Understanding the Use of "ref" with Reference-Type Variables in C#
While it's clear that the "ref" keyword allows us to pass a reference to value-type variables by value, its significance with reference-types may be less apparent. This article explores the specific use cases of "ref" with reference-type variables.
Consider the following class:
public class Foo { public string Name; public Foo(string name) { Name = name; } }
Passing a Reference-Type Variable by Value vs. Reference:
Without the "ref" keyword, passing a reference-type variable (e.g., "x") to a method still passes a reference, not a copy. This means that the method operates on the original object. For example:
var x = new Foo("1"); void Bar(Foo y) { y.Name = "2"; } Bar(x); // changes the Name property of the original object
Using "ref" to Change the Reference of a Reference-Type Variable:
However, the "ref" keyword with reference-type variables serves a specific purpose: it allows us to change the reference of the variable within the method. For instance:
Foo foo = new Foo("1"); void Bar(ref Foo y) { y = new Foo("2"); // creates a new object and assigns it to y } Bar(ref foo); // changes the reference of 'foo' to point to the new object
In this case, after calling "Bar(ref foo)", "foo" will no longer reference the original object but instead will reference the newly created object with the Name property "2".
Practical Application:
This functionality can be useful in scenarios where we want to return a new object from a method without having to explicitly pass the reference back as an out parameter. For example, we could create a method that finds and returns the first element in a list that meets a certain criteria:
public static T FindFirst<t>(List<t> list, Func<t bool> predicate) where T : class { foreach (T item in list) { if (predicate(item)) return item; } return null; }</t></t></t>
Using the "ref" keyword in this method allows us to avoid having to create an out parameter while still being able to return the found object.
The above is the detailed content of When and Why Use 'ref' with Reference-Type Variables in C#?. For more information, please follow other related articles on the PHP Chinese website!
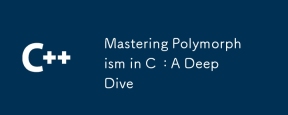
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
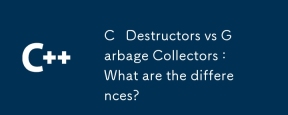
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
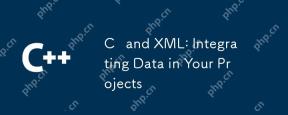
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
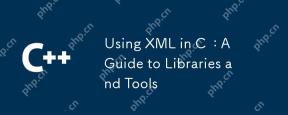
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
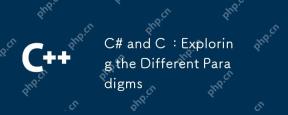
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
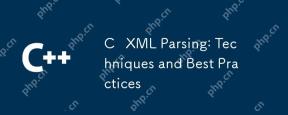
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
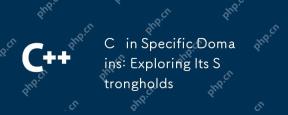
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
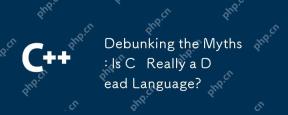
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
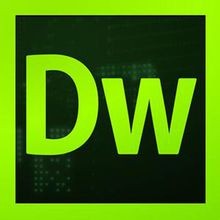
Dreamweaver CS6
Visual web development tools
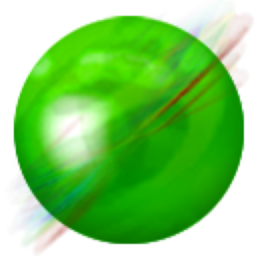
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
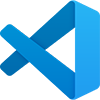
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
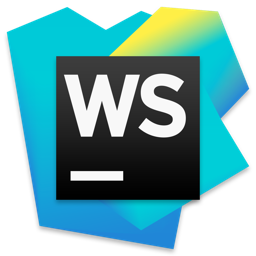
WebStorm Mac version
Useful JavaScript development tools
