Introduction
In Go programming, creating custom types with validation is paramount for ensuring data integrity and security. This article explores a code structure that exemplifies the creation of a custom type, incorporating robust validation and adhering to best practices for safety and compliance.
Code Structure
Let's break down the essential components:
- Necessary Imports:
import ( "fmt" "strings" )
- Custom Type Definition:
type Example string
We define a custom type Example as a string, providing a clear and concise representation of the data.
- Constants and Allowed Options:
const ( ArgumentA = "value_a" ArgumentB = "value_b" ) var AllowedOptions = []string{string(ArgumentA), string(ArgumentB)}
We define constants for allowed values and store them in a slice for easy reference and management.
- Methods for the Example Type:
- String(): Returns the string representation of the Example value.
func (f Example) String() string { return string(f) }
- Type(): Returns the name of the type.
func (f *Example) Type() string { return "Example" }
- Set(): Validates the input value and sets the Example value if valid.
func (f *Example) Set(value string) error { for _, exampleOption := range AllowedOptions { if exampleOption == value { *f = Example(value) return nil } } return fmt.Errorf("allowed values: %s", strings.Join(AllowedOptions, ", ")) }
Advantages of Using Custom Types with Validation
- Enhanced Data Security: By rigorously validating input, we prevent invalid or malicious data from entering the system, bolstering overall security.
- Improved Compliance: Adhering to validation rules helps ensure compliance with relevant standards like GDPR or HIPAA.
- Increased Code Maintainability: Custom types promote modularity and make code easier to maintain and extend.
- Enhanced Type Safety: Go's type system provides compile-time checks, minimizing runtime errors and improving code quality.
- Improved Code Readability: Custom types make code more self-documenting, enhancing understanding and collaboration.
Conclusion
Employing custom types with validation in Go is a best practice for developing robust, secure, and maintainable applications. This approach is particularly valuable in scenarios demanding high data integrity, such as financial systems or healthcare applications.
Additional Considerations
- Thorough Testing: Rigorous testing of custom types, especially the Set method, is crucial to ensure validation works as expected.
- Meaningful Error Handling: Provide informative error messages to aid in debugging and troubleshooting.
- Contextual Adaptation: Tailor validation logic to specific use cases, such as command-line arguments or configuration file parsing.
By embracing custom types with validation, you can significantly enhance the quality, security, and reliability of your Go applications.
Complete Code Example:
import ( "fmt" "strings" )
- Official Go Documentation
- Effective Go
- Go Data Structures
- Package flag in Go
The above is the detailed content of Creating Safe Custom Types with Validation in Go. For more information, please follow other related articles on the PHP Chinese website!
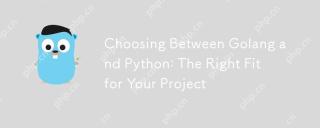
Golangisidealforperformance-criticalapplicationsandconcurrentprogramming,whilePythonexcelsindatascience,rapidprototyping,andversatility.1)Forhigh-performanceneeds,chooseGolangduetoitsefficiencyandconcurrencyfeatures.2)Fordata-drivenprojects,Pythonisp
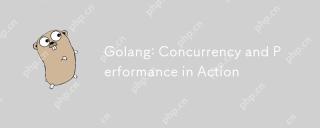
Golang achieves efficient concurrency through goroutine and channel: 1.goroutine is a lightweight thread, started with the go keyword; 2.channel is used for secure communication between goroutines to avoid race conditions; 3. The usage example shows basic and advanced usage; 4. Common errors include deadlocks and data competition, which can be detected by gorun-race; 5. Performance optimization suggests reducing the use of channel, reasonably setting the number of goroutines, and using sync.Pool to manage memory.
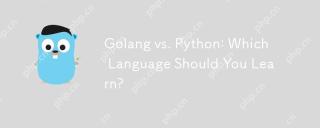
Golang is more suitable for system programming and high concurrency applications, while Python is more suitable for data science and rapid development. 1) Golang is developed by Google, statically typing, emphasizing simplicity and efficiency, and is suitable for high concurrency scenarios. 2) Python is created by Guidovan Rossum, dynamically typed, concise syntax, wide application, suitable for beginners and data processing.
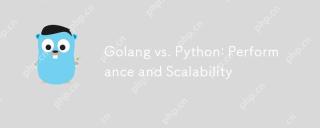
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
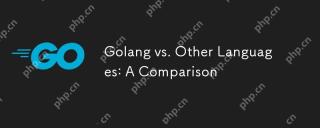
Go language has unique advantages in concurrent programming, performance, learning curve, etc.: 1. Concurrent programming is realized through goroutine and channel, which is lightweight and efficient. 2. The compilation speed is fast and the operation performance is close to that of C language. 3. The grammar is concise, the learning curve is smooth, and the ecosystem is rich.
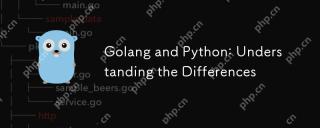
The main differences between Golang and Python are concurrency models, type systems, performance and execution speed. 1. Golang uses the CSP model, which is suitable for high concurrent tasks; Python relies on multi-threading and GIL, which is suitable for I/O-intensive tasks. 2. Golang is a static type, and Python is a dynamic type. 3. Golang compiled language execution speed is fast, and Python interpreted language development is fast.
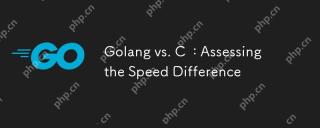
Golang is usually slower than C, but Golang has more advantages in concurrent programming and development efficiency: 1) Golang's garbage collection and concurrency model makes it perform well in high concurrency scenarios; 2) C obtains higher performance through manual memory management and hardware optimization, but has higher development complexity.
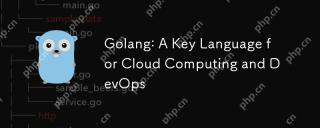
Golang is widely used in cloud computing and DevOps, and its advantages lie in simplicity, efficiency and concurrent programming capabilities. 1) In cloud computing, Golang efficiently handles concurrent requests through goroutine and channel mechanisms. 2) In DevOps, Golang's fast compilation and cross-platform features make it the first choice for automation tools.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
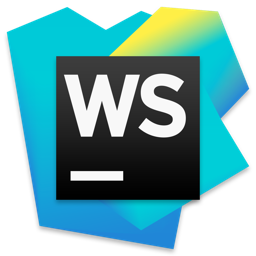
WebStorm Mac version
Useful JavaScript development tools
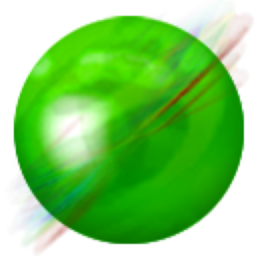
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Notepad++7.3.1
Easy-to-use and free code editor