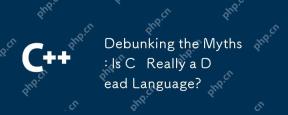
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.
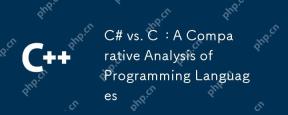
The main differences between C# and C are syntax, memory management and performance: 1) C# syntax is modern, supports lambda and LINQ, and C retains C features and supports templates. 2) C# automatically manages memory, C needs to be managed manually. 3) C performance is better than C#, but C# performance is also being optimized.
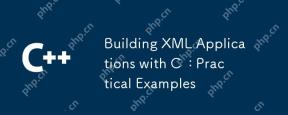
You can use the TinyXML, Pugixml, or libxml2 libraries to process XML data in C. 1) Parse XML files: Use DOM or SAX methods, DOM is suitable for small files, and SAX is suitable for large files. 2) Generate XML file: convert the data structure into XML format and write to the file. Through these steps, XML data can be effectively managed and manipulated.
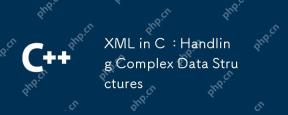
Working with XML data structures in C can use the TinyXML or pugixml library. 1) Use the pugixml library to parse and generate XML files. 2) Handle complex nested XML elements, such as book information. 3) Optimize XML processing code, and it is recommended to use efficient libraries and streaming parsing. Through these steps, XML data can be processed efficiently.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Atom editor mac version download
The most popular open source editor
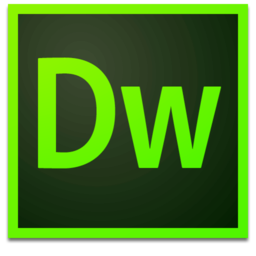
Dreamweaver Mac version
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
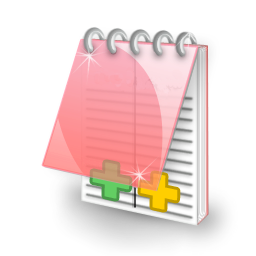
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
