Dynamic execution of C# code files
Question:
Your WPF C# application comes with a button that needs to execute code stored in a separate text file in the application's runtime directory.
Solution:
To dynamically execute code in a text file, follow these steps:
- Compile code: Use a C# compiler (such as CSharpCodeProvider) to compile the code in the text file into an assembly.
- Create an instance of a compiled assembly: Use the CreateInstance() method of the System.Reflection.Assembly class to create an instance of a compiled assembly.
- Invoking a method: Use the GetMethod() and Invoke() methods of the System.Reflection.Type class to invoke the desired method on the instance.
Here is sample code on how to execute a dynamically compiled class method:
using System; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.IO; using System.Reflection; using System.Net; using Microsoft.CSharp; using System.CodeDom.Compiler; namespace ConsoleApplication2 { class Program { static void Main(string[] args) { string source = @" namespace Foo { public class Bar { public void SayHello() { System.Console.WriteLine(""Hello World""); } } } "; Dictionary<string, string> providerOptions = new Dictionary<string, string> { {"CompilerVersion", "v3.5"} }; CSharpCodeProvider provider = new CSharpCodeProvider(providerOptions); CompilerParameters compilerParams = new CompilerParameters {GenerateInMemory = true, GenerateExecutable = false}; CompilerResults results = provider.CompileAssemblyFromSource(compilerParams, source); if (results.Errors.Count != 0) throw new Exception("编译失败!"); object o = results.CompiledAssembly.CreateInstance("Foo.Bar"); MethodInfo mi = o.GetType().GetMethod("SayHello"); mi.Invoke(o, null); } } }
Following these steps, you can dynamically execute C# code from a code file in a WPF application.
The above is the detailed content of How Can I Dynamically Execute C# Code from a File in a WPF Application?. For more information, please follow other related articles on the PHP Chinese website!
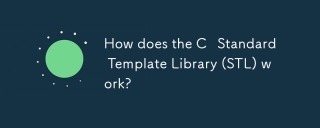
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
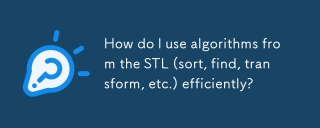
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
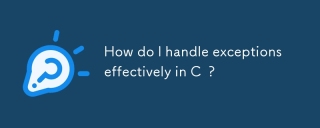
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
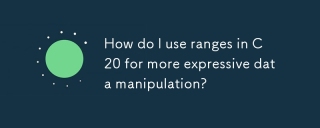
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
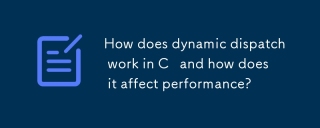
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
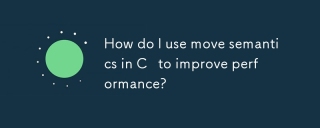
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
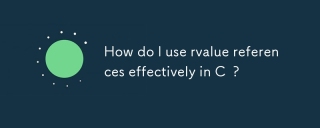
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
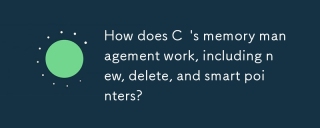
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!
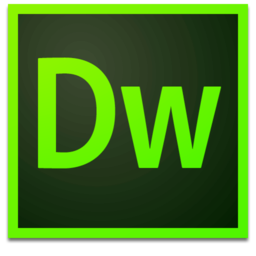
Dreamweaver Mac version
Visual web development tools
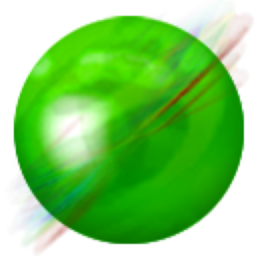
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
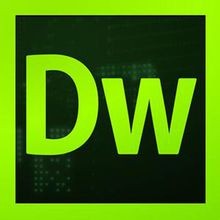
Dreamweaver CS6
Visual web development tools