


How to Build a Generic CRUD Controller in Laravel for Multiple Resources
Managing multiple CRUD operations in a Laravel application can be overwhelming, especially when handling a growing number of models. In this post, I'll guide you on creating a generic CRUD controller that allows you to handle existing and future CRUD operations in a single controller.
Why Use a Generic Controller?
A generic controller helps:
- Minimize repetitive code.
- Make adding new models a breeze.
- Provide a consistent structure for your application.
Let’s dive into the implementation step by step!
Step 1: Setting Up the Controller
Start by creating a new controller:
php artisan make:controller GenericController
Step 2: Writing the Controller Logic
Here’s how you can design your GenericController to handle CRUD operations for any model:
namespace App\Http\Controllers; use Illuminate\Http\Request; use Illuminate\Support\Str; class GenericController extends Controller { protected function getModel($modelName) { $modelClass = 'App\Models\' . Str::studly($modelName); if (!class_exists($modelClass)) { abort(404, "Model $modelName not found."); } return new $modelClass; } public function index($model) { $modelInstance = $this->getModel($model); return response()->json($modelInstance::all()); } public function show($model, $id) { $modelInstance = $this->getModel($model); return response()->json($modelInstance::findOrFail($id)); } public function store(Request $request, $model) { $modelInstance = $this->getModel($model); $data = $request->validate($modelInstance->getFillable()); $created = $modelInstance::create($data); return response()->json($created, 201); } public function update(Request $request, $model, $id) { $modelInstance = $this->getModel($model); $item = $modelInstance::findOrFail($id); $data = $request->validate($modelInstance->getFillable()); $item->update($data); return response()->json($item); } public function destroy($model, $id) { $modelInstance = $this->getModel($model); $item = $modelInstance::findOrFail($id); $item->delete(); return response()->json(['message' => 'Deleted successfully.']); } }
Step 3: Dynamic Routing
Configure your routes to use dynamic endpoints:
use App\Http\Controllers\GenericController; Route::controller(GenericController::class)->prefix('api/{model}')->group(function () { Route::get('/', 'index'); Route::get('/{id}', 'show'); Route::post('/', 'store'); Route::put('/{id}', 'update'); Route::delete('/{id}', 'destroy'); });
Step 4: Prepare Your Models
Ensure each model has:
- A $fillable property to specify mass-assignable fields.
Example for a Post model:
namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Post extends Model { use HasFactory; protected $fillable = ['title', 'content']; }
Key Advantages
- Scalability: Easily handle new models by adding only the model file.
- Code Reusability: Reduces redundancy.
- Simplifies Maintenance: Focus on business logic without worrying about boilerplate code.
When to Use This Approach?
This is ideal for:
- Applications with standard CRUD logic.
- Projects where models share common behavior.
For more complex business logic, you may still need dedicated controllers.
The above is the detailed content of How to Build a Generic CRUD Controller in Laravel for Multiple Resources. For more information, please follow other related articles on the PHP Chinese website!
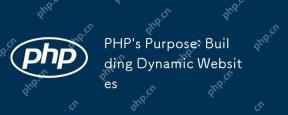
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
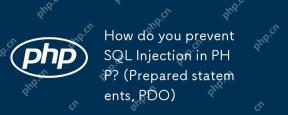
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.
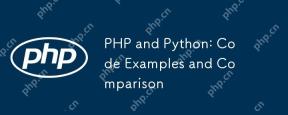
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
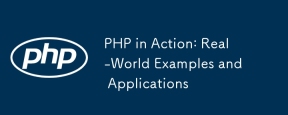
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
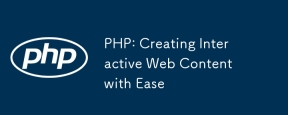
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
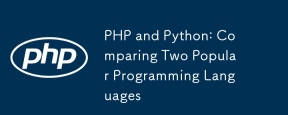
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
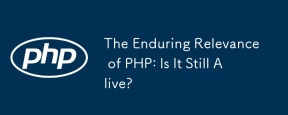
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
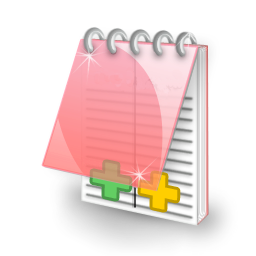
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
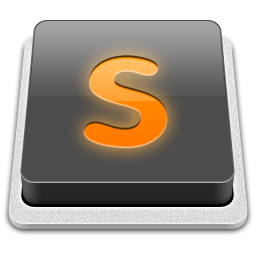
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.