Atomic and Reliable Row Handling with T-SQL
To maintain the integrity of a booking system, it's crucial to ensure that updates and insertions are atomic and reliable. An atomic transaction guarantees that if multiple users attempt to update the same row concurrently, only one update will succeed, preventing race conditions.
Suppose you have a table named "Bookings" with a unique index on the "FlightId" column. Your goal is to create a stored procedure that updates the "TicketsBooked" column for a specific flight ID. If the row corresponding to that flight ID doesn't exist, you need to insert it.
Here's how you can achieve this atomic and reliable operation using T-SQL:
-- BEGIN TRANSACTION BEGIN TRANSACTION; -- Check if the row exists IF EXISTS (SELECT * FROM Bookings WHERE FlightID = @Id) BEGIN -- Update the existing row UPDATE Bookings SET TicketsBooked = TicketsBooked + @TicketsToBook WHERE FlightID = @Id AND TicketsMax <p>This stored procedure uses the BEGIN TRANSACTION and COMMIT TRANSACTION statements to define the transaction boundary. Within the transaction, it first checks if the row with the specified flight ID exists using the IF EXISTS statement. If it doesn't, the INSERT statement inserts a new row.</p><p>If the row exists, the UPDATE statement updates the "TicketsBooked" column only if the updated value doesn't exceed the "TicketsMax" limit. By using a transaction, we ensure that either the update or insertion is successfully committed, or if any error occurs, the transaction is rolled back.</p><p>Finally, the CASE statement checks the @@ERROR global variable to determine the success or failure of the transaction and returns a Boolean value (TRUE or FALSE) accordingly.</p>
The above is the detailed content of How to Guarantee Atomic and Reliable Row Handling in T-SQL?. For more information, please follow other related articles on the PHP Chinese website!
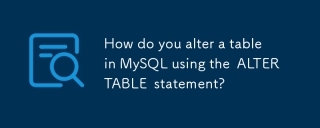
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
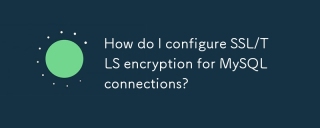
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
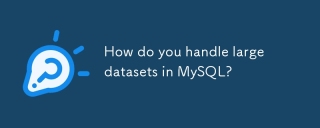
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
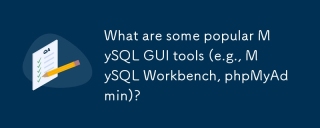
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
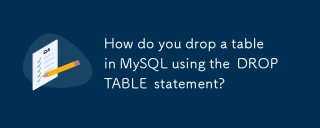
The article discusses dropping tables in MySQL using the DROP TABLE statement, emphasizing precautions and risks. It highlights that the action is irreversible without backups, detailing recovery methods and potential production environment hazards.
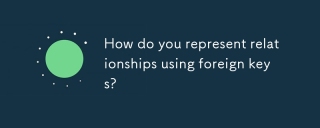
Article discusses using foreign keys to represent relationships in databases, focusing on best practices, data integrity, and common pitfalls to avoid.
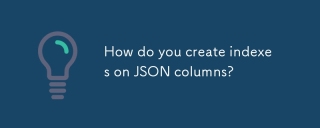
The article discusses creating indexes on JSON columns in various databases like PostgreSQL, MySQL, and MongoDB to enhance query performance. It explains the syntax and benefits of indexing specific JSON paths, and lists supported database systems.
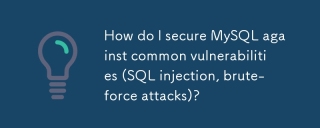
Article discusses securing MySQL against SQL injection and brute-force attacks using prepared statements, input validation, and strong password policies.(159 characters)


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
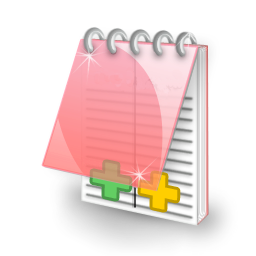
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
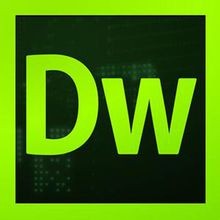
Dreamweaver CS6
Visual web development tools
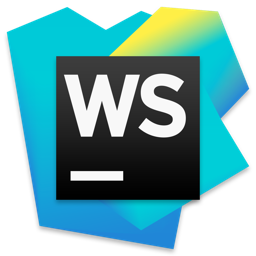
WebStorm Mac version
Useful JavaScript development tools
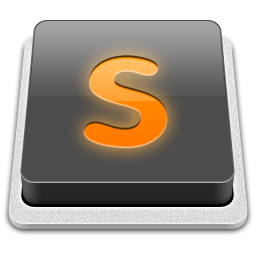
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
