React is a powerful and versatile JavaScript library used for building user interfaces. One of its modern features, Suspense, allows components to handle asynchronous data gracefully. However, the concept of "Legacy Promise Throwing Behavior" in React often causes confusion among developers. This blog post aims to break down what this behavior entails, how it fits into React’s rendering process, and why it’s important to understand when working with concurrent features.
What Is Legacy Promise Throwing Behavior?
Legacy Promise Throwing Behavior refers to the mechanism where React components "throw" a promise during rendering. This tells React that the component is waiting for some asynchronous data to be resolved before it can be fully rendered.
When a promise is thrown, React pauses rendering of that part of the component tree and instead renders a fallback UI, if defined, using Suspense. Once the promise resolves, React re-renders the component with the resolved data.
Key Features of Legacy Promise Throwing:
- Throwing Promises During Rendering: Components can indicate they’re awaiting data by throwing a promise.
- Integration with Suspense: This behavior works seamlessly with Suspense to show fallback content.
- Asynchronous Data Handling: It provides a declarative way to handle async data fetching within React’s rendering lifecycle.
How Does It Work?
Let’s take a step-by-step look at how this behavior functions:
- Component Throws a Promise: During rendering, a component encounters a promise and throws it.
- Rendering Paused: React halts rendering for that branch of the component tree.
- Fallback UI: If a Suspense boundary is present, React renders the fallback UI.
- Promise Resolves: Once the promise resolves, React resumes rendering and integrates the resolved data.
Example of Legacy Promise Throwing
import React, { Suspense } from 'react'; // Simulated fetch function function fetchData() { return new Promise((resolve) => { setTimeout(() => resolve("Data loaded!"), 2000); }); } // Component that throws a promise function AsyncComponent() { const data = useData(); // Custom hook return <div>{data}</div>; } function useData() { const promise = fetchData(); if (!promise._result) { throw promise; } return promise._result; } function App() { return ( <suspense fallback="{<div">Loading...}> <asynccomponent></asynccomponent> </suspense> ); } export default App;
Explanation:
- Fetching Data: The useData hook fetches data asynchronously using the fetchData function.
- Throwing a Promise: If the data is not yet available, the promise is thrown.
- Suspense Fallback: The Suspense component displays "Loading..." until the promise resolves.
- Resolved Data: Once the promise resolves, the AsyncComponent renders the loaded data.
Modern React and Concurrent Features
React 18 introduced concurrent rendering, which refines how promise throwing works. Key improvements include:
- Time-Slicing: React’s concurrent rendering splits work into smaller chunks, keeping the UI responsive even while waiting for async data.
- Controlled Suspense Boundaries: Suspense boundaries handle promise throwing more gracefully.
- Improved Error Handling: Better error boundaries for components that fail to fetch data.
Best Practices
While Legacy Promise Throwing is foundational to Suspense, developers should use modern libraries and patterns for a better experience:
Use React Query or SWR
Libraries like React Query and SWR provide robust solutions for data fetching, caching, and synchronization, eliminating the need to manually throw promises.
Leverage use Hook (React 18 )
React 18 introduced the use hook (experimental) for handling promises in a cleaner, declarative way.
import React, { Suspense } from 'react'; // Simulated fetch function function fetchData() { return new Promise((resolve) => { setTimeout(() => resolve("Data loaded!"), 2000); }); } // Component that throws a promise function AsyncComponent() { const data = useData(); // Custom hook return <div>{data}</div>; } function useData() { const promise = fetchData(); if (!promise._result) { throw promise; } return promise._result; } function App() { return ( <suspense fallback="{<div">Loading...}> <asynccomponent></asynccomponent> </suspense> ); } export default App;
Avoid Throwing Promises Directly
Throwing promises manually can lead to unexpected issues, especially in non-concurrent environments. Instead, rely on libraries or utilities that abstract these complexities.
Wrap Suspense for Reusability
Encapsulate Suspense logic in reusable components for cleaner code:
function AsyncComponent() { const data = use(fetchData()); return <div>{data}</div>; }
Conclusion
Legacy Promise Throwing Behavior is a cornerstone of React’s Suspense feature, enabling seamless handling of asynchronous data during rendering. However, as React evolves, so do the tools and patterns for managing async operations. By understanding this behavior and leveraging modern practices, developers can create efficient, responsive, and maintainable applications.
The above is the detailed content of Understanding Legacy Promise Throwing Behavior in React. For more information, please follow other related articles on the PHP Chinese website!
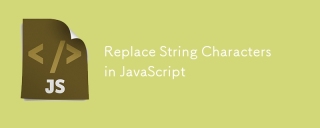
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
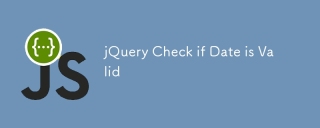
Simple JavaScript functions are used to check if a date is valid. function isValidDate(s) { var bits = s.split('/'); var d = new Date(bits[2] '/' bits[1] '/' bits[0]); return !!(d && (d.getMonth() 1) == bits[1] && d.getDate() == Number(bits[0])); } //test var
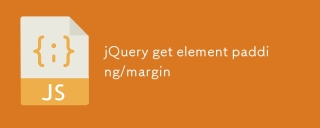
This article discusses how to use jQuery to obtain and set the inner margin and margin values of DOM elements, especially the specific locations of the outer margin and inner margins of the element. While it is possible to set the inner and outer margins of an element using CSS, getting accurate values can be tricky. // set up $("div.header").css("margin","10px"); $("div.header").css("padding","10px"); You might think this code is
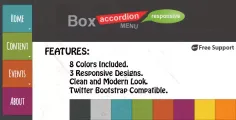
This article explores ten exceptional jQuery tabs and accordions. The key difference between tabs and accordions lies in how their content panels are displayed and hidden. Let's delve into these ten examples. Related articles: 10 jQuery Tab Plugins
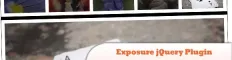
Discover ten exceptional jQuery plugins to elevate your website's dynamism and visual appeal! This curated collection offers diverse functionalities, from image animation to interactive galleries. Let's explore these powerful tools: Related Posts: 1
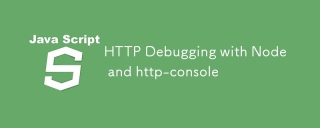
http-console is a Node module that gives you a command-line interface for executing HTTP commands. It’s great for debugging and seeing exactly what is going on with your HTTP requests, regardless of whether they’re made against a web server, web serv
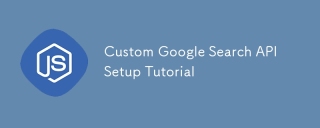
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
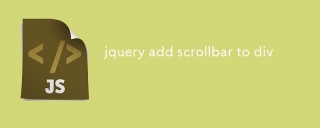
The following jQuery code snippet can be used to add scrollbars when the div content exceeds the container element area. (No demonstration, please copy it directly to Firebug) //D = document //W = window //$ = jQuery var contentArea = $(this), wintop = contentArea.scrollTop(), docheight = $(D).height(), winheight = $(W).height(), divheight = $('#c


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
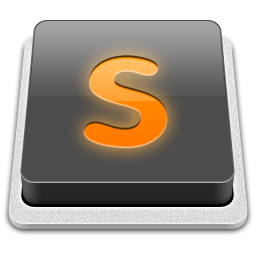
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
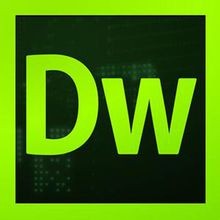
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
