Calling SignalR Core Hub Methods from Asp.Net Core Controller
In this article, we'll explore how to invoke SignalR Core Hub methods from within an ASP.NET Core controller. This functionality allows for server-side communication to connected clients to broadcast events or update real-time data.
Problem Overview
In the provided scenario, an ASP.NET Core application receives a request from a Windows service upon task completion. The task is to notify all connected SignalR Core clients about this event. However, the Windows service cannot directly establish a connection to SignalR.
Solution 1: Injecting HubContext into Controller
One approach is to inject the appropriate HubContext into the controller class. This enables direct invocation of hub methods from the controller's action methods.
[Route("API/vardesigncomm")] public class VarDesignCommController : Controller { [HttpPut("ProcessVarDesignCommResponse/{id}")] public async Task<iactionresult> ProcessVarDesignCommResponse(int id) { await this.HubContext.Clients.All.InvokeAsync("Completed", id); return new JsonResult(true); } private IHubContext<vardesignhub> HubContext { get; set; } }</vardesignhub></iactionresult>
This approach allows direct communication with all connected clients, but may have performance implications depending on the number of clients.
Solution 2: Typed Hubs and Client Interfaces
Another approach involves defining a typed client interface and inheriting from the corresponding Hub with the typed client interface. This enables the injection of the typed HubContext into the controller for specific method invocations.
Typed Client Interface:
public interface ITypedHubClient { Task BroadcastMessage(string name, string message); }
Hub Inheriting from Typed Client Interface:
public class ChatHub : Hub<itypedhubclient> { public void Send(string name, string message) { Clients.All.BroadcastMessage(name, message); } }</itypedhubclient>
Controller Injection and Method Invocation:
[Route("api/demo")] public class DemoController : Controller { IHubContext<chathub itypedhubclient> _chatHubContext; public DemoController(IHubContext<chathub itypedhubclient> chatHubContext) { _chatHubContext = chatHubContext; } [HttpGet] public IEnumerable<string> Get() { _chatHubContext.Clients.All.BroadcastMessage("test", "test"); return new string[] { "value1", "value2" }; } }</string></chathub></chathub>
This approach allows for more targeted and efficient communication with clients.
The above is the detailed content of How to Call SignalR Core Hub Methods from an ASP.NET Core Controller?. For more information, please follow other related articles on the PHP Chinese website!
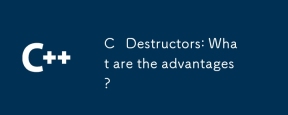
C destructorsprovideseveralkeyadvantages:1)Theymanageresourcesautomatically,preventingleaks;2)Theyenhanceexceptionsafetybyensuringresourcerelease;3)TheyenableRAIIforsaferesourcehandling;4)Virtualdestructorssupportpolymorphiccleanup;5)Theyimprovecode
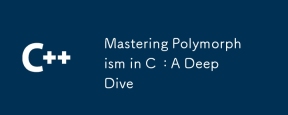
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
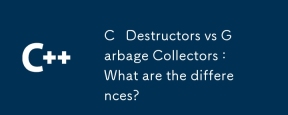
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
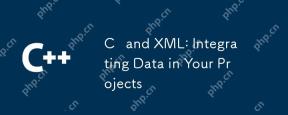
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
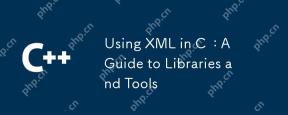
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
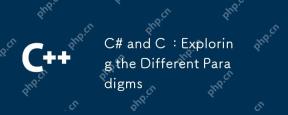
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
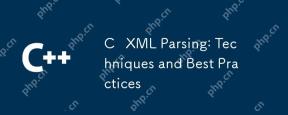
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
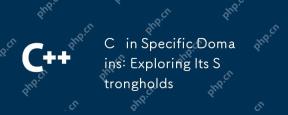
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
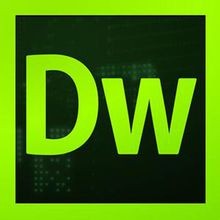
Dreamweaver CS6
Visual web development tools
