


Async/Await: Should I Wrap My Method in `Task.Run` for Background Threading?
Unraveling Async/Await: Method Wrapper or Background Thread?
In the pursuit of understanding async/await, the dilemma arises: Is it necessary to encapsulate a method within Task.Run to achieve both async behavior and background thread execution?
Async Methods vs. Awaitable Tasks
"Async" denotes a method that can yield control to the calling thread before commencing execution. This yielding occurs through await expressions. In contrast, "asynchronous" as defined by MSDN (an often misleading term) refers to code that runs on a separate thread.
Separately, "awaitable" describes a type that can be used with the await operator. Common awaitables include Task and Task
Tailoring Code for Background Thread Execution
To execute a method on a background thread while maintaining awaitability, utilize Task.Run:
private Task<int> DoWorkAsync() { return Task.Run(() => 1 + 2); }</int>
However, this approach is generally discouraged.
Enabling Asynchronous Yielding
To create an async method that can pause and yield control, declare the method as async and employ await at designated yielding points:
private async Task<int> GetWebPageHtmlSizeAsync() { var html = await client.GetAsync("http://www.example.com/"); return html.Length; }</int>
Linking Asynchronous Code and Awaitables
Async code relies on awaitables in its await expressions. Awaitables can be either other async methods or synchronous methods that return awaitables.
Wrapping Methods in Task.Run: A Discouraged Practice
Avoid indiscriminately wrapping synchronous methods within Task.Run. Instead, maintain synchronous signatures, leaving the option of wrapping to the consumer.
Additional Resources for Async/Await
- [Async/Await Fundamentals](https://blog.stephencleary.com/2012/02/async-await-fundamentals.html)
- [MSDN Async Documentation](https://docs.microsoft.com/en-us/dotnet/csharp/programming-guide/concepts/async/)
The above is the detailed content of Async/Await: Should I Wrap My Method in `Task.Run` for Background Threading?. For more information, please follow other related articles on the PHP Chinese website!
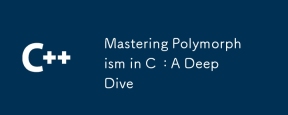
Mastering polymorphisms in C can significantly improve code flexibility and maintainability. 1) Polymorphism allows different types of objects to be treated as objects of the same base type. 2) Implement runtime polymorphism through inheritance and virtual functions. 3) Polymorphism supports code extension without modifying existing classes. 4) Using CRTP to implement compile-time polymorphism can improve performance. 5) Smart pointers help resource management. 6) The base class should have a virtual destructor. 7) Performance optimization requires code analysis first.
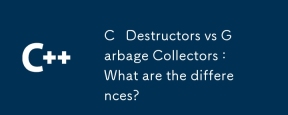
C destructorsprovideprecisecontroloverresourcemanagement,whilegarbagecollectorsautomatememorymanagementbutintroduceunpredictability.C destructors:1)Allowcustomcleanupactionswhenobjectsaredestroyed,2)Releaseresourcesimmediatelywhenobjectsgooutofscop
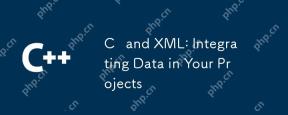
Integrating XML in a C project can be achieved through the following steps: 1) parse and generate XML files using pugixml or TinyXML library, 2) select DOM or SAX methods for parsing, 3) handle nested nodes and multi-level properties, 4) optimize performance using debugging techniques and best practices.
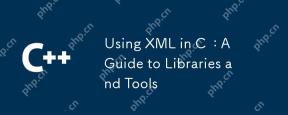
XML is used in C because it provides a convenient way to structure data, especially in configuration files, data storage and network communications. 1) Select the appropriate library, such as TinyXML, pugixml, RapidXML, and decide according to project needs. 2) Understand two ways of XML parsing and generation: DOM is suitable for frequent access and modification, and SAX is suitable for large files or streaming data. 3) When optimizing performance, TinyXML is suitable for small files, pugixml performs well in memory and speed, and RapidXML is excellent in processing large files.
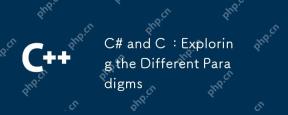
The main differences between C# and C are memory management, polymorphism implementation and performance optimization. 1) C# uses a garbage collector to automatically manage memory, while C needs to be managed manually. 2) C# realizes polymorphism through interfaces and virtual methods, and C uses virtual functions and pure virtual functions. 3) The performance optimization of C# depends on structure and parallel programming, while C is implemented through inline functions and multithreading.
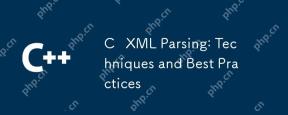
The DOM and SAX methods can be used to parse XML data in C. 1) DOM parsing loads XML into memory, suitable for small files, but may take up a lot of memory. 2) SAX parsing is event-driven and is suitable for large files, but cannot be accessed randomly. Choosing the right method and optimizing the code can improve efficiency.
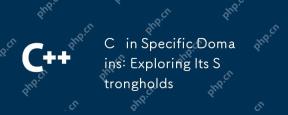
C is widely used in the fields of game development, embedded systems, financial transactions and scientific computing, due to its high performance and flexibility. 1) In game development, C is used for efficient graphics rendering and real-time computing. 2) In embedded systems, C's memory management and hardware control capabilities make it the first choice. 3) In the field of financial transactions, C's high performance meets the needs of real-time computing. 4) In scientific computing, C's efficient algorithm implementation and data processing capabilities are fully reflected.
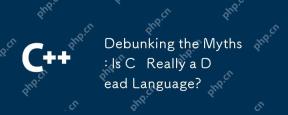
C is not dead, but has flourished in many key areas: 1) game development, 2) system programming, 3) high-performance computing, 4) browsers and network applications, C is still the mainstream choice, showing its strong vitality and application scenarios.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
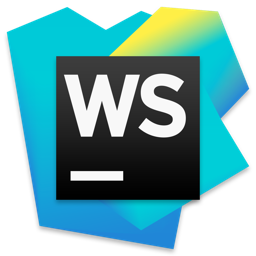
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!
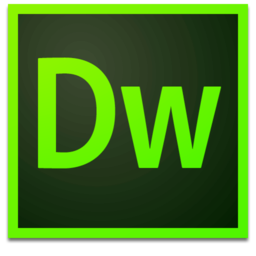
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
