As the web development landscape evolves, the demand for faster, more efficient, and scalable solutions continues to grow. React Server Components (RSC) have emerged as a game-changing feature, designed to address these needs by optimizing how we build and deliver modern web applications. Let’s explore what React Server Components are, why they matter, and how you can start using them.
What Are React Server Components?
React Server Components (RSC) are a new type of React component that runs on the server rather than the client. Unlike traditional React components, which rely on client-side rendering, RSC allows developers to offload logic and rendering to the server, reducing the amount of JavaScript sent to the browser. This approach improves performance and user experience.
Key Features of RSC:
- Server-First Rendering: Components are rendered on the server, reducing the need for hydration.
- Efficient Data Fetching: Fetch data directly on the server without additional client-side API calls.
- Less Client-Side JavaScript: Minimize JavaScript payloads, leading to faster page loads.
- Seamless Integration: Works alongside traditional client components, enabling hybrid rendering.
Benefits of React Server Components
1. Improved Performance
By shifting rendering to the server, RSC reduces the amount of JavaScript that needs to be downloaded and executed in the browser. This results in faster load times and improved performance, especially on low-powered devices.
2. Simplified Data Fetching
With RSC, you can fetch data directly on the server as part of the component’s rendering process. This eliminates the need for complex client-side state management or additional API calls.
3. SEO-Friendly Applications
Server-rendered components ensure that search engines can easily index your content, enhancing the discoverability of your web application.
4. Reduced Bundle Size
Since RSC doesn’t require client-side JavaScript for certain components, it significantly reduces the overall bundle size, leading to faster page loads.
How Do React Server Components Work?
RSC leverages the server’s processing power to handle rendering, enabling a more efficient workflow. Here’s a simplified overview:
- Component Rendering: The server renders React components and sends the result as serialized HTML and JSON to the client.
- Hybrid Components: You can use both server and client components in the same application. For instance, use RSC for static content and client components for interactive elements.
- Streaming: React supports streaming responses, allowing content to progressively load in the browser while rendering continues on the server.
Real-World Example: Building with React Server Components
Let’s walk through a simple implementation of React Server Components.
Setting Up Your Project
To start using RSC, you need a React setup that supports server rendering. Tools like Next.js or frameworks integrating React 18 are ideal.
Example Code
1. Server Component:
// components/ProductList.server.js import fetch from 'node-fetch'; export default async function ProductList() { const res = await fetch('https://api.example.com/products'); const products = await res.json(); return (
-
{products.map((product) => (
- {product.name} - ${product.price} ))}
2. Client Component:
// components/ProductDetail.client.js import { useState } from 'react'; export default function ProductDetail({ product }) { const [details, setDetails] = useState(null); async function fetchDetails() { const res = await fetch(`/api/product/${product.id}`); const data = await res.json(); setDetails(data); } return ( <div> <h2 id="product-name">{product.name}</h2> <button onclick="{fetchDetails}">View Details</button> {details && <p>{details.description}</p>} </div> ); }
3. Combining Components:
// pages/index.js import ProductList from '../components/ProductList.server'; import ProductDetail from '../components/ProductDetail.client'; export default function Home() { return ( <div> <h1 id="Product-Store">Product Store</h1> <productlist></productlist> </div> ); }
Challenges and Considerations
- Server Dependency: RSC relies on server resources, making it less suitable for static hosting environments.
- Learning Curve: Developers need to adapt to a new paradigm of separating client and server components.
- Tooling and Framework Support: Ensure your framework supports RSC for seamless implementation.
The Future of React Server Components
React Server Components represent a significant step forward in web development, bridging the gap between server-side rendering and client-side interactivity. As frameworks like Next.js continue to enhance their RSC support, we can expect even more powerful and scalable web applications in the future.
Conclusion
React Server Components are revolutionizing modern web development by offering a hybrid approach to rendering, reducing client-side JavaScript, and improving performance. While they come with their own set of challenges, their benefits make them an exciting addition to any developer’s toolkit. If you’re building dynamic, scalable applications, RSC is a technology you should explore.
Are you using React Server Components in your projects? Share your thoughts and experiences in the comments below!
The above is the detailed content of React Server Components: Revolutionizing Modern Web Development. For more information, please follow other related articles on the PHP Chinese website!
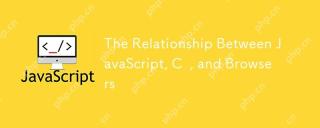
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
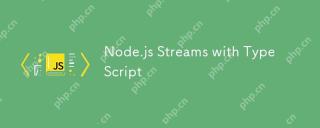
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
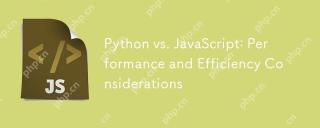
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
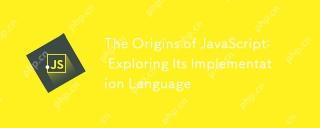
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
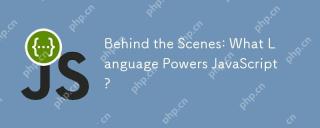
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
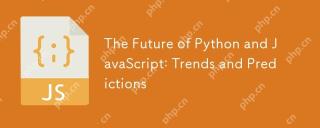
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
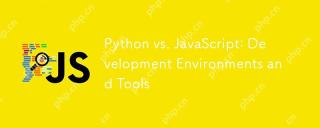
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
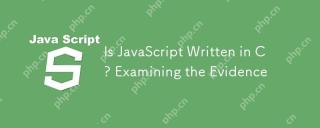
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
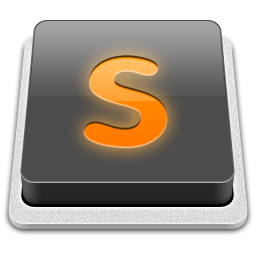
SublimeText3 Mac version
God-level code editing software (SublimeText3)
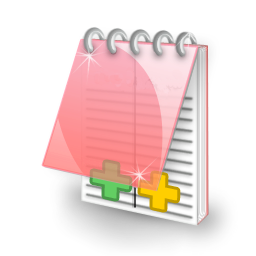
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version

Zend Studio 13.0.1
Powerful PHP integrated development environment
