


Efficiently Finding Maximum Values and Associated Data in Large SQL Tables
Often, database queries require finding the maximum value in a column and retrieving the corresponding data from other columns within the same row. This is especially challenging with very large datasets. Consider a table needing to find the highest version number for each unique ID, along with its associated tag:
Sample Table:
<code>ID | tag | version -----+-----+----- 1 | A | 10 2 | A | 20 3 | B | 99 4 | C | 30 5 | F | 40</code>
Desired Result:
<code>ID | tag | version -----+-----+----- 2 | A | 20 3 | B | 99 4 | C | 30 5 | F | 40</code>
For tables with around 28 million rows, standard methods like nested SELECT
statements or simple GROUP BY
with MAX
can be incredibly slow. A much more efficient solution uses the ROW_NUMBER()
window function:
SELECT s.id, s.tag, s.version FROM ( SELECT t.*, ROW_NUMBER() OVER(PARTITION BY t.id ORDER BY t.version DESC) AS rnk FROM YourTable t ) s WHERE s.rnk = 1;
This query works in two steps:
-
Inner Query: It assigns a unique rank (
rnk
) to each row within eachID
partition (group of rows with the same ID). The ranking is based on theversion
column in descending order, meaning the highest version gets rank 1. -
Outer Query: It filters the results from the inner query, selecting only the rows where
rnk = 1
. This effectively gives us the row with the maximumversion
for eachID
.
This approach avoids nested queries and GROUP BY
operations, making it significantly faster for large datasets. The use of ROW_NUMBER()
provides a clean and efficient way to achieve the desired outcome.
The above is the detailed content of How to Efficiently Retrieve the Maximum Value and Corresponding Data from a Large SQL Database Table?. For more information, please follow other related articles on the PHP Chinese website!
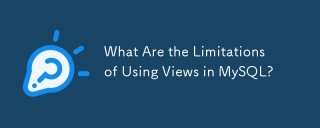
MySQLviewshavelimitations:1)Theydon'tsupportallSQLoperations,restrictingdatamanipulationthroughviewswithjoinsorsubqueries.2)Theycanimpactperformance,especiallywithcomplexqueriesorlargedatasets.3)Viewsdon'tstoredata,potentiallyleadingtooutdatedinforma
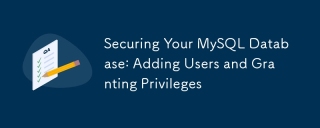
ProperusermanagementinMySQLiscrucialforenhancingsecurityandensuringefficientdatabaseoperation.1)UseCREATEUSERtoaddusers,specifyingconnectionsourcewith@'localhost'or@'%'.2)GrantspecificprivilegeswithGRANT,usingleastprivilegeprincipletominimizerisks.3)
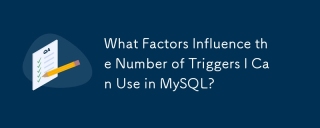
MySQLdoesn'timposeahardlimitontriggers,butpracticalfactorsdeterminetheireffectiveuse:1)Serverconfigurationimpactstriggermanagement;2)Complextriggersincreasesystemload;3)Largertablesslowtriggerperformance;4)Highconcurrencycancausetriggercontention;5)M
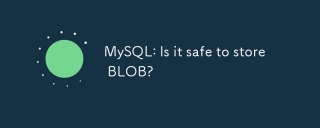
Yes,it'ssafetostoreBLOBdatainMySQL,butconsiderthesefactors:1)StorageSpace:BLOBscanconsumesignificantspace,potentiallyincreasingcostsandslowingperformance.2)Performance:LargerrowsizesduetoBLOBsmayslowdownqueries.3)BackupandRecovery:Theseprocessescanbe
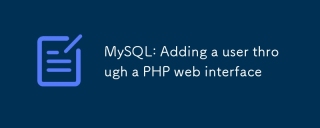
Adding MySQL users through the PHP web interface can use MySQLi extensions. The steps are as follows: 1. Connect to the MySQL database and use the MySQLi extension. 2. Create a user, use the CREATEUSER statement, and use the PASSWORD() function to encrypt the password. 3. Prevent SQL injection and use the mysqli_real_escape_string() function to process user input. 4. Assign permissions to new users and use the GRANT statement.
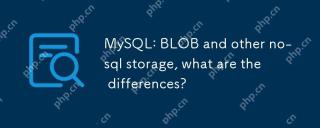
MySQL'sBLOBissuitableforstoringbinarydatawithinarelationaldatabase,whileNoSQLoptionslikeMongoDB,Redis,andCassandraofferflexible,scalablesolutionsforunstructureddata.BLOBissimplerbutcanslowdownperformancewithlargedata;NoSQLprovidesbetterscalabilityand
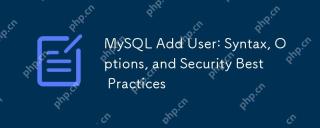
ToaddauserinMySQL,use:CREATEUSER'username'@'host'IDENTIFIEDBY'password';Here'showtodoitsecurely:1)Choosethehostcarefullytocontrolaccess.2)SetresourcelimitswithoptionslikeMAX_QUERIES_PER_HOUR.3)Usestrong,uniquepasswords.4)EnforceSSL/TLSconnectionswith
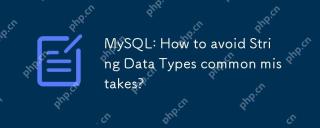
ToavoidcommonmistakeswithstringdatatypesinMySQL,understandstringtypenuances,choosetherighttype,andmanageencodingandcollationsettingseffectively.1)UseCHARforfixed-lengthstrings,VARCHARforvariable-length,andTEXT/BLOBforlargerdata.2)Setcorrectcharacters


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
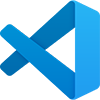
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
